I. Introduction
“Text is everywhere—on street signs, product labels, scanned documents, you name it. But making a machine accurately detect and extract it? That’s a whole different challenge.”
Over the years, I’ve worked with multiple text detection methods, from traditional computer vision techniques to deep learning-based approaches.
Some work like magic on clean documents, while others struggle with real-world noise—blurred images, uneven lighting, or rotated text. If you’ve ever tried to extract text from messy data, you know exactly what I’m talking about.
Here’s the deal: OpenCV, combined with some smart preprocessing, can get you surprisingly good results—without needing a heavy deep-learning model.
In this guide, I’ll walk you through the best methods I’ve used for text detection, focusing on practical implementation with minimal theory and maximum code.
By the end, you’ll know exactly:
✔️ How to preprocess images for optimal text detection
✔️ The best OpenCV techniques for different scenarios (EAST, MSER, contours)
✔️ How to extract text using Tesseract
✔️ Advanced tricks to improve accuracy and reduce false positives
Let’s get started.
II. Setting Up the Environment
1. Installing the Necessary Libraries
Before we jump into code, let’s make sure your setup is ready. Here’s what you’ll need:
- OpenCV – for image processing and text detection
- NumPy – for handling arrays efficiently
- Tesseract-OCR – for text extraction (optional but highly recommended)
I personally prefer installing everything using pip
because it’s fast and avoids compatibility issues. Run this command:
pip install opencv-python numpy pytesseract
On Linux/macOS, you’ll also need to install Tesseract separately:
sudo apt-get install tesseract-ocr # Ubuntu/Debian
brew install tesseract # macOS
For Windows, download and install it from Tesseract’s official site. Once installed, make sure to add it to your system’s PATH.
2. Verifying the Installation
Trust me, you don’t want to start coding only to realize something is missing. A quick way to check if everything is installed properly:
import cv2
import numpy as np
import pytesseract
print("OpenCV version:", cv2.__version__)
print("NumPy version:", np.__version__)
print("Tesseract version:", pytesseract.get_tesseract_version())
If this runs without errors, you’re good to go.
Up next, we’ll dive into image preprocessing—because raw images aren’t always text-friendly, and that’s where the real magic starts.
III. Loading and Preprocessing Images
“Garbage in, garbage out.”
That’s a saying I learned the hard way when working with text detection. If your input image is noisy, blurry, or poorly lit, even the best algorithms will struggle. Over time, I’ve figured out a few must-do preprocessing steps that can dramatically improve detection accuracy.
Let’s break them down.
1. Image Resizing – Finding the Sweet Spot
You might be tempted to throw high-resolution images into your model, but trust me—bigger isn’t always better. Large images slow down processing, and tiny text regions might get lost when resizing.
Personally, I’ve found that keeping the width between 600-1200 pixels works well for most cases.
import cv2
def resize_image(image, width=1000):
aspect_ratio = width / float(image.shape[1])
height = int(image.shape[0] * aspect_ratio)
return cv2.resize(image, (width, height))
# Load and resize
image = cv2.imread('sample_image.jpg')
image = resize_image(image, width=800)
cv2.imshow('Resized Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
This ensures a balanced trade-off between speed and accuracy.
2. Noise Reduction – Because Real-World Data is Messy
One thing I’ve learned from working with OCR is that clean input = better results. If your image is full of noise (like scanned documents or outdoor signs), you’ll need to smooth it out before detecting text.
I usually rely on two methods:
🔹 Gaussian Blur – Works well for light noise
🔹 Bilateral Filter – Preserves edges while reducing noise (better for text-heavy images)
# Convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian Blur
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# Apply Bilateral Filter (alternative for noisy images)
bilateral = cv2.bilateralFilter(gray, 9, 75, 75)
When dealing with scanned documents, Bilateral Filtering has often saved me from losing fine text details.
3. Grayscale Conversion – Making Text Stand Out
I always convert images to grayscale before further processing. Why? Because color information is useless for text detection—what really matters is contrast.
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow('Grayscale Image', gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
Simple, but crucial.
4. Adaptive Thresholding – Enhancing Text for Detection
This is where things get interesting. If your text has varying lighting conditions, regular thresholding won’t cut it. Instead, I use adaptive thresholding to dynamically adjust the threshold based on local pixel intensity.
thresh = cv2.adaptiveThreshold(gray, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C,
cv2.THRESH_BINARY, 11, 2)
cv2.imshow('Thresholded Image', thresh)
cv2.waitKey(0)
cv2.destroyAllWindows()
This method has saved me countless times when dealing with uneven lighting in images. The text pops out beautifully, making detection easier.
Final Thoughts
If you take away one thing from this section, it’s this: Preprocessing is not optional. It can make or break your text detection pipeline.
I’ve personally fine-tuned these steps over multiple projects, and trust me, getting this right upfront saves hours of debugging later.
In the next section, we’ll dive into text detection methods—where the real action begins.
IV. Text Detection Techniques in OpenCV
“If all you have is a hammer, everything looks like a nail.”
That’s exactly how I felt when I first started working with text detection. I used one method for everything—until I realized that different approaches work better depending on the type of text, background, and image conditions.
So, instead of forcing a single solution, I’ve learned to pick the right tool for the job. Let’s go through three powerful OpenCV-based techniques:
1. Contour-Based Detection – Best for Clean, Structured Text
If your text is well-separated from the background—like on a white document or a clear sign—contour-based detection can work wonders. It’s fast, lightweight, and doesn’t require deep learning.
Here’s how I typically use it:
✅ Find edges using Canny Edge Detection
✅ Extract contours and filter them based on size/shape
✅ Draw bounding boxes around detected text
Code: Contour-Based Text Detection
import cv2
import numpy as np
# Load image
image = cv2.imread('sample_image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Edge detection
edges = cv2.Canny(gray, 50, 150)
# Find contours
contours, _ = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Filter contours based on area and aspect ratio
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
if w > 30 and h > 10: # Adjust thresholds based on your use case
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.imshow('Detected Text', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
🔹 When to use this? When working with structured text layouts, like invoices, receipts, or digital documents.
🔹 Limitations? Struggles with handwritten text or highly cluttered images.
2. EAST – Deep Learning-Based Scene Text Detection
Now, what if you’re dealing with real-world images—street signs, product packaging, or text in dynamic environments?
This is where EAST (Efficient and Accurate Scene Text Detector) shines. Unlike traditional methods, EAST is a deep learning-based model that can detect text in any orientation, without needing multiple preprocessing steps.
Code: EAST-Based Text Detection
import cv2
import numpy as np
# Load the pre-trained EAST model
net = cv2.dnn.readNet("frozen_east_text_detection.pb")
# Load and preprocess image
image = cv2.imread('sample_image.jpg')
orig = image.copy()
(H, W) = image.shape[:2]
# Resize to multiple of 32 (requirement for EAST)
newW, newH = (320, 320)
rW, rH = W / float(newW), H / float(newH)
image = cv2.resize(image, (newW, newH))
# Prepare image as input blob
blob = cv2.dnn.blobFromImage(image, 1.0, (newW, newH),
(123.68, 116.78, 103.94), swapRB=True, crop=False)
net.setInput(blob)
# Get scores and geometry from the model
scores, geometry = net.forward(['feature_fusion/Conv_7/Sigmoid',
'feature_fusion/concat_3'])
# Post-processing to extract bounding boxes
def decode_predictions(scores, geometry, conf_threshold=0.5):
rects, confidences = [], []
for y in range(scores.shape[2]):
for x in range(scores.shape[3]):
if scores[0, 0, y, x] < conf_threshold:
continue
offsetX, offsetY = x * 4.0, y * 4.0
angle = geometry[0, 4, y, x]
cosA, sinA = np.cos(angle), np.sin(angle)
h, w = geometry[0, 0, y, x], geometry[0, 1, y, x]
endX = int(offsetX + (cosA * w) + (sinA * h))
endY = int(offsetY - (sinA * w) + (cosA * h))
startX, startY = int(endX - w), int(endY - h)
rects.append((startX, startY, endX, endY))
confidences.append(scores[0, 0, y, x])
return rects, confidences
# Apply Non-Maximum Suppression to filter overlapping boxes
from imutils.object_detection import non_max_suppression
rects, confidences = decode_predictions(scores, geometry)
boxes = non_max_suppression(np.array(rects), probs=confidences)
# Draw detected text regions
for (startX, startY, endX, endY) in boxes:
cv2.rectangle(orig, (startX, startY), (endX, endY), (0, 255, 0), 2)
cv2.imshow("EAST Text Detection", orig)
cv2.waitKey(0)
cv2.destroyAllWindows()
🔹 Why use EAST? Because it’s robust against perspective distortions, tilted text, and low-contrast images.
🔹 Where does it struggle? It’s slower than contour-based methods and requires a pre-trained model.
3. MSER – Detecting Text in Tough Conditions
Sometimes, I deal with unstructured text layouts, like graffiti, cluttered backgrounds, or text embedded in artistic designs. In such cases, MSER (Maximally Stable Extremal Regions) has been surprisingly effective.
MSER works by detecting regions with stable intensity, making it great for text detection in varying lighting conditions.
Code: MSER-Based Text Detection
import cv2
import numpy as np
# Load image
image = cv2.imread('sample_image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply MSER
mser = cv2.MSER_create()
regions, _ = mser.detectRegions(gray)
# Draw detected regions
for p in regions:
hull = cv2.convexHull(p.reshape(-1, 1, 2))
cv2.polylines(image, [hull], True, (0, 255, 0), 2)
cv2.imshow("MSER Text Detection", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
🔹 Best for? Complex backgrounds, irregular fonts, and non-standard text placement.
🔹 Downside? Can detect too many false positives, so post-processing is needed.
Final Thoughts
No single method works for every situation. Here’s my personal rule of thumb:
✔️ Use Contours if the text is well-structured and separated from the background.
✔️ Use EAST if you need to detect text in real-world, unstructured environments.
✔️ Use MSER when dealing with cluttered backgrounds and tough lighting conditions.
From my experience, combining preprocessing techniques with the right detection method gives the best accuracy. And that’s exactly what we’ll dive into next—extracting text from these detections using OCR.
V. Post-Processing and Refinement
“Getting good detections is one thing—getting usable detections is another.”
When I first started working with text detection, I noticed something frustrating: bounding boxes everywhere—some overlapping, some redundant, and some just plain wrong. That’s when I realized post-processing is just as critical as detection itself.
So, let’s refine our detections with two key techniques:
1️⃣ Non-Maximum Suppression (NMS) – To filter out redundant detections
2️⃣ Morphological Transformations – To clean up text contours
1. Non-Maximum Suppression (NMS) – Eliminating Duplicate Detections
EAST, for example, outputs multiple bounding boxes for the same text. That’s why NMS is crucial—it removes overlapping boxes while keeping the most confident detection.
Code: Applying Non-Maximum Suppression
import numpy as np
def non_max_suppression(boxes, scores, overlapThresh=0.5):
if len(boxes) == 0:
return []
boxes = np.array(boxes)
scores = np.array(scores)
x1, y1, x2, y2 = boxes[:, 0], boxes[:, 1], boxes[:, 2], boxes[:, 3]
areas = (x2 - x1 + 1) * (y2 - y1 + 1)
idxs = scores.argsort()[::-1] # Sort boxes by confidence score
picked_boxes = []
while len(idxs) > 0:
i = idxs[0]
picked_boxes.append(boxes[i])
xx1 = np.maximum(x1[i], x1[idxs[1:]])
yy1 = np.maximum(y1[i], y1[idxs[1:]])
xx2 = np.minimum(x2[i], x2[idxs[1:]])
yy2 = np.minimum(y2[i], y2[idxs[1:]])
w = np.maximum(0, xx2 - xx1 + 1)
h = np.maximum(0, yy2 - yy1 + 1)
overlap = (w * h) / areas[idxs[1:]]
idxs = idxs[np.where(overlap <= overlapThresh)[0] + 1]
return picked_boxes
🔹 What this does:
✔ Filters out redundant detections
✔ Keeps the most relevant bounding boxes
✔ Helps when multiple overlapping detections appear
2. Morphological Transformations – Refining Text Contours
Ever had text fragmented into pieces during detection? That’s where morphological operations come in handy. Dilating, eroding, and closing gaps help connect broken parts of detected text.
Code: Using Morphological Transformations for Cleanup
import cv2
import numpy as np
# Load thresholded image from previous step
thresh = cv2.imread("thresh_image.jpg", 0)
# Define kernel for morphological operations
kernel = np.ones((3, 3), np.uint8)
# Apply closing to connect broken parts of text
morph = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, kernel, iterations=2)
cv2.imshow("Refined Text", morph)
cv2.waitKey(0)
cv2.destroyAllWindows()
🔹 Why this works?
✔ Connects broken letters
✔ Removes small noise
✔ Helps OCR perform better
Now that we’ve cleaned up our detections, let’s move on to extracting actual text from these images.
VI. Extracting Text Using Tesseract
“Detecting text is great, but what’s the point if we can’t read it?”
That’s exactly where Tesseract OCR comes in. But here’s the deal: Tesseract is picky. If your text isn’t well-prepared, it’ll give you garbage output.
So, before running Tesseract, I always follow these rules:
✅ Ensure proper DPI (300+ is ideal for best accuracy)
✅ Use binary thresholding (like Otsu’s or Adaptive Thresholding)
✅ Resize text to an optimal size (not too small, not too large)
1. Preprocessing for Tesseract OCR
The better your input, the better your OCR results. Let’s prepare the image properly before extracting text.
Code: Optimizing Image for OCR
import cv2
import pytesseract
# Load and preprocess image
image = cv2.imread('sample_image.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)[1]
# Resize for better OCR accuracy
thresh = cv2.resize(thresh, None, fx=1.5, fy=1.5, interpolation=cv2.INTER_CUBIC)
cv2.imshow("Preprocessed Image", thresh)
cv2.waitKey(0)
cv2.destroyAllWindows()
🔹 Why this works?
✔ Enhances text visibility
✔ Removes unwanted noise
✔ Resizes text to a readable size
2. Extracting Text Using Tesseract
Once we have a clean, well-prepared image, extracting text is simple.
Code: Running OCR with Tesseract
import pytesseract
# Ensure Tesseract is installed and its path is configured (if needed)
pytesseract.pytesseract.tesseract_cmd = r'C:\Program Files\Tesseract-OCR\tesseract.exe' # Change path if necessary
# Perform OCR
text = pytesseract.image_to_string(thresh, lang='eng')
print("Detected Text:\n", text)
🔹 Best Practices:
✔ Use "lang='eng'"
to specify language
✔ Fine-tune Tesseract parameters if needed (e.g., --psm 6
for dense text)
Final Thoughts
From my experience, text detection is only half the battle—cleaning up results and extracting accurate text is what makes a real difference.
Here’s a quick summary of what I always do:
✔ Apply Non-Maximum Suppression to remove redundant detections
✔ Use Morphological Operations to refine detected text regions
✔ Preprocess images for OCR (resize, threshold, remove noise)
✔ Extract text using Tesseract with fine-tuned parameters
By using these post-processing tricks, I’ve been able to boost OCR accuracy significantly—especially when dealing with real-world images, blurry receipts, or distorted text.
Next, we’ll take it a step further: fine-tuning OCR parameters and handling low-quality images.
VII. Performance Optimization and Best Practices
“Speed is everything—until accuracy fails you.”
When I first started working with large-scale text detection, I ran into a brutal reality: my models were accurate but painfully slow. If you’ve ever worked with real-time applications, you know exactly what I’m talking about. High accuracy is useless if your system lags.
So, how do you optimize for speed without compromising accuracy?
Let’s dive into four critical optimizations I use regularly:
1️⃣ Speeding up detection for large-scale data
2️⃣ Reducing false positives
3️⃣ Fine-tuning parameters for optimal performance
4️⃣ Balancing accuracy vs. speed trade-offs
1. Speeding Up Text Detection on Large-Scale Data
If you’re processing thousands of images (or worse, real-time video), efficiency is non-negotiable. Here’s what I’ve found most effective:
✅ Resize Input Images: Models like EAST work on fixed input sizes. Instead of processing high-resolution images, downscale before feeding them in.
✅ Use FP16 Instead of FP32: If you’re on a GPU, switch to 16-bit floating-point precision (FP16). You get the same results at nearly double the speed.
✅ Run Inference on a Smaller ROI (Region of Interest): If your text is localized in a specific area, crop the input instead of processing the entire image.
Code: Faster Inference Using Optimized Blob Size
import cv2
# Load pre-trained EAST model
net = cv2.dnn.readNet("frozen_east_text_detection.pb")
# Resize image for faster processing
H, W = 320, 320 # Optimal size for EAST
blob = cv2.dnn.blobFromImage(image, 1.0, (W, H), (123.68, 116.78, 103.94), swapRB=True, crop=False)
# Run inference
net.setInput(blob)
scores, geometry = net.forward(["feature_fusion/Conv_7/Sigmoid", "feature_fusion/concat_3"])
🔹 Why this works?
✔ Reduces input size → Faster computations
✔ Uses optimized DNN inference → Fewer redundant calculations
2. Reducing False Positives
“You don’t want your model detecting ‘text’ where there is none.”
One of the biggest challenges is false positives—random objects being detected as text. I’ve tackled this by:
✅ Applying Non-Maximum Suppression (NMS) – We covered this earlier; it eliminates redundant bounding boxes.
✅ Filtering by Aspect Ratio & Area – Most real text follows a certain width-to-height ratio. Setting min/max values removes garbage detections.
✅ Using a Confidence Threshold – Many text detectors output a confidence score. Setting a threshold (e.g., >0.7) removes unreliable detections.
Code: Filtering Text Based on Aspect Ratio & Confidence
valid_boxes = []
min_confidence = 0.7
min_aspect_ratio = 2.0 # Text is usually wider than tall
for i in range(len(boxes)):
x, y, w, h = boxes[i]
confidence = scores[i]
aspect_ratio = w / float(h)
if confidence > min_confidence and aspect_ratio > min_aspect_ratio:
valid_boxes.append(boxes[i])
🔹 Why this works?
✔ Removes random false positives
✔ Keeps only text-like regions
✔ Ensures higher OCR accuracy later
3. Fine-Tuning Parameters for Optimal Performance
“There is no perfect parameter setting—only the best one for your use case.”
Here’s what I tweak first when optimizing:
🔹 EAST Model
- Reduce input resolution for speed
- Increase resolution for better small-text detection
🔹 MSER (for scene text)
- Δ (delta parameter) – Higher values reduce noise but may miss fine details
🔹 Tesseract OCR
"--psm 6"
works best for dense text"--oem 1"
uses the latest neural nets for better accuracy
4. Balancing Accuracy vs. Performance Trade-offs
“Faster isn’t always better—but neither is slower.”
When I work on real-world projects, I always ask:
✅ Is the system for real-time applications? → Prioritize speed
✅ Is text detection for static document scans? → Prioritize accuracy
🔹 Example Trade-offs
Scenario | Optimization Focus |
---|---|
Real-time Video OCR | Reduce image size, use GPU, FP16 inference |
Document OCR | High-resolution input, use Tesseract "--psm 6" |
Handwritten Text | Adaptive thresholding, noise removal |
VIII. Evaluation and Metrics
“If you can’t measure it, you can’t improve it.”
When I evaluate text detection models, I focus on three metrics:
1️⃣ Precision – How many of the detected text regions are actually text?
2️⃣ Recall – How much real text did the model successfully detect?
3️⃣ F1 Score – The balance between Precision & Recall
1. Precision, Recall, and F1 Score Explained
Let’s say your model detects 100 text boxes, but only 80 are correct.
And out of the actual 120 text regions, your model found 80.
Precision = Correct Detections / Total DetectionsPrecision = 80 / 100 = 0.8 (80%)
Recall = Correct Detections / Actual Text RegionsRecall = 80 / 120 = 0.67 (67%)
F1 Score = (2 × Precision × Recall) / (Precision + Recall)F1 Score = (2 × 0.8 × 0.67) / (0.8 + 0.67) ≈ 0.73 (73%)
2. Sample Code: Evaluating Text Detection Performance
Let’s compute Precision, Recall, and F1 Score programmatically.
from sklearn.metrics import precision_score, recall_score, f1_score
# Ground truth (1 = text present, 0 = no text)
y_true = [1, 1, 1, 0, 1, 0, 1, 1, 0, 1]
# Model predictions (1 = detected as text, 0 = not detected)
y_pred = [1, 1, 0, 0, 1, 0, 1, 1, 1, 1]
# Compute metrics
precision = precision_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
f1 = f1_score(y_true, y_pred)
print(f"Precision: {precision:.2f}")
print(f"Recall: {recall:.2f}")
print(f"F1 Score: {f1:.2f}")
🔹 Why this matters?
✔ Helps understand if the model is overfitting (high precision, low recall)
✔ Helps improve model performance by tuning detection thresholds
IX. Deployment Considerations
“A model that sits in your Jupyter notebook isn’t solving real problems.”
I’ve seen this happen countless times—someone builds an incredible text detection system, but when it comes to deployment, things fall apart. Performance bottlenecks, API latency issues, and compatibility nightmares—I’ve dealt with them all.
So, let’s go over three critical aspects of deployment:
1️⃣ Exporting the model for real-time applications
2️⃣ Deployment options: Flask, FastAPI, and cloud services
3️⃣ Using Docker for scalable deployment
1. Exporting the Model for Real-Time Use
If you want to use your model outside your training environment, exporting it properly is non-negotiable.
Key things I focus on when exporting:
✅ Convert to ONNX – This optimizes inference speed across different platforms.
✅ Use TensorRT (if on GPU) – NVIDIA’s TensorRT can accelerate inference by 5-10x.
✅ Save the model in a portable format – For OpenCV’s EAST model, .pb
(Protocol Buffer) works best.
Code: Exporting an ONNX Model for Fast Inference
import torch
# Load trained PyTorch model
model = YourTextDetectionModel()
model.load_state_dict(torch.load("text_detector.pth"))
model.eval()
# Export to ONNX
dummy_input = torch.randn(1, 3, 320, 320) # Adjust input shape
torch.onnx.export(model, dummy_input, "text_detector.onnx", opset_version=11)
🔹 Why ONNX?
✔ Works across PyTorch, TensorFlow, and OpenCV
✔ Runs faster than raw PyTorch/TensorFlow models
✔ Compatible with mobile and cloud deployments
2. Deployment Options: Flask, FastAPI, and Cloud
“So, where should you deploy your model?”
You have three solid options:
🔹 Flask → Simple, good for quick API creation
🔹 FastAPI → Faster than Flask, built for async requests
🔹 Cloud (AWS Lambda, GCP, Azure) → Best for scalable, production-grade applications
I personally prefer FastAPI for real-time applications because of its speed and ease of use.
Code: Deploying with FastAPI
from fastapi import FastAPI, UploadFile, File
import cv2
import numpy as np
app = FastAPI()
@app.post("/predict/")
async def predict(file: UploadFile = File(...)):
image = np.frombuffer(await file.read(), np.uint8)
image = cv2.imdecode(image, cv2.IMREAD_COLOR)
# Call your text detection function
boxes = detect_text(image)
return {"detected_boxes": boxes}
# Run the server: uvicorn filename:app --reload
🔹 Why FastAPI?
✔ 50x faster than Flask
✔ Supports async processing (great for batch requests)
✔ Built-in Swagger UI for easy API testing
3. Using Docker for Scalable Deployment
“Works on my machine” doesn’t mean it works everywhere.”
If you’re deploying to multiple servers or cloud environments, Docker makes sure your model runs consistently.
Dockerfile: Packaging Your API for Deployment
FROM python:3.9
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["uvicorn", "app:app", "--host", "0.0.0.0", "--port", "8000"]
To build and run
docker build -t text-detector .
docker run -p 8000:8000 text-detector
🔹 Why Docker?
✔ Ensures consistent environment
✔ Easy to deploy on Kubernetes, AWS, GCP
✔ Scalable—just spin up multiple containers
X. Conclusion
“Every advanced technique starts with mastering the basics.”
You’ve now gone through the end-to-end process of building a robust text detection system—from preprocessing and model selection to optimization and deployment.
Here’s a quick recap of what we covered:
✅ Preprocessing: Adaptive thresholding, edge detection
✅ Text Detection Models: EAST, CTPN, DBNet, MSER
✅ Optimization: NMS, false-positive filtering, tuning
✅ Performance Evaluation: Precision, Recall, F1 Score
✅ Deployment: FastAPI, ONNX, Docker
How to Choose the Right Approach?
“There’s no one-size-fits-all solution.”
Here’s how I decide which technique to use:
Scenario | Best Approach |
---|---|
Real-time OCR (videos, live streams) | EAST + FastAPI + ONNX |
Scanned documents (clean text) | Tesseract + Adaptive Thresholding |
Noisy backgrounds (street signs, natural scenes) | DBNet + MSER + CNN |
Mobile deployment | Convert to TensorFlow Lite or ONNX |
Further Learning Resources
If you want to go even deeper, here are some resources I highly recommend:
Deep Learning for Computer Vision – Ian Goodfellow
Text Detection Papers – paperswithcode.com
FastAPI Docs – fastapi.tiangolo.com
ONNX for Model Optimization – onnx.ai
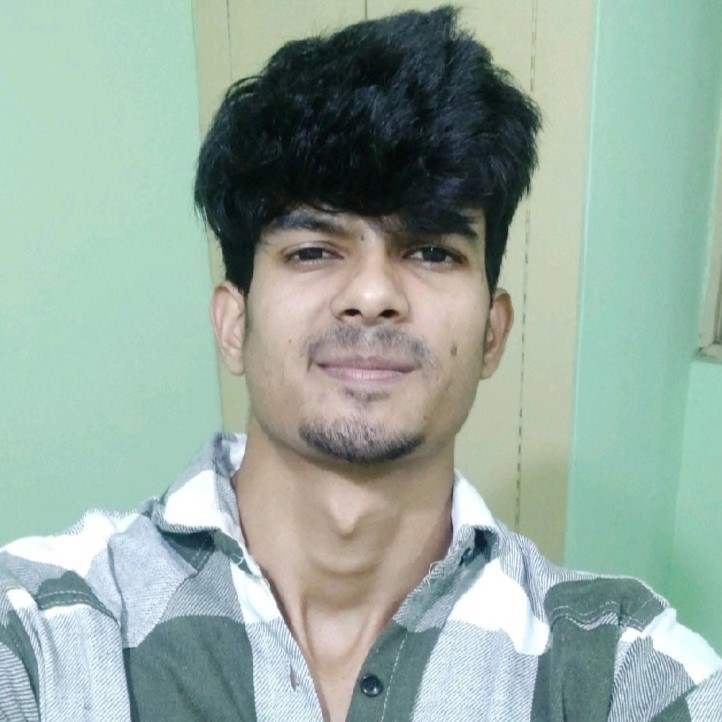
I’m a Data Scientist.