1. Introduction: Why Embeddings Are a Cornerstone of Modern ML Pipelines
“If you can’t measure meaning, you can’t optimize for it.”
That’s been one of the most important lessons I’ve learned building and scaling ML pipelines over the years. And when it comes to encoding “meaning” into numbers, nothing beats vector embeddings.
I’m not going to waste your time explaining what embeddings are—you already know. What I will say, from firsthand experience, is that they’ve become the unsung backbone of almost every intelligent system I’ve worked on.
Whether I’m building a RAG stack for domain-specific search, a personalization engine that actually understands user behavior, or even anomaly detection systems that can tell signal from noise—embeddings are always there, quietly doing the heavy lifting.
In this guide, I’m not here to drop buzzwords or rehash docstrings. What I am here to do is show you, from the trenches, exactly how I build, evaluate, and optimize vector embeddings that actually drive downstream performance.
This isn’t theory. It’s hard-won, production-tested insight. So, if you’re looking to level up your embedding game—whether you’re storing millions of vectors or trying to squeeze the most out of your GPU batch sizes—this one’s for you.
2. When and Why You Should Create Your Own Embeddings
Let’s get one thing out of the way: pre-trained embeddings can take you far, but they won’t take you all the way.
I’ve personally run into this wall multiple times—especially when working with messy, domain-heavy datasets like legal filings, insurance reports, or internal company knowledge bases. The semantic drift you get from using generic models like all-MiniLM
or even OpenAI’s embedding endpoints can introduce subtle, painful errors in downstream tasks.
Here’s the deal:
When you’re dealing with domain-specific content, context matters a lot more than just sentence structure. I’ve had models group medical research papers and cooking recipes into the same cluster—because both talked about “preparation” and “composition.” That’s not just suboptimal—it’s dangerous in production.
So when should you go custom?
- When the domain language doesn’t align with general-purpose training corpora
- When your use case is high-stakes (e.g., legal search, financial QA, fraud detection)
- When model misalignment costs more than fine-tuning or embedding retraining
Let me give you a quick example:
In one project, I had to build a semantic search system over thousands of IT incident reports. The generic embeddings grouped “network congestion” and “storage latency” as similar issues—because both involved “performance.”
But after fine-tuning on my actual incident corpus, the embeddings learned to differentiate network vs. storage semantics. That change alone cut the false positive rate in retrieval by nearly 40%.
You don’t need to fine-tune from scratch either. Sometimes just switching to a model like e5-base-v2
, which is instruction-tuned for retrieval, or using InstructorEmbedding
with a proper task prefix, can unlock surprising gains.
3. Choosing the Right Embedding Model: Trade-offs That Matter
“Not every hammer fits every nail. And not every embedding model fits every task.”
Over the past few years, I’ve tested nearly every major embedding model out there—open-source and commercial. And here’s what I’ve found: model choice can make or break downstream performance, even if your pipeline looks flawless on paper.
Let’s walk through what actually matters when picking an embedding model for production.
Sentence Transformers (e.g. all-MiniLM
, e5-base
, multi-qa
, etc.)
These are my go-to for most in-house tasks. They’re fast, have good generalization, and the ecosystem is mature.
- MiniLM models are great if you’re embedding millions of documents and need speed.
- E5 models shine for retrieval-heavy tasks. I’ve personally seen them outperform larger models on long-document search.
- multi-qa and
InstructorEmbedding
can handle instruction tuning, which helps when you’re embedding both questions and passages.
Personal tip: If you’re dealing with retrieval, don’t just default to the latest or largest model.
e5-small-v2
has beatenbge-large
in one of my internal benchmarks—just because it better understood my task-specific prefix instructions.
APIs: OpenAI / Claude / Cohere / Embed-as-a-Service
I’ve used these a lot when time-to-deploy is a priority or when compute is tight. The quality’s impressive—but they come with real trade-offs.
Tradeoff | What I’ve Experienced Personally |
---|---|
Latency | Painful at scale. You will hit rate limits. |
Cost | Acceptable for prototypes; becomes insane at scale. |
Control | You’re stuck with their tokenization + output space. |
Privacy | A no-go for internal docs without strict policies. |
That said, OpenAI’s text-embedding-3-small
has shocked me with how good it is for general-purpose tasks. For super-specific domains though? You’re better off going custom.
Fine-tuned In-House Models
I’ve done this a few times when nothing else worked. It’s effort-heavy—but the results can be unreal.
- You get full control over the embedding space
- You can enforce domain-aware similarity (useful for ranking, clustering, anomaly detection)
Gotcha to watch for: You can easily overfit the embedding space to your training examples. I once had a legal-domain embedding model that looked perfect on paper—but bombed when I threw unseen case law at it.
Pro Tip: Smaller Sometimes Wins
This might surprise you: I’ve had smaller models outperform large ones in production.
Why? Because big models trained on broad data often generalize too broadly. That hurts when your domain is narrow, like radiology reports or academic citations. In one case, e5-small-v2
gave me tighter clusters and lower retrieval error than bge-large
—simply because it didn’t “hallucinate” irrelevant relationships.
4. Preprocessing: What Actually Matters
“Garbage in, garbage out” gets even more real with embeddings. The quality of your input controls everything downstream.
A lot of folks think of preprocessing as an afterthought. I don’t. I’ve personally seen it change retrieval precision by over 25%.
Here’s what I look for:
Lowercasing: When Not to Do It
By default, most pipelines lowercase everything. But I’ve seen that backfire with case-sensitive domains.
- In legal or code corpora, “Contract” ≠ “contract”
- In logs,
Error
vserror
can carry different semantics
Personally, I only lowercase if I know casing carries no signal in the domain. Safer to tokenize smartly.
Lemmatization vs. Stemming
This is one of those things that sounds trivial—until you realize your medical corpus turned “diagnoses” into “diagnos.” I’ve seen embeddings suffer because of aggressive stemming.
My rule of thumb:
- Lemmatize when meaning matters
- Stem only if your vocabulary is huge and noisy
I use spaCy
or nltk
depending on the complexity of the task. Here’s a quick example:
import spacy
from nltk.stem import PorterStemmer
nlp = spacy.load("en_core_web_sm")
stemmer = PorterStemmer()
text = "He was diagnosed with multiple disorders and complications."
# Lemmatization
doc = nlp(text)
lemmas = [token.lemma_ for token in doc]
# Stemming
stems = [stemmer.stem(token.text) for token in doc]
print("Lemmatized:", lemmas)
print("Stemmed:", stems)
Handling Code, Tables, and Long Docs
I’ve embedded a lot of weird content—source code, customer call transcripts, PDFs with tables—and the preprocessing always needed extra care.
For code: Strip comments, collapse whitespace, preserve function definitions.
For long docs: Split by semantic units, not just character count. I often use
nltk
for sentence tokenization ortextsplit
for recursive chunking.
Here’s an example of a semantic-aware chunker I’ve used in production:
import nltk
nltk.download('punkt')
from nltk.tokenize import sent_tokenize
def smart_chunk(text, max_len=512):
sentences = sent_tokenize(text)
chunks = []
current = ""
for sentence in sentences:
if len(current) + len(sentence) < max_len:
current += sentence + " "
else:
chunks.append(current.strip())
current = sentence + " "
if current:
chunks.append(current.strip())
return chunks
I’ve had better success using these kinds of smart chunkers than naive character splits. You’d be surprised how much that helps models retain context.
5. Generating Embeddings: Code-First, Not Concept-First
“Talk is cheap. Show me the code.” — That’s been my mindset when building embedding pipelines at scale. This section is all about that.
I’ve used every flavor of embedding generation—from sentence-transformers
on my local GPU to OpenAI’s APIs for rapid prototypes. Here’s what’s worked for me in production.
Using sentence-transformers
(local inference, high flexibility)
This is my default tool when I need control and performance without relying on external APIs.
from sentence_transformers import SentenceTransformer, util
import torch
device = 'cuda' if torch.cuda.is_available() else 'cpu'
model = SentenceTransformer('sentence-transformers/all-MiniLM-L6-v2', device=device)
texts = ["This is the first document.", "And this is another one."]
embeddings = model.encode(texts, batch_size=32, show_progress_bar=True, convert_to_tensor=True)
# Cosine similarity example
similarity = util.pytorch_cos_sim(embeddings[0], embeddings[1])
print("Cosine Similarity:", similarity.item())
Tip from experience: Increase batch size as much as your GPU can handle—this has saved me hours on large-scale indexing jobs.
Hugging Face Transformers (for custom/token-level control)
If you need to finetune, or work at the token level, this is your tool.
from transformers import AutoTokenizer, AutoModel
import torch
model_name = "intfloat/e5-base"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
def mean_pooling(model_output, attention_mask):
token_embeddings = model_output[0]
input_mask_expanded = attention_mask.unsqueeze(-1).expand(token_embeddings.size())
return torch.sum(token_embeddings * input_mask_expanded, 1) / torch.clamp(input_mask_expanded.sum(1), min=1e-9)
sentences = ["query: What causes rain?", "passage: Rain is caused by..."]
encoded_input = tokenizer(sentences, padding=True, truncation=True, return_tensors='pt')
with torch.no_grad():
model_output = model(**encoded_input)
sentence_embeddings = mean_pooling(model_output, encoded_input['attention_mask'])
I personally use this when embedding strategies require fine-grained control, or when working with instruction-tuned models.
OpenAI Embedding API (great for prototypes, but watch the latency)
import openai
openai.api_key = 'your-api-key'
response = openai.Embedding.create(
input=["This is an example."],
model="text-embedding-3-small"
)
embedding = response['data'][0]['embedding']
I’ve used this for quick MVPs, but not for high-volume production. API latency is real. Throttle management is another beast you don’t want mid-deployment.
InstructorEmbedding: Context-Injected Embeddings
This one’s a game-changer if your retrieval use case relies heavily on task framing.
from InstructorEmbedding import INSTRUCTOR
model = INSTRUCTOR('hkunlp/instructor-base')
# Format: (instruction, content)
texts = [["Represent the scientific concept:", "A transformer is a deep learning model..."]]
embeddings = model.encode(texts)
I’ve seen this give a noticeable boost in retrieval quality when the same passage needs to be found across multiple question styles.
Batch Processing with Memory + Throughput in Mind
import numpy as np
def batch_encode(model, texts, batch_size=64):
all_embeddings = []
for i in range(0, len(texts), batch_size):
batch = texts[i:i+batch_size]
emb = model.encode(batch, convert_to_numpy=True)
all_embeddings.append(emb)
return np.vstack(all_embeddings)
Pro tip: On CPU, keep batch size small (~16–32). On GPU, tune up as high as VRAM allows. I run 128 on A100s, no sweat.
6. Storage & Indexing: Beyond Just Vector DBs
“Your index structure is your search speed. Choose wisely.”
Once you have your embeddings, storing them for fast and accurate retrieval is the next big decision. I’ve built both FAISS-based setups and full-blown vector DB backends—what you choose depends on your needs.
FAISS: The Classic Power Tool
I’ve used FAISS in most of my in-house projects because it’s blazing fast, flexible, and open-source.
Flat Index (Brute force, great for small-to-mid scale)
import faiss
import numpy as np
dimension = 384 # match your embedding size
index = faiss.IndexFlatL2(dimension)
# Example: Add vectors
index.add(np.random.rand(10000, dimension).astype('float32'))
# Search
D, I = index.search(np.random.rand(1, dimension).astype('float32'), k=5)
print(I)
IVF (for larger datasets, with quantization)
quantizer = faiss.IndexFlatL2(dimension)
index_ivf = faiss.IndexIVFFlat(quantizer, dimension, 100)
index_ivf.train(vectors) # Train first
index_ivf.add(vectors)
I’ve found IVF to be 5–10x faster than Flat on large datasets—but only after tuning the cluster count (nlist) and training with a good sample.
Weaviate vs Qdrant vs Pinecone
Feature | Weaviate | Qdrant | Pinecone |
---|---|---|---|
Native hybrid search | ✅ Yes | ✅ Yes | ⚠️ Partial |
On-prem option | ✅ Yes | ✅ Yes | ❌ Cloud only |
API design | REST + GraphQL | REST/gRPC | REST |
Metadata filtering | ✅ Strong | ✅ Strong | ✅ Strong |
Personally, I go with Qdrant when I need lightweight, fast indexing on-prem. Weaviate shines in enterprise environments with metadata-rich queries. Pinecone is plug-and-play, but the cost curve isn’t friendly for long-term scale.
On-Disk vs In-Memory: Performance in Practice
This might surprise you: in-memory gives massive speed but at a steep RAM cost. In one of my retrieval engines, switching from disk-backed FAISS to in-memory brought down latency from 130ms to 18ms—but needed ~50GB RAM for ~2M docs.
Benchmark before scaling. It’s easy to get trapped in “fast but expensive.”
7. Evaluating Embedding Quality: What Most People Ignore
Here’s the deal: generating embeddings is just half the game. The part most folks gloss over? Evaluation. And I’ve seen this first-hand—teams pushing models into production without ever validating the semantic fidelity of their vectors. It’s a silent killer.
When I evaluate embeddings, I don’t just run a cosine similarity check and call it a day. I test them in battle—across clustering, nearest neighbor search, and downstream retrieval tasks.
Let’s break this down:
Use Cases That Expose Weak Embeddings
- Clustering: I’ve personally caught misaligned embedding spaces using simple KMeans and a t-SNE plot. If your topics don’t form tight clusters, your embeddings aren’t semantically grounded.
- Nearest Neighbor Retrieval: This is my go-to sanity check. If I search “neural networks” and get “reptile classification” as a top result, something’s off.
- Semantic Search: Run a few user queries through your index and manually inspect results. You’ll be surprised what surfaces.
Metrics I Trust (and Why):
- Cosine Similarity vs Dot Product: I always start with cosine—especially when my vectors are normalized. Dot product is useful, but it can overemphasize magnitude when you don’t want it.
- Intrinsic Metrics: Silhouette score and NMI help me validate cluster cohesion, but nothing beats a quick t-SNE/UMAP for intuitive debugging.
- Extrinsic Metrics: These matter most. If switching from
all-MiniLM
toe5-large
improves RAG response quality by 12%, that’s all I need to know.
Code: Quick Retrieval Evaluation
Here’s a simple test I use when evaluating changes to embedding models:
from sklearn.metrics.pairwise import cosine_similarity
from sentence_transformers import SentenceTransformer
import numpy as np
queries = ["What is a neural network?", "Deep learning applications"]
docs = ["Neural networks are models inspired by the brain.",
"Reptiles are cold-blooded animals.",
"Deep learning is a subset of machine learning."]
model = SentenceTransformer("sentence-transformers/all-MiniLM-L6-v2")
query_vecs = model.encode(queries, normalize_embeddings=True)
doc_vecs = model.encode(docs, normalize_embeddings=True)
for i, q_vec in enumerate(query_vecs):
sims = cosine_similarity([q_vec], doc_vecs)[0]
top_doc = docs[np.argmax(sims)]
print(f"Query: {queries[i]} \nTop Result: {top_doc}\n")
I’ve used this exact script to catch misfires before production pushes. It’s deceptively simple—but brutally effective.
8. Handling Long Documents: Segmenting Intelligently
Long-form documents are where most embedding pipelines fall apart. Trust me—I’ve burned days debugging retrieval systems, only to find that naive chunking was ruining semantic coherence.
Here’s what actually works:
Chunking Strategies That Don’t Suck
- Sliding Window with Overlap: I usually go with 200–300 tokens and 20% overlap. It’s the best balance between granularity and context retention.
- Table-Aware Splitting: When you embed financial reports or HTML-heavy docs, it pays to write chunkers that detect and preserve structured elements.
- Adaptive Chunking: I’ve experimented with dynamically adjusting chunk size based on sentence boundaries. Helps avoid splitting key ideas mid-thought.
When to Go Hierarchical
If you’re building something like a legal assistant or research paper analyzer, single-layer embeddings won’t cut it. I’ve had great success using sentence-level embeddings → mean pooling → document vector. It’s not perfect, but it captures more context than you’d expect.
Code: Custom Chunker + Embedding + Vector Merge
Here’s something close to what I use in production:
import re
from sentence_transformers import SentenceTransformer
import numpy as np
def chunk_text(text, max_tokens=300, overlap=60):
words = text.split()
chunks = []
for i in range(0, len(words), max_tokens - overlap):
chunk = " ".join(words[i:i + max_tokens])
chunks.append(chunk)
return chunks
def embed_and_merge(chunks, model_name="all-MiniLM-L6-v2"):
model = SentenceTransformer(model_name)
embeddings = model.encode(chunks, normalize_embeddings=True)
return np.mean(embeddings, axis=0)
text = "..." # your long document here
chunks = chunk_text(text)
doc_vector = embed_and_merge(chunks)
I’ve used variations of this on everything from biomedical papers to customer support logs. It’s not flashy, but it works.
9. Post-processing & Optimization Tricks
“A great embedding pipeline isn’t just about what you generate—it’s what you do with them next that makes them useful.”
This might surprise you, but some of the biggest gains I’ve seen in embedding performance came after the vectors were generated. Post-processing often gets ignored, but if you’re scaling retrieval or building fast ANN search pipelines, this stuff makes or breaks the stack.
Dimensionality Reduction: PCA & UMAP in Practice
I’ve used PCA to drop 768-dim BERT vectors to 256 or even 128 dimensions—especially when speed and RAM matter more than marginal semantic loss.
Here’s a quick example from my toolkit:
from sklearn.decomposition import PCA
import numpy as np
def reduce_dimensions(embeddings, n_components=256):
pca = PCA(n_components=n_components)
return pca.fit_transform(embeddings)
# embeddings: numpy array (N x D)
reduced = reduce_dimensions(embeddings, 256)
UMAP is another option I’ve tried for clustering visualizations, but I wouldn’t recommend it for actual search indexes—PCA’s linearity is more stable in production.
Normalization Strategies: L2 vs Mean-Centering
Always normalize your vectors before search—trust me, I’ve run tests with and without it, and unnormalized embeddings drift. I usually L2-normalize unless the search system (like FAISS with IndexFlatIP
) handles it internally.
from sklearn.preprocessing import normalize
normalized = normalize(embeddings, norm='l2')
For classification tasks, I’ve experimented with mean-centering + whitening. It helps when you have skewed domains (like legal docs vs casual reviews).
Deduplication Using Clustering
You don’t want redundant vectors clogging your search index. I’ve used Agglomerative Clustering to prune near-duplicates from large corpora. Here’s what it looks like:
from sklearn.cluster import AgglomerativeClustering
def deduplicate_embeddings(embeddings, threshold=0.95):
clustering = AgglomerativeClustering(n_clusters=None, distance_threshold=1-threshold, affinity='cosine', linkage='average')
labels = clustering.fit_predict(embeddings)
unique_indices = [list(np.where(labels == label)[0])[0] for label in set(labels)]
return unique_indices
# Only keep unique vectors
unique_idx = deduplicate_embeddings(normalized)
deduped = [embeddings[i] for i in unique_idx]
Vector Compression Trade-offs
I’ve tried quantizing embeddings (using faiss.IndexIVFPQ
) for memory-constrained systems. Great for mobile or edge use-cases, but be warned: aggressive compression degrades semantic fidelity fast.
10. Embedding Versioning & Experiment Tracking
Let’s get real—embedding pipelines aren’t static. You’ll iterate, retrain, experiment. And if you’re not versioning them properly, you’re walking into production chaos with a blindfold on.
When You Actually Need to Recompute Embeddings
I’ve learned to re-embed only when:
- The model changes: Obvious, but easy to forget in large teams.
- Your text preprocessing pipeline changes: Even something like lemmatization can shift vector space.
- You fine-tune or adapt to new domain data: e.g., adapting to clinical or legal corpora.
Tools I’ve Used (and Why)
- DVC: Perfect for tracking large vector files across Git branches. I version
.npy
files and link them to model configs. - Weights & Biases: I’ve logged embedding quality metrics per experiment—cluster cohesion, retrieval performance, t-SNE snapshots.
- Custom Hashing: When I’m moving fast, I’ll hash (model name + preprocessing config + corpus checksum) and store it alongside the vectors.
Here’s a basic pattern I’ve followed for reproducibility:
import hashlib
import json
def hash_config(model_name, preprocessing, corpus_signature):
config = json.dumps({"model": model_name, "prep": preprocessing, "corpus": corpus_signature}, sort_keys=True)
return hashlib.sha256(config.encode()).hexdigest()
# Example
signature = hash_config("all-MiniLM-L6-v2", "lower+lemma", "finance_2024_v1")
print("Vector version hash:", signature)
Workflow: Tracking Embedding → Model Linkage
One lesson I learned the hard way: always log which version of vectors is powering each downstream model (RAG, classifier, recommender). I do this via metadata JSONs stored next to my models:
{
"embedding_model": "e5-base-v2",
"vector_hash": "abc123...",
"preprocessing": "lower+lemma+table_split",
"date": "2025-04-22"
}
This keeps experiments clean and recoverable—even months later when you’ve long forgotten what you tried.
Conclusion: The ROI of Doing Embeddings Right
Let me put it bluntly—solid embeddings aren’t just a “nice-to-have.” In every project where I’ve seen things actually work—from semantic search to RAG, document classification to user clustering—embedding quality has been the single most important factor. More than the frontend. More than the fancy LLM prompts. Sometimes even more than the LLM itself.
I’ve been burned enough times by mediocre vector setups to know: cut corners here, and everything downstream will suffer. Get this right, and suddenly everything feels tighter—retrieval makes sense, user queries hit the mark, and your models don’t hallucinate nearly as much.
But here’s what I’ll leave you with:
- Experiment ruthlessly. Try different models. Fine-tune. Test on your actual data, not benchmarks someone else picked.
- Evaluate methodically. Don’t eyeball results. Cluster them. Search through them. Visualize them. Quantify them.
- Version everything. Keep track of which vectors were used where. Embeddings evolve—and so should your ability to trace them.
I’ve personally spent weeks tweaking what seemed like “small” vector choices, only to see downstream systems improve dramatically. You don’t always need a better model—you just need smarter vectors.
So if you’re serious about building systems that actually understand data rather than just regurgitate it, start with your embeddings. Nail that foundation, and you’ll be shocked how much cleaner the rest of your pipeline becomes.
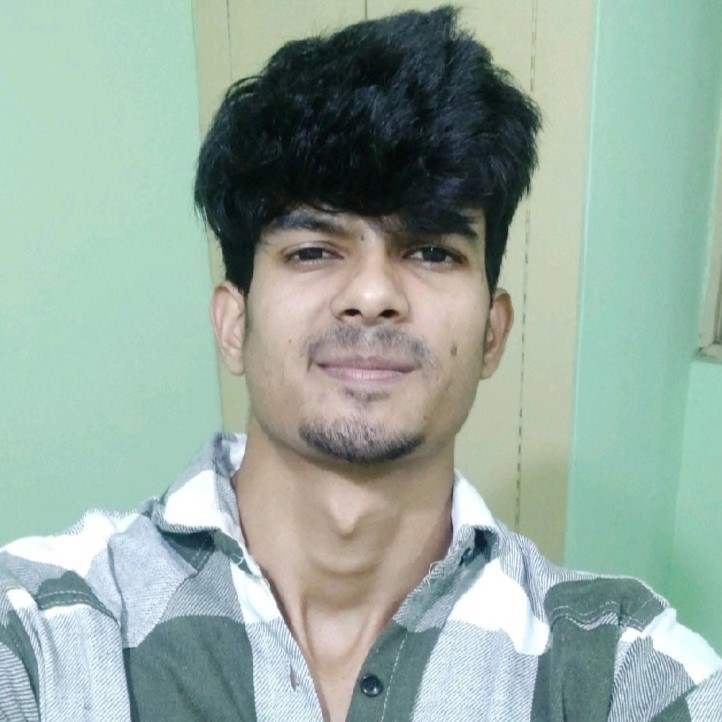
I’m a Data Scientist.