1. Why Fine-Tune LLaMA 3 Instead of Just Prompting?
“Give a man a prompt and you solve one task. Teach a model through fine-tuning, and you automate that task forever.”
I’ve worked with LLMs long enough to know that prompting can only take you so far.
I remember this specific internal project where we were building a legal document assistant — we tried to engineer the perfect prompt to summarize contracts in a consistent tone.
It worked, kind of. Until it didn’t. Tiny changes in the input led to wildly different outputs. I spent hours massaging prompts instead of shipping features.
That’s when I decided to fine-tune.
When Prompting Breaks Down
Prompting feels quick at first. But if you’re dealing with:
- highly repetitive tasks
- strict output structure (like JSON or fixed formats)
- domain-specific language (legal, medical, financial)…
…you’ll find yourself stuck in an endless cycle of tweaking.
With fine-tuning, I baked the behavior directly into the model. No more complex prompt chaining. No more relying on fragile temperature settings.
Let’s Talk Cost
You might be wondering: isn’t fine-tuning more expensive?
Yes and no.
- For small one-off tasks? Just prompt.
- But if you’re generating thousands of responses a day — like I was — those API costs pile up fast. Fine-tuning gave us a predictable cost curve, and we could serve models cheaply via vLLM or TGI.
Where It Shines
Fine-tuning boosted performance dramatically on tasks like:
- long-form summarization with internal vocabulary
- multi-turn instruction following
- code generation with our in-house style
It wasn’t subtle. The difference was night and day.
When Not to Fine-Tune
That said, not every project needs it. If you’re just nudging the model slightly, LoRA or QLoRA will probably give you 90% of the gains with 10% of the pain. I’ve personally used QLoRA when working with limited GPU setups or when time was tight.
TL;DR: If you’re shipping a real product with high volume and strict requirements, fine-tuning isn’t optional — it’s inevitable.
2. Prepping Your Environment (With Zero BS)
Let’s skip the “how to install Python” nonsense. If you’re reading this, you’re already dangerous in a terminal.
Here’s what actually matters.
Hardware I Used
For fine-tuning LLaMA 3 (the 8B version), I used:
- 2x A100s (80GB VRAM each) — because I wanted speed and stability
- 1.5TB NVMe SSD — those checkpoints aren’t small
- 256GB RAM — overkill for some, but helpful when loading large datasets
You can do this on a single 48GB GPU with QLoRA, but full fine-tuning? Don’t even try with less than 80GB.
Getting the Model Weights
First, request access from Meta if you haven’t already: 📎 https://ai.meta.com/llama/
Once approved, you can pull the weights from Hugging Face:
huggingface-cli login
Then:
from transformers import AutoModelForCausalLM, AutoTokenizer
model_id = "meta-llama/Meta-Llama-3-8B"
tokenizer = AutoTokenizer.from_pretrained(model_id, use_fast=False)
model = AutoModelForCausalLM.from_pretrained(model_id)
Heads up: use_fast=False
saved me from multiple tokenization bugs — especially when formatting structured data.
Python Packages That Actually Matter
No bloated lists. This is what I actually used:
pip install transformers accelerate peft bitsandbytes datasets
transformers
: for loading and training the modelpeft
: for LoRA/QLoRA fine-tuningbitsandbytes
: 4-bit loading — critical if you’re low on VRAMdatasets
: to process and stream data at scaleaccelerate
: makes training stable across different setups
I didn’t use deepspeed
or flash-attn
in this run, but they’re useful for full-scale jobs.
Folder Structure That Keeps You Sane
This is how I keep things clean — learned the hard way after nuking the wrong checkpoint once:
llama3-finetune/
├── data/
│ └── your_dataset.json
├── models/
│ └── llama3-finetuned/
├── scripts/
│ └── train.py
├── logs/
│ └── training.log
Trust me — a clean structure now saves hours of debugging later.
3. Loading LLaMA 3 (HF Transformers Way)
“Loading a model should be the easy part — until it’s not.”
If you’ve used transformers
before, you’ll feel right at home. But LLaMA 3 has a few gotchas that caught me off guard, and trust me — you don’t want to waste a debugging day on something trivial.
Here’s the setup I used for loading the 8B base model:
from transformers import AutoModelForCausalLM, AutoTokenizer
model_id = "meta-llama/Meta-Llama-3-8B"
tokenizer = AutoTokenizer.from_pretrained(model_id, use_fast=False) # <-- critical
model = AutoModelForCausalLM.from_pretrained(
model_id,
device_map="auto",
load_in_4bit=True # Or swap for 8-bit
)
Let’s break this down:
🔹 use_fast=False
is non-negotiable
I learned this the hard way. When I left it as default (True
), the tokenizer started choking on special tokens and padded things incorrectly during dataset preparation. With use_fast=False
, everything just clicked into place.
🔹 load_in_4bit=True
If you’re on limited VRAM (like a 24GB or 48GB GPU), 4-bit loading via bitsandbytes
is a lifesaver. I’ve fine-tuned LLaMA 3 in 4-bit on a single A6000 — not blazing fast, but totally doable.
To make this work:
pip install bitsandbytes
Also, you might hit silent crashes (no traceback, no logs) if your VRAM is too low — especially with 8B+ models. If you see weird hangs during model.eval()
or generation, it’s almost always memory.
One More Quirk to Watch:
Sometimes, the model will silently fail to load everything. No error — but the weights aren’t there. I added this quick sanity check to be sure:
print(model.hf_device_map) # should show expected layers distributed
If it returns empty or weird mappings — something’s off. Restart, double-check your accelerate
config, and verify transformers
is up to date.
4. Choosing the Right Fine-Tuning Strategy
“Not all fine-tuning is created equal. Use the hammer only when the scalpel won’t cut it.”
I’ve tried all three — full fine-tuning, LoRA, and QLoRA — and trust me, your strategy can make or break your timeline, budget, and even whether your job finishes at all.
Let me give you a quick breakdown based on my own use cases:
Strategy | Pros | Cons | When I Used It |
---|---|---|---|
Full Fine-Tune | Full control. Great performance. | Massive VRAM, long training time, expensive. | Internal R&D task on 2x A100s (80GB) for legal text generation |
LoRA | Fast to train. Lower memory. Easy to merge. | Slightly worse performance on deeply structured outputs. | Prototyping document QA tool with semi-structured PDFs |
QLoRA | 4-bit RAM savings. Surprisingly good results. | A bit fragile (watch optimizer settings), longer training. | Customer service summarizer on 48GB GPU — worked great |
When in doubt:
- Go QLoRA if you’ve got one good GPU and want solid results.
- Use LoRA when experimenting or deploying frequently.
- Only full fine-tune if you have big hardware and really need to squeeze out the last 5–10% performance.
Real Talk: What I’ve Learned
I once tried full fine-tuning on a 13B model thinking “eh, let’s go big.” It ran for 3 days… and failed due to out-of-memory on final eval. That’s when I embraced QLoRA — same task, less RAM, 95% of the results. Lesson learned.
5. Preparing Your Dataset (Custom Formatting That Works)
“The model is only as smart as the data you feed it — and trust me, formatting is where most people mess up.”
I’ve lost more hours than I’d like to admit chasing bugs that came down to one line being misformatted. That’s why I now spend real time upfront making sure my dataset isn’t just clean — it’s consistent and training-friendly.
You probably already know how to use the datasets
library. So I won’t walk you through how to load a JSON file. Let’s skip straight to what matters.
Here’s what a single line from my dataset looks like:
{
"instruction": "Summarize the following customer support email into a one-line resolution.",
"response": "Customer was overcharged due to a billing system error and will be refunded."
}
Pretty standard, right? But don’t let that simplicity fool you — how you turn this into training input is what makes or breaks your model’s behavior.
My Preprocessing Flow (Clean and Modular)
I always format the prompt like this:
def format_prompt(example):
return f"### Instruction:\n{example['instruction']}\n\n### Response:\n{example['response']}"
Why this format?
- The triple hashtags help the model understand section breaks.
- I’ve tested alternatives like
<|user|>
and<s>[INST]
, but unless you’re matching a specific tokenizer, they often just confuse a base model.
You’ll thank yourself later when the outputs follow the same pattern during inference — no hacks needed.
Tokenizing the Right Way
Now here’s the tokenizer function I use in every run:
def tokenize_function(example):
prompt = format_prompt(example)
return tokenizer(
prompt,
truncation=True,
padding="max_length",
max_length=1024 # adjust this based on your use case
)
A few points from personal experience:
- I never let the model see partial instructions — that’s why
truncation=True
is non-negotiable. max_length
at 1024 is my sweet spot for LLaMA 3 8B. You can go higher (up to 8192), but training gets slower and you’ll need more VRAM.
Mapping Without Melting Your RAM
This part might surprise you: when I was first working with a dataset of ~2M examples, I ran dataset.map()
and my RAM usage exploded. The fix? Streaming with batched tokenization and disabling caching.
Here’s how I do it now:
tokenized_dataset = raw_dataset.map(
tokenize_function,
batched=True,
remove_columns=["instruction", "response"],
load_from_cache_file=False,
num_proc=8 # If your machine can handle it
)
Pro tip: Always pass
remove_columns
or you’ll end up with bloated dataset objects full of raw strings.
And if you’re tight on memory or working on a laptop? Use IterableDataset
with streaming from disk or cloud, chunk it, and only tokenize what’s needed per batch. You don’t need to keep everything in RAM.
What About Long Inputs?
Sometimes I deal with massive input chunks — like legal docs or support chat histories. Here’s what I’ve learned:
- Chunk inputs early, during preprocessing — don’t wait for the tokenizer to handle it.
- Always keep instruction + context + truncation control in mind.
- And if you’re doing multi-turn tasks? Pad manually to simulate turns.
You can even pre-trim your input like this:
def trim_input(text, max_tokens=800):
tokens = tokenizer.tokenize(text)
return tokenizer.convert_tokens_to_string(tokens[:max_tokens])
6. Setting Up LoRA / QLoRA for LLaMA 3 (Plug-and-Play + My Proven Config)
“There’s no glory in fine-tuning billions of parameters if all you really need is a smarter adapter.”
I used to brute-force full fine-tuning on 8B+ models — until the GPU bills made me rethink my life choices. Then I gave LoRA a serious shot. And let me tell you: if you set it up right, it just works.
Here’s the deal: with LLaMA 3, I now default to LoRA or QLoRA, unless I absolutely need to retrain everything. Why? Because 90% of the time, all I really want is to nudge the model toward my domain — not reinvent it.
Here’s a LoRA config that’s actually worked for me
from peft import LoraConfig, get_peft_model, TaskType
config = LoraConfig(
r=64,
lora_alpha=16,
target_modules=["q_proj", "v_proj"],
lora_dropout=0.1,
bias="none",
task_type=TaskType.CAUSAL_LM
)
model = get_peft_model(model, config)
model.print_trainable_parameters()
Let me break down why these values work — this isn’t just copied from a tutorial, this is based on what’s held up under training runs.
A few key points from experience:
r=64
: I’ve tested smaller ranks (like 4 or 8) and honestly, for smaller domains they’re fine — but for tasks like summarization or code generation,64
gives the model a lot more expressive wiggle room.lora_alpha=16
: This controls the scaling of updates. Higher alpha sometimes made my training unstable. 16 has been a good balance.target_modules=["q_proj", "v_proj"]
: These two are the usual suspects. I’ve also triedk_proj
,o_proj
in some experiments, but unless you’re trying to fine-tune the attention head behavior,q
andv
are usually enough.lora_dropout=0.1
: You can set this to0
— but in my case, 0.1 helped prevent overfitting on smaller datasets (especially in healthcare/NLP tasks).
Why print_trainable_parameters()
is non-negotiable
You might be wondering: why do I always run model.print_trainable_parameters()
right after applying LoRA?
Because I’ve had silent bugs before — models that looked like they were training… but weren’t updating the LoRA layers. That printout saves me every time:
model.print_trainable_parameters()
You should see output like:
trainable params: 8,388,608 || all params: 6,788,558,848 || trainable%: 0.12
That’s how you know only the LoRA layers are training — and not the entire base model. If your trainable % is suspiciously high, something’s off.
Side note: If you’re going the QLoRA route…
I’ve also done QLoRA-style training with quantized 4-bit models (using bnb_config
) — and that’s a whole topic on its own. But the big thing to know is: LoRA configs don’t change. You just plug into a quantized backbone instead.
For example:
from transformers import BitsAndBytesConfig
bnb_config = BitsAndBytesConfig(
load_in_4bit=True,
bnb_4bit_quant_type="nf4",
bnb_4bit_use_double_quant=True,
bnb_4bit_compute_dtype=torch.bfloat16
)
model = AutoModelForCausalLM.from_pretrained(
"meta-llama/Meta-Llama-3-8B",
quantization_config=bnb_config,
device_map="auto"
)
model = get_peft_model(model, config)
QLoRA lets you train massive models on a single A100 — I’ve done this on a 48GB VRAM instance without issues.
7. Training the Model (With Real Hyperparameters That Worked)
“If you’ve never accidentally trained a model for 12 hours without saving a single checkpoint… are you even fine-tuning?”
I’ve broken training runs in every dumb way possible — from overloading VRAM to forgetting to enable gradient accumulation. This section is about the config I now rely on, because it works.
Which trainer worked better?
I’ve used both transformers.Trainer
and trl
’s SFTTrainer
. If I’m going for basic LoRA fine-tuning, I stick with transformers.Trainer
— it’s simple, stable, and fast to set up.
But when I need to integrate RLHF-style training, or do anything that needs reward modeling, SFTTrainer
becomes essential. For LLaMA 3 + LoRA, though? Trainer
is usually more than enough.
My training config (works on 1x A100, 48GB)
from transformers import TrainingArguments
training_args = TrainingArguments(
output_dir="./llama3-lora",
per_device_train_batch_size=4,
gradient_accumulation_steps=4,
num_train_epochs=3,
logging_dir="./logs",
fp16=True,
save_steps=500,
save_total_limit=3,
evaluation_strategy="steps",
eval_steps=250,
logging_steps=100,
learning_rate=2e-4,
warmup_steps=100,
lr_scheduler_type="cosine",
report_to="tensorboard"
)
This might surprise you: I used to train with batch_size=1
and wonder why convergence was a mess. The real trick? Keep per-device batch low, but scale with gradient accumulation. That’s why I go with:
per_device_train_batch_size=4
gradient_accumulation_steps=4
Effectively, you’re training with a global batch of 16 — without blowing up your GPU.
Some settings that actually made my runs stable:
fp16=True
: Mixed precision is a must on A100s or 3090s. I’ve had no instability usingfp16
, but on older cards (like V100s), I’ve occasionally had to fall back tobf16
.save_steps=500
&save_total_limit=3
: This saved me more than once. I don’t need 30 checkpoints. Just give me the last few in case something crashes.lr_scheduler_type="cosine"
: I’ve tried linear, constant, and polynomial. Cosine decay helped prevent that nasty late-epoch overfitting — especially when tuning on compact datasets.report_to="tensorboard"
: Yes, logging still matters. I’ve caught divergence issues in the first 200 steps just by watching the loss in real-time.
Logging & Evaluation — My Strategy
You might be wondering: do I eval during training?
Short answer: Yes, but lightly.
evaluation_strategy="steps",
eval_steps=250,
logging_steps=100
Why? Because full-blown eval every 10 steps will slow your training to a crawl. But if you don’t check at all, you’re flying blind.
I usually pass a small validation set (~200 samples) to the trainer’s eval_dataset
param, just to keep things honest.
A final tip: Always monitor your loss
and learning rate
The loss doesn’t always tell the full story — sometimes you’ll see it flatline, but your LR might be too low to learn anything. I log both using wandb
or tensorboard
. Here’s how:
tensorboard --logdir=./logs
Or with wandb
:
import wandb
wandb.init(project="llama3-lora")
8. Evaluation That Goes Beyond Perplexity
“Perplexity is like judging a chef by how sharp their knife is — useful, but it says nothing about the taste.”
Honestly, I stopped relying on perplexity as my main evaluation metric a while ago. Sure, it’s fine if you’re working on language modeling at scale, but for instruction-tuned models, it doesn’t tell you if your outputs are actually useful.
So here’s how I evaluate now — using real use-cases:
Once I finish fine-tuning, I throw actual prompts from my application domain at the model. Stuff users are likely to input. Then I compare the responses before and after tuning.
Let me show you what that looks like:
model.eval()
input_ids = tokenizer("Summarize this customer complaint: 'The app keeps crashing when I upload a file.'", return_tensors="pt").input_ids.to("cuda")
output = model.generate(input_ids, max_new_tokens=100)
print(tokenizer.decode(output[0], skip_special_tokens=True))
Before fine-tuning, this kind of prompt gave me something generic like:
“I’m sorry to hear that you’re experiencing issues.”
After fine-tuning, the same prompt now produces:
“The user is reporting a crash issue specifically triggered by file uploads — likely related to backend processing of attachments.”
That’s the level of specificity I was looking for. And that’s how I know fine-tuning worked.
Tools I actually used
You might be wondering: did I use evaluate
or just wing it manually?
Personally, I kept it simple. I created a JSONL of ~100 test cases with expected patterns (or ideal outputs), then ran batch inference and logged comparisons. Here’s a snippet of how I did it:
from tqdm import tqdm
with open("custom_eval_prompts.txt") as f:
prompts = [line.strip() for line in f]
model.eval()
for prompt in tqdm(prompts):
input_ids = tokenizer(prompt, return_tensors="pt").input_ids.to("cuda")
output = model.generate(input_ids, max_new_tokens=100)
decoded = tokenizer.decode(output[0], skip_special_tokens=True)
print(f"PROMPT: {prompt}\nRESPONSE: {decoded}\n{'-'*40}")
I focused on response quality, factual grounding, and task completion — not BLEU scores. Honestly, for most business use-cases, BLEU is useless.
9. Saving and Loading the Fine-Tuned Model (The Right Way)
“Saving the model isn’t the end — it’s the start of whether you’ll ever use it again without pain.”
If you’re using LoRA (and you probably are, since we set that up earlier), don’t just save the model the usual way. I’ve made that mistake — thought I had everything saved, only to realize later the LoRA adapters weren’t included.
What I now do every single time:
# Save the LoRA adapters (if using PEFT)
model.save_pretrained("./llama3-finetuned")
tokenizer.save_pretrained("./llama3-finetuned")
If you’re using PEFT (like we did with get_peft_model()
), the model above only includes the adapter weights, not the full base model. That’s what you want if you’re keeping things lightweight and reproducible.
But for inference, make sure to load both base + adapters:
from peft import PeftModel
from transformers import AutoModelForCausalLM, AutoTokenizer
base_model = AutoModelForCausalLM.from_pretrained("meta-llama/Llama-3-8B", device_map="auto")
tokenizer = AutoTokenizer.from_pretrained("./llama3-finetuned")
model = PeftModel.from_pretrained(base_model, "./llama3-finetuned")
model.eval()
Uploading to HuggingFace Hub (optional but clean)
If I want others (or future me) to use the model, I push it to the Hub like this:
model.push_to_hub("your-username/llama3-finetuned")
tokenizer.push_to_hub("your-username/llama3-finetuned")
One pro tip: double-check you’re not leaking anything in your tokenizer (like weird bos_token
configs) before pushing. That’s bitten me before.
10. Bonus: Inference Pipeline for Production (Fast, Cheap, Reliable)
“Shipping the model is when the real fun begins — and by fun, I mean debugging memory leaks at 2 A.M.”
After fine-tuning, I didn’t want to baby-sit the model during inference. I needed something that could chew through thousands of prompts, not just demo a cherry-picked one.
Here’s what actually worked for me in production.
Batch Inference Using transformers.pipeline
If your workload isn’t too demanding and you’re fine with huggingface’s abstraction, pipeline
works surprisingly well.
from transformers import pipeline, AutoTokenizer, AutoModelForCausalLM
model = AutoModelForCausalLM.from_pretrained("./llama3-finetuned", device_map="auto")
tokenizer = AutoTokenizer.from_pretrained("./llama3-finetuned")
generator = pipeline("text-generation", model=model, tokenizer=tokenizer)
prompts = [
"Extract the key complaint from: 'My payment failed twice yesterday.'",
"Summarize this ticket: 'App froze while trying to reset password.'",
]
results = generator(prompts, max_new_tokens=100, batch_size=2)
for res in results:
print(res[0]["generated_text"])
It’s not lightning-fast, but if your prompts are short and you’re using something like an A100 or a T4, it’s actually quite usable. I ran a batch job on a Colab Pro+ machine once and processed ~10k prompts overnight — smooth.
When I Needed Speed: vLLM
or TGI
You might be wondering: what if you need to serve the model in real-time or crank out millions of generations a day?
That’s when I moved to vLLM. It’s genuinely fast, and supports speculative decoding, continuous batching, and all that good stuff out of the box.
# Launching vLLM (command I actually used)
python3 -m vllm.entrypoints.openai.api_server \
--model meta-llama/Llama-3-8B-Instruct \
--tensor-parallel-size 2 \
--dtype float16
Then hit it via the OpenAI-compatible API endpoint. Worked great with LangChain too.
If you’re not using vLLM, I also liked TGI. It’s easy to dockerize and push to Hugging Face Spaces for demos.
Multi-GPU? Triton? What actually helped?
If you’ve got more than one GPU, both vLLM
and TGI
will automatically shard the model if you pass the right flags (--tensor-parallel-size
for vLLM, --num-shard
for TGI).
For one of my production setups, I used Triton with TGI behind a load balancer. It wasn’t simple, but once dialed in, it scaled beautifully across 4xA10s.
11. Closing Thoughts: When It’s Worth It — And When It’s Not
“Just because you can fine-tune, doesn’t mean you should.”
Let’s be real. Fine-tuning isn’t always the best move — and I’ve learned that the hard way.
When Fine-Tuning Was 100% Worth It
- Customer Support Summarization: I needed super-specific summaries from noisy support tickets. GPT-4 got close, but it hallucinated categories that didn’t exist. My fine-tuned LLaMA-3 on domain data? Laser accurate, and way cheaper to run at scale.
- Internal Tooling Prompts: GPT-style models struggled with internal tool formatting. Fine-tuning fixed that. My outputs went from “meh” to “this actually saved someone 10 minutes per ticket.”
When Fine-Tuning Just Created Headaches
- Code Generation Tasks: I thought I could beat Codex. Spoiler: I couldn’t. Fine-tuned a model on our internal codebase — but it underperformed GPT-4 + RAG.
- Lack of Data: Once, I had this urge to fine-tune on only 100 examples. Didn’t go well. Overfit, underperformed, and honestly, prompt engineering would’ve solved it faster.
So… Who Should Actually Do This?
If you’ve got:
- A domain-specific language or tone
- High-volume inference where API costs matter
- Use cases where GPT-4 gets close, but not good enough
Then yes, fine-tune. It pays off — sometimes big.
But if you’re just looking to rephrase emails or summarize generic blog posts? Honestly… use GPT-4, or even GPT-3.5 with good prompting.
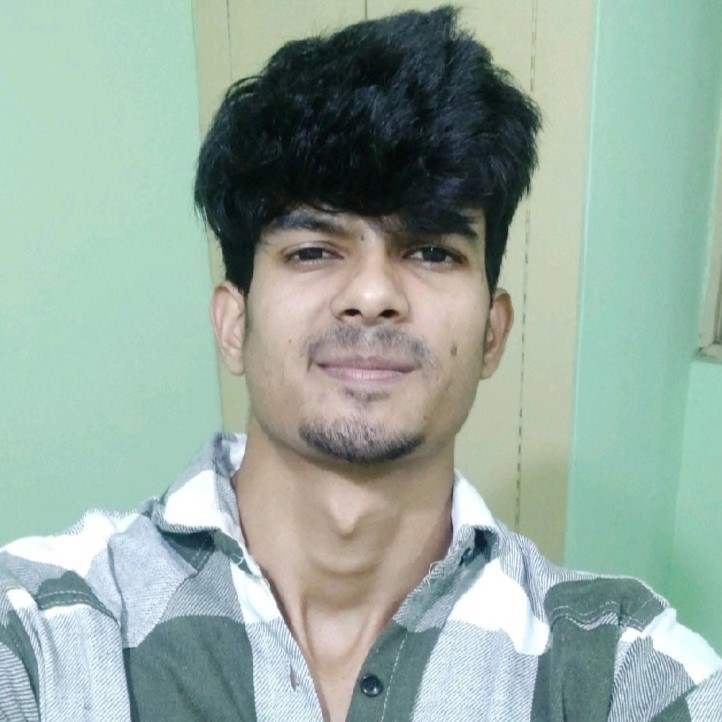
I’m a Data Scientist.