1. Why Fine-Tune LLaMA 2 Instead of Using It Out-of-the-Box?
“You don’t need a scalpel to slice bread — unless the bread is custom-made and laced with data-specific requirements.”
That’s pretty much how I explain fine-tuning to other folks on my team.
Here’s the deal: I’ve worked on projects where prompt engineering just couldn’t cut it.
The moment you start dealing with domain-heavy data — legal text, medical jargon, niche engineering formats — the general-purpose models start showing cracks.
No matter how clever your prompts are, the model still struggles to consistently stay on-format or understand your context.
Now, I’m not saying prompt engineering is useless — far from it. For prototyping or general NLP tasks, it’s often enough.
But the moment format fidelity, output consistency, or compliance becomes a hard requirement, fine-tuning becomes the only reliable option.
With LLaMA 2, the flexibility is a big win. You’re not locked into an API, so latency is low, and if you’re handling sensitive data — you can fine-tune and serve the model locally.
That was a game-changer for one project I did in the finance space — no more worrying about sending sensitive queries to an external API.
I’ll give you a breakdown of how I see the decision matrix:
Use Case | Prompt Engineering | Fine-Tuning | LoRA Fine-Tuning |
---|---|---|---|
Quick prototypes | ✅ Yes | ❌ Overkill | ❌ Overkill |
Domain-specific logic | 🚫 Struggles | ✅ Works | ✅ Works |
Fast iteration | ✅ | 🚫 Slower | ✅ Faster |
Resource-constrained hardware | 🚫 | 🚫 | ✅ Yes |
Format-sensitive output (JSON/XML) | ❌ Often fails | ✅ Reliable | ✅ Reliable |
On-prem deployment with privacy needs | ❌ | ✅ | ✅ |
Bottom line: I’ve personally moved towards LoRA-based fine-tuning with LLaMA 2 as my default for any serious, domain-heavy work. It’s more predictable, and I can bake the business logic into the model instead of hacking it in through prompts.
2. Setup: Don’t Just Install—Optimize Your Environment
Getting the environment right before training will save you hours of frustration — I’ve learned that the hard way.
Let’s skip the basic pip installs and go straight into what actually works — especially if you’re on a GPU with less than 24GB of VRAM (like the 3090 or even a T4). I’ve tried multiple setups, and here’s the one I keep going back to:
conda create -n llama2-finetune python=3.10
conda activate llama2-finetune
pip install torch==2.1.0 torchvision torchaudio --index-url https://download.pytorch.org/whl/cu118
pip install transformers datasets peft bitsandbytes accelerate
Pro tip: Make sure your CUDA version matches the PyTorch build. If you’ve ever run into that annoying “illegal instruction” or silent crashes mid-training — yeah, that’s usually the mismatch talking.
Here’s a working requirements.txt
from my last successful run:
torch==2.1.0
transformers==4.36.2
peft==0.7.1
datasets==2.16.1
bitsandbytes==0.42.0
accelerate==0.26.1
Also — just being honest here — I personally prefer conda
for these setups. It isolates GPU-specific dependencies better than pip. I’ve had too many broken wheel installs on pip when mixing CUDA versions.
If you’re on an Apple Silicon or CPU-only system… honestly, don’t bother. I tried, and it’s not worth the pain unless you’re just experimenting with the tokenizer or prepping datasets.
💡 A quick checklist I use before launching training:
- Does the GPU show up in
nvidia-smi
? - Does
torch.cuda.is_available()
return True? - Do I have
accelerate
configured with the right device map? - Is my VRAM at least 22GB for 7B? (LoRA helps, but still.)
3. Choosing the Right LLaMA 2 Variant (7B vs 13B vs 70B)
“Just because you can fit a 70B model doesn’t mean you should.”
Here’s what I’ve learned from experience — the model size decision isn’t just about raw hardware anymore. It’s a triangle of memory, training time, and quality — and you only get to pick two.
I’ve personally used all three variants (7B, 13B, 70B) across different projects. Here’s how I decide:
Model | VRAM (float16) | LoRA + 8bit VRAM | Training Time | Sweet Spot |
---|---|---|---|---|
7B | ~15GB | ~9GB | Fast | Fine-tune on a single A100 or even a 3090 |
13B | ~25GB | ~15GB | Slower | Better reasoning + format adherence |
70B | ~80GB+ | ~45GB+ (LoRA) | Painfully long | Only when you really need coherence in complex tasks |
Don’t underestimate 13B.
You can run it with quantization + LoRA on consumer GPUs — I’ve done it on a Colab Pro with T4s using bitsandbytes
. It’s just slower, but not impossible. And in return, it gives noticeably better performance on structured tasks (like JSON or SQL generation). You get less hallucination, too.
You might be wondering: “Is 70B even practical?” Personally, I’ve only used 70B when I had multiple A100s or cloud credits to burn. Even inference becomes a challenge unless you split across GPUs. It’s great, but unless you’re building a production-grade assistant or tackling abstract reasoning, 13B hits the balance.
💰 Cost vs Performance Tip:
For most business-facing tasks, 13B with LoRA gives 85% of 70B’s performance with a fraction of the footprint.
4. Data Preparation: The Stuff Most Guides Gloss Over
“Garbage in, garbage out” — but in fine-tuning, even slightly misformatted gold can blow up your training.
I’ve spent more time fixing data than training models — and I wish more people would talk about this.
Let me walk you through how I actually prep data for instruction fine-tuning:
Format: Why JSONL > Everything Else
I’ve tried CSVs, Parquet, even SQL exports — but JSONL
wins every time for one simple reason: streaming + structure. Each line is a standalone example, and tools like Hugging Face Datasets love that.
from datasets import load_dataset
dataset = load_dataset("json", data_files={
"train": "data/train.jsonl",
"validation": "data/val.jsonl"
})
Pro tip: Avoid deeply nested fields. Keep it clean: {"instruction": ..., "input": ..., "output": ...}
.
Tokenization Strategy
This is where I see folks trip up — should you tokenize in advance or let the trainer handle it?
I let the Trainer
or SFTTrainer
handle tokenization on-the-fly using a tokenizer loaded with trust_remote_code=True
. Why? Because if the tokenizer or pad_token_id
mismatches your model, you’ll get cryptic runtime errors. I’ve lost half-days to that.
Still, here’s how you’d do manual tokenization if you need it (for dataset caching or debugging):
from transformers import AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("meta-llama/Llama-2-7b-hf", trust_remote_code=True)
def tokenize(example):
return tokenizer(
f"### Instruction:\n{example['instruction']}\n\n### Input:\n{example['input']}\n\n### Output:\n{example['output']}",
truncation=True,
padding="max_length",
max_length=1024
)
tokenized_dataset = dataset.map(tokenize)
Cleaning: Real-world Strategies
Here’s what I actually do with messy datasets:
- Remove examples with output length < 20 tokens (they add noise).
- Strip HTML, markdown, weird formatting — especially from scraped data.
- Normalize quotes (
“
→"
), dashes (–
→-
), etc. - Drop examples where instruction is similar to input (often duplication or poor labeling).
I usually run a script like this before saving my JSONL:
def clean(sample):
if len(sample['output']) < 20:
return None
sample['instruction'] = sample['instruction'].strip().replace("“", '"')
sample['input'] = sample['input'].strip()
sample['output'] = sample['output'].strip()
return sample
cleaned = [clean(s) for s in raw_data if clean(s)]
Example: One Good Training Sample
{
"instruction": "Summarize the following research abstract.",
"input": "In this paper, we propose a novel attention-based mechanism for...",
"output": "The paper introduces an attention mechanism that improves..."
}
Don’t overthink format — just make sure it’s clear, consistent, and represents the task you’re targeting.
5. LoRA + PEFT = Why You’re Not Training from Scratch
“You don’t need to retrain the whole brain just to teach it one new trick.”
When I first started fine-tuning LLaMA 2, I quickly realized full finetuning wasn’t just expensive — it was overkill. Unless you’re doing research-level work or trying to squeeze the last 2% accuracy, you’re better off with LoRA (Low-Rank Adaptation).
So here’s what I actually use:
from peft import LoraConfig, get_peft_model, TaskType
lora_config = LoraConfig(
r=8,
lora_alpha=32,
target_modules=["q_proj", "v_proj"],
lora_dropout=0.05,
bias="none",
task_type=TaskType.CAUSAL_LM
)
I usually stick with r=8
and alpha=32
. You can go lower for memory savings, but I’ve found this config gives a solid balance — especially for models like LLaMA 2 7B and 13B.
You might be wondering: “Why only
q_proj
andv_proj
?” From my own runs, modifying those two gives you most of the gains without ballooning memory usage. When I addedk_proj
oro_proj
, I barely saw improvement, but memory shot up fast — especially on single-GPU setups.
Why PEFT Makes All This Click
If you’re using HuggingFace’s transformers
, you’ll need to bring in the PEFT library to glue LoRA to the model. I’ve seen a lot of folks struggle with getting the two to play nicely.
Here’s the minimal setup that works for me:
from transformers import AutoModelForCausalLM, AutoTokenizer
model = AutoModelForCausalLM.from_pretrained(
"meta-llama/Llama-2-7b-hf",
load_in_8bit=True,
device_map="auto"
)
model = get_peft_model(model, lora_config)
Make sure you load with load_in_8bit=True
if you’re running on a 3090 or T4. On A100s, I usually skip that and go bfloat16
instead. But either way, LoRA cuts memory usage down by 70–80%, no exaggeration.
Real-World Issue: Size Mismatch Errors
This one hit me early on:
RuntimeError: Error(s) in loading state_dict for LlamaForCausalLM...
size mismatch for model.layers.0.self_attn.q_proj.lora_A.weight...
It happens when:
- You change the
target_modules
between training and loading - You load LoRA weights into the wrong base model
- Your model’s architecture isn’t exactly the same
My fix: Always save and log the full LoraConfig
and base model name in a metadata file. I even include the SHA hash of the base model directory sometimes. It saves me hours later.
Optional: Save + Load Your LoRA Model
Saving just the LoRA weights is simple:
model.save_pretrained("lora-llama2-7b")
tokenizer.save_pretrained("lora-llama2-7b")
Then to reload it:
from peft import PeftModel, PeftConfig
config = PeftConfig.from_pretrained("lora-llama2-7b")
base_model = AutoModelForCausalLM.from_pretrained(config.base_model_name_or_path)
model = PeftModel.from_pretrained(base_model, "lora-llama2-7b")
This lets you keep your fine-tuned adapters lightweight (under 500MB), and plug them into any matching base model later.
Final Thought
I’ll be honest — once I started using LoRA + PEFT, it changed the game for how fast and cheap I could iterate. You can fine-tune thousands of examples in under an hour, even on modest hardware, and your model stays sharp.
6. Fine-Tuning with transformers
Trainer + PEFT
“Give me six hours to chop down a tree, and I’ll spend the first four setting up my Trainer config.”
I’ve run fine-tuning jobs that melted my GPU… and others that quietly underfit for three days without learning a thing. The difference almost always came down to how I set up my Trainer — the not-so-glamorous plumbing work.
Let’s go step-by-step, but from a place of actual experience — what I’ve personally found to matter when combining HuggingFace transformers
with PEFT + LoRA.
The Basic Wiring (Model → Tokenizer → Dataset → Trainer)
Here’s a minimal but practical setup that works for most LLaMA 2 fine-tuning tasks using LoRA adapters:
from transformers import AutoTokenizer, AutoModelForCausalLM, Trainer, TrainingArguments
from peft import get_peft_model, LoraConfig, TaskType
from datasets import load_dataset
# Load base model + tokenizer
model_name = "meta-llama/Llama-2-7b-hf"
tokenizer = AutoTokenizer.from_pretrained(model_name, trust_remote_code=True)
tokenizer.pad_token = tokenizer.eos_token
model = AutoModelForCausalLM.from_pretrained(
model_name,
load_in_8bit=True,
device_map="auto"
)
# Add LoRA on top
lora_config = LoraConfig(
r=8,
lora_alpha=32,
target_modules=["q_proj", "v_proj"],
lora_dropout=0.05,
bias="none",
task_type=TaskType.CAUSAL_LM
)
model = get_peft_model(model, lora_config)
# Load your fine-tuning dataset
dataset = load_dataset("json", data_files={"train": "data/train.jsonl", "validation": "data/val.jsonl"})
This works well for most setups on A100, T4, and 3090 — as long as you’re using 8-bit loading (bitsandbytes
) or BF16, which I’ll come to in a sec.
The Trainer Config that Actually Moves the Needle
training_args = TrainingArguments(
output_dir="./results",
per_device_train_batch_size=4,
gradient_accumulation_steps=4,
learning_rate=2e-5,
num_train_epochs=3,
logging_dir="./logs",
logging_steps=50,
save_strategy="steps",
save_steps=500,
evaluation_strategy="steps",
eval_steps=500,
save_total_limit=2,
fp16=True, # or bf16=True depending on GPU
gradient_checkpointing=True,
lr_scheduler_type="cosine",
warmup_steps=100
)
Let me walk you through a few that matter from experience, not from theory:
gradient_accumulation_steps=4
: This is your workaround when you can’t fit larger batch sizes. I’ve pushed this to 8 or even 16 on a 3090, depending on memory.fp16=True
vsbf16=True
: If you’re on A100 or newer GPUs with BF16 support, bf16 is the way to go. It’s more stable and requires less fiddling. On 3090s or T4s, go withfp16
.gradient_checkpointing=True
: This saved me from OOM errors more times than I can count. But heads up — it slows training down a bit.cosine
scheduler + warmup: I’ve had smoother convergence with this than withlinear
. It just plays nicer with larger models.
OOM Errors and What Actually Helps
You’re gonna hit these. It’s not “if”, it’s “when.” Here’s how I usually escape:
- Enable
gradient_checkpointing
— hands down the most impactful flag for reducing memory. - Use
load_in_8bit=True
(frombitsandbytes
) if you’re tight on VRAM — especially on consumer GPUs. - Drop batch size and increase
gradient_accumulation_steps
— no shame in running microbatches if you’re memory-constrained.
When none of that works, I’ve even pruned layers or reduced LoRA r
values mid-experiment to keep it going.
Training It All Together
Assuming your dataset is already tokenized properly (don’t worry, we’ll tackle tokenization quirks later), training is as simple as:
trainer = Trainer(
model=model,
args=training_args,
train_dataset=dataset["train"],
eval_dataset=dataset["validation"]
)
trainer.train()
Personally, I always monitor loss manually from the logs instead of relying on fancy dashboards. In tight loops, I like staying close to the ground.
7. Logging, Tracking, and Debugging: Lessons from Pain
“What you don’t track, you can’t fix.” — Every person who’s lost a 3-day training run to NaNs.
I learned this the hard way. Early in my fine-tuning experiments, I had a model that silently tanked mid-training. No crash. No loss spike. Just… quietly stopped learning. That was the moment I stopped treating experiment tracking as optional.
Let me walk you through what’s actually helped me — not theory, just what’s saved my time (and sanity).
wandb
vs tensorboard
: What Actually Works?
I’ve used both. If you’re just after loss curves and metrics, tensorboard
is dead simple. But for real-world training — where you’re juggling configs, GPU types, LoRA settings, and dataset versions — I always end up using Weights & Biases (wandb
).
Why? Because it lets you track everything, not just scalar metrics. Here’s what I typically log:
- Hyperparams (batch size, learning rate, LoRA config)
- GPU utilization over time
- Custom metrics (e.g., JSON parse success rates for outputs)
- Example generations (before & after fine-tuning)
import wandb
# Initialize tracking
wandb.init(
project="llama2-finetuning",
config=training_args.to_dict(),
name="7b-lora-r8-causal",
)
Then just set report_to="wandb"
in your TrainingArguments
, and you’re good to go.
training_args = TrainingArguments(
...,
report_to="wandb",
)
This might seem obvious, but log example generations early and often. Loss might go down — but if your outputs still hallucinate or misformat, you’ll catch it faster by inspecting the samples.
Loss Spikes: When to Panic (and When Not To)
Loss isn’t linear. Especially with instruction tuning or noisy data, you’ll see weird spikes. Not every spike is worth worrying about.
From what I’ve seen, here’s the pattern:
- Early training spikes? Totally normal. Probably just warming up.
- Mid-training spike + never recovers? That’s when I dig in. Could be:
- Bad batch (especially if using JSONL and you didn’t pre-validate inputs)
- Learning rate too high
- Mixed precision instability (if not using
bf16
)
I’ve had success reducing learning_rate
from 2e-5
to 1e-5
when spikes become frequent near the halfway point.
Silent Crashes: My Most Hated Bug
This one took me a while to catch: training jobs that just hang or die quietly, no error, no logs. What helped me debug:
- Use
deepspeed
oraccelerate
with verbose logging — especially for multi-GPU setups. - Watch
nvidia-smi
like a hawk. If one process stalls at 100% but others idle, it’s likely a deadlock. - Try running with
CUDA_LAUNCH_BLOCKING=1
to force errors to show up properly. - If using LoRA with
load_in_8bit=True
— mismatched device maps or improperly sharded models can silently hang. Always double-check device placement with:
model.hf_device_map
8. Evaluation: Don’t Just Look at Loss
“You don’t need 5 decimal places of loss — you need one sample that actually makes sense.”
Loss is a rough proxy. But I’ve seen models with great loss numbers output absolute garbage — malformed JSON, broken instructions, or wrong answers altogether.
So here’s how I evaluate models in practice — especially for generation-heavy tasks.
Custom Eval Functions: What I Actually Measure
Let’s say I’m fine-tuning on a domain-specific task — like generating structured JSON from prompts. BLEU and ROUGE are useless here. What I really care about is:
- Does the output match required keys?
- Can the JSON be parsed?
- Does it answer the prompt correctly?
Here’s a simple eval function I use for JSON format validity:
import json
def json_validity_score(outputs):
success = 0
for out in outputs:
try:
_ = json.loads(out)
success += 1
except:
pass
return success / len(outputs)
Pair this with simple keyword checks or regex patterns for your domain.
Sample Outputs: Before vs After Fine-Tuning
This is my go-to sanity check — just generate side-by-side outputs from the base model and the fine-tuned one.
from transformers import pipeline
pipe = pipeline("text-generation", model=model, tokenizer=tokenizer)
prompt = "Generate a valid JSON object describing a user profile."
# Before fine-tuning
base_output = pipe(prompt, max_new_tokens=200)
# After fine-tuning
finetuned_output = pipe(prompt, max_new_tokens=200)
print("Base Model Output:\n", base_output[0]['generated_text'])
print("\nFine-Tuned Model Output:\n", finetuned_output[0]['generated_text'])
This alone can surface 90% of fine-tuning issues: weird formatting, irrelevant answers, or hallucinations.
Real-World Metrics > Academic Scores
For instruction-tuned models, I often use:
- Exact match accuracy (if outputs are deterministic)
- Fuzzy match with scoring functions like
SequenceMatcher
- Custom test sets with expected answers — manually curated
If you’re deploying to production, your evaluation needs to mimic your end task, not just measure string similarity.
9. Saving + Loading the LoRA-Injected Model
“You don’t really own the model until you’ve saved it properly.”
There’s a difference between finishing a fine-tuning run and actually being ready to deploy. Trust me, I’ve learned this lesson after accidentally losing several models when I didn’t save them properly. So here’s how I make sure everything is saved, ready to go, and in the right format for sharing or deploying.
Merging LoRA Weights into the Base Model
Now, this might be obvious to some, but the first time I tried fine-tuning with LoRA, I almost didn’t realize I had to merge the LoRA weights back into the base model before saving. You can’t deploy a model that only has the LoRA weights injected into it; you need the full, merged model for inference.
Here’s the part I’m talking about:
from peft import merge_and_unload
# Merge the LoRA weights into the base model
model = merge_and_unload(model)
# Now save the model
model.save_pretrained("llama2-finetuned")
I’ve made the mistake of skipping this before, thinking the weights were already there. Turns out, the model needs to be fully merged with the LoRA components before you save it, especially if you’re going to load it later for inference or upload it to Hugging Face.
Uploading to Hugging Face: Pitfalls to Avoid
This might surprise you: Uploading models to Hugging Face isn’t as straightforward as just saving and pushing. If you don’t clean up your model properly before uploading, you risk cluttering your model repository with unnecessary files or, worse, uploading broken versions.
Here’s a checklist that I’ve picked up:
- Ensure LoRA layers are merged: If you forget to merge the LoRA weights back into the model, the Hugging Face Hub won’t recognize the changes.
- Remove unnecessary files: Always check if your model folder has redundant files before pushing.
- Use the
transformers-cli
for uploading: You could use thehuggingface_hub
library, but I findtransformers-cli
to be a smoother experience.
Here’s a small reminder for uploading:
transformers-cli login
transformers-cli upload --repo "username/repo-name" --path "./llama2-finetuned"
That’ll get your model on the hub, but always double-check the model’s README to provide detailed instructions for others who might want to use it.
Using the Fine-Tuned Model for Inference
Once your model is merged and saved, it’s time to bring it back into the pipeline for inference. Here’s the part that can sometimes trip people up — using the fine-tuned model in a pipeline()
call.
The pipeline API works seamlessly, but when you’ve fine-tuned a model, you want to make sure you load the right version — i.e., the model with LoRA weight adjustments or the merged model.
from transformers import pipeline
# Load your model and tokenizer
model = model.from_pretrained("llama2-finetuned")
tokenizer = tokenizer.from_pretrained("llama2-finetuned")
# Use the pipeline for text generation
pipe = pipeline("text-generation", model=model, tokenizer=tokenizer)
# Generate some text
output = pipe("What is the meaning of life?", max_new_tokens=100)
print(output)
Here’s the deal: make sure you’re using the exact same tokenizer that you fine-tuned with. Otherwise, you might end up with unexpected results or formatting issues.
10. Deploying the Fine-Tuned Model
“Fine-tuning is only half the journey; deployment is where the rubber hits the road.”
Once the model is trained, merged, and ready to go, it’s time to deploy. This step can feel like a whole new world if you’re not careful about the deployment tools you use.
Inference using pipeline()
with Optimized Configs
For inference, the text-generation pipeline is your go-to. But don’t overlook configuration optimizations that can make a massive difference in performance.
When you’re deploying to production, I always optimize the inference pipeline like this:
from transformers import pipeline
# Use batch generation
pipe = pipeline(
"text-generation",
model="llama2-finetuned",
tokenizer="llama2-finetuned",
device=0, # Use GPU
config={"max_length": 200, "num_beams": 5, "temperature": 0.7}
)
I’ve found that beam search with num_beams=5
strikes the right balance between quality and performance for most use cases. But again, it really depends on your task. For faster, lower-latency scenarios, reduce num_beams
or even go greedy.
Real-World Inference Benchmarks (with and without Quantization)
Now, here’s where I really started optimizing: inference speed. Quantization can make a world of difference in reducing model size and speeding up inference, but you have to balance that with potential loss in accuracy.
Here’s the real takeaway from my experience: FP16 and BF16 are great for reducing memory overhead, but quantization (e.g., 8-bit
) is what really drops inference times. Here’s an example of how I set that up:
from transformers import AutoModelForCausalLM, AutoTokenizer
# Load quantized model
model = AutoModelForCausalLM.from_pretrained(
"llama2-finetuned",
load_in_8bit=True
)
# Setup tokenizer
tokenizer = AutoTokenizer.from_pretrained("llama2-finetuned")
# Create pipeline for faster inference
pipe = pipeline("text-generation", model=model, tokenizer=tokenizer, device=0)
You’ll get noticeably faster generation times without sacrificing much accuracy. Test both — and keep a close eye on the trade-offs, especially for tasks where you need more precision.
Serving with vLLM or TGI: Pros/Cons
When you go to serve your model, you have a choice of frameworks: vLLM or TGI (TensorFlow Serving). Here’s how I think about each:
- vLLM: Best for production environments with multiple GPUs, low latency, and high throughput. It has a more streamlined architecture for large-scale deployments.
- TGI (TensorFlow Serving): If you’re using TensorFlow models or need a more generic serving solution. It’s reliable, but not as optimized for Hugging Face models compared to vLLM.
I typically choose vLLM for models like LLaMA, where throughput and latency are a priority.
Final Thoughts & Best Practices
As I wrap up this guide, I want to leave you with a few critical lessons I’ve learned from working with fine-tuning, deployment, and real-world optimization. These insights come from hard-earned experience, and I think they’ll help you make the right choices for your own projects.
When to Stop Fine-Tuning and Start RLHF / DPO
You might be wondering: When should I stop fine-tuning and transition to more advanced techniques like RLHF (Reinforcement Learning with Human Feedback) or DPO (Direct Preference Optimization)?
Here’s my take: Fine-tuning is great for adapting a model to your specific task, but it has its limits. If your goal is to teach the model to respond better to nuanced human preferences or optimize its behavior based on human feedback, RLHF or DPO are the way to go.
Here’s when to make the jump:
- Stop fine-tuning when your model reaches a plateau. You’ve tuned your model, and it’s giving solid results, but those incremental gains start to shrink. That’s when you know it’s time to step up the game.
- Start RLHF/DPO when you need to optimize for human-like decisions. If your model’s output needs to align more closely with human intuition or feedback (think conversational AI, recommendations), these methods can push performance further by learning directly from human feedback.
From my own experience, RLHF can be a game-changer when you need your model to “understand” human context, but it takes time and computational resources. So, always consider if the marginal benefits are worth the investment.
Why Smaller, Domain-Tuned Models Often Beat Bigger, Generic Ones
I’ve had plenty of chances to experiment with both large, general-purpose models (like GPT-3 or LLaMA) and smaller, domain-specific models. And let me tell you, smaller models tuned for a specific task can often outperform their bigger counterparts — and by a significant margin.
Here’s why:
- Task Specialization: A model that’s fine-tuned on a specific domain or task can focus all its capacity on understanding the nuances of that domain. It doesn’t need to generalize as much, which means it can deliver more accurate and relevant results.
- Efficiency: Smaller models are typically faster, require less memory, and are easier to deploy. Fine-tuning these models with the right dataset means you can get great performance without the overhead of dealing with massive model weights.
- Avoiding Overfitting: Larger models tend to have a lot of parameters, which means they can overfit if not tuned correctly. Smaller models, on the other hand, often generalize better when fine-tuned with the right dataset.
In my experience, especially with production models, I’ve consistently seen that fine-tuning smaller models for specific tasks yields better results both in terms of performance and efficiency.
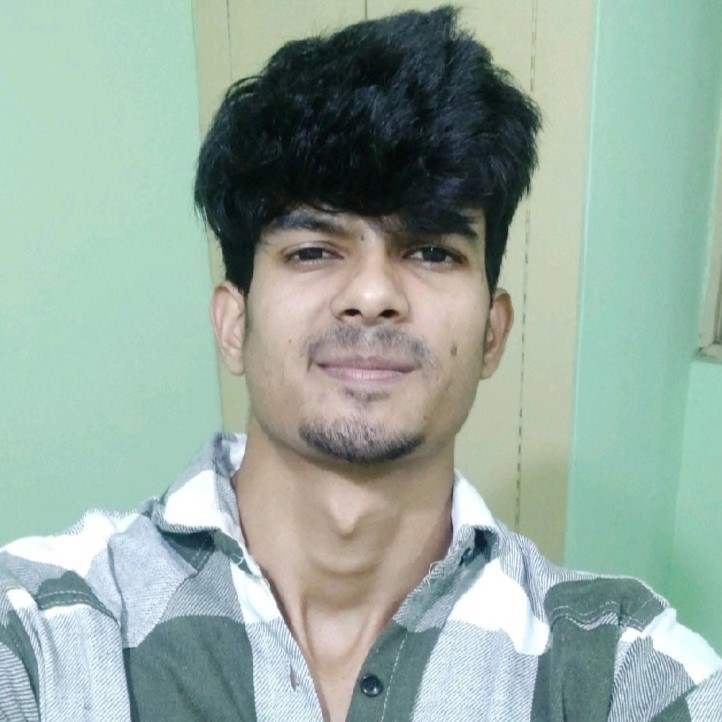
I’m a Data Scientist.