1. Introduction
“The best way to predict the future is to create it.” – Peter Drucker
I’ve spent a lot of time fine-tuning large language models, and let me tell you—Falcon 40B is an absolute powerhouse. But like any massive model, getting it to work efficiently for a specific task is where things get tricky. You can either throw brute-force compute at it (which gets expensive fast) or fine-tune it intelligently.
Why Fine-Tune Falcon 40B?
You might be wondering: Why even bother fine-tuning Falcon 40B when it’s already trained on massive datasets?
Here’s the deal—while Falcon 40B is a fantastic generalist model, it lacks domain-specific precision. If you’re working in finance, legal, scientific research, or any niche field, you need a model that understands the context deeply.
Personally, I’ve found that zero-shot or even few-shot inference often falls short when handling specialized queries. Fine-tuning bridges this gap by:
- Adapting the model to your dataset (scientific papers, legal texts, financial reports)
- Improving response accuracy on domain-specific tasks
- Reducing hallucinations, especially in critical applications
- Boosting efficiency, so the model can perform better with fewer tokens
Now, let’s talk about the cost-benefit analysis.
Fine-Tuning vs. API-Based Solutions
I’ve worked with API-based models before, and while they’re great for rapid prototyping, they come with limitations:
- API-based solutions → Quick to deploy, but expensive at scale and limited in customization.
- Fine-tuning Falcon 40B → More upfront work, but gives you full control and cost savings in the long run.
In my experience, if you’re working on a long-term project that requires precision, fine-tuning is the way to go.
What This Guide Covers
I won’t bore you with theory. This guide is all about practical implementation—every step, every code snippet, and every optimization technique you need to fine-tune Falcon 40B efficiently. Here’s what you’ll get:
- End-to-end setup: Hardware, dependencies, dataset preparation, training, evaluation, and deployment.
- Optimized configurations: Avoid OOM errors and optimize for memory usage.
- Code implementations: Every section comes with detailed, working code.
If you’re ready to fine-tune Falcon 40B like a pro, let’s dive into the system requirements.
2. System Requirements & Environment Setup
“Give me six hours to chop down a tree, and I will spend the first four sharpening the axe.” – Abraham Lincoln
When fine-tuning Falcon 40B, your setup makes or breaks your workflow. I’ve learned this the hard way—choosing the wrong hardware can slow down training by hours or even days. Worse, an inefficient environment setup can lead to OOM (out of memory) errors, wasted compute, and unnecessary headaches.
Let’s get straight to the point: here’s the ideal setup for fine-tuning Falcon 40B efficiently.
2.1 Hardware Requirements
Fine-tuning a 40-billion parameter model isn’t something you do on a gaming laptop (trust me, I’ve tried). You need serious hardware.
Which GPU Should You Use?
Here’s a breakdown of what works best:
GPU | VRAM | Suitability | My Thoughts |
---|---|---|---|
A100 (80GB) | 80GB | Best for single-GPU fine-tuning | My go-to choice. Handles LoRA/QLoRA with ease. |
H100 | 80GB | More power-efficient than A100 | Great, but expensive. Overkill for LoRA. |
4x RTX A6000 | 48GB each | Multi-GPU fine-tuning | Works well, but needs DeepSpeed ZeRO. |
TPUv5 | Variable | Works with JAX & Flax | If you’re comfortable with Google Cloud TPUs. |
My recommendation?
- LoRA/QLoRA: A single A100 (80GB) works great.
- Full fine-tuning? At least 4x A100s or TPUs, but prepare for massive costs.
CPU & RAM Considerations
You don’t need a crazy CPU, but RAM matters. I’ve seen models crash at tokenization because of low RAM.
- Minimum RAM: 64GB
- Ideal RAM: 128GB+ (if working with large datasets)
Storage & IOPS Requirements
If your dataset is huge (think 1TB+), storage speed becomes a bottleneck.
- SSD/NVMe (Recommended): Faster data loading → fewer training slowdowns.
- HDD (Avoid): Will cripple data loading speeds.
- Cloud Storage (If Remote Training): Google Cloud’s Persistent Disks or AWS EBS (gp3) are decent choices.
2.2 Setting Up the Environment
Now that you’ve got the hardware ready, it’s time to set up the software environment.
Installing Dependencies
I always recommend using a clean Docker container or Conda environment to avoid dependency conflicts.
Check GPU Availability
Before you do anything, confirm your GPU is detected:
nvidia-smi
If you don’t see your GPU listed, stop here—your drivers might not be installed.
Install Required Python Packages
Let’s install everything Falcon 40B needs:
!pip install torch transformers accelerate deepspeed bitsandbytes datasets peft trl wandb
Here’s what each package does:
- torch → PyTorch (the backbone of training)
- transformers → Falcon 40B model & tokenizer
- accelerate → Helps with multi-GPU setup
- deepspeed → Memory optimization for training
- bitsandbytes → Enables 8-bit & 4-bit quantization
- datasets → Efficient dataset handling
- peft → Parameter-efficient fine-tuning (LoRA, QLoRA)
- trl → Hugging Face’s RLHF training tools
- wandb → For logging & monitoring
Verify Installation
Before moving forward, make sure everything is installed correctly:
import torch
print(torch.cuda.is_available()) # Should return True
If this returns False
, something is wrong with your CUDA setup.
Docker vs. Conda vs. Virtualenv
I’ve tried all three setups, and here’s my honest take:
Method | Pros | Cons |
---|---|---|
Docker | Isolated, reproducible | Slightly complex setup |
Conda | Easy to manage dependencies | Can have conflicts |
Virtualenv | Lightweight | Lacks GPU-specific optimizations |
Personally, I prefer Docker when working on cloud servers and Conda when working locally.
Final Thoughts
Setting up Falcon 40B correctly saves you from a world of pain later. I’ve had training runs crash after hours simply because I ignored a minor setup issue. Follow these steps, and you’ll be fine-tuning without frustration.
Next up: Preparing your dataset and tokenization!
3. Dataset Preparation & Tokenization
“A model is only as good as the data it learns from.” – Every ML Engineer Ever
When I first started fine-tuning LLMs, I made the mistake of thinking any dataset would work. I learned the hard way—garbage in, garbage out. If you don’t prepare your dataset properly, your model will memorize noise, generate irrelevant text, or worse, hallucinate nonsense.
In this section, I’ll walk you through how to choose the right dataset, clean it, and tokenize it for Falcon 40B so your fine-tuning process is smooth and efficient.
3.1 Choosing the Right Dataset
Picking the right dataset isn’t just about downloading something from Hugging Face and calling it a day. It has to be aligned with your use case, clean, and formatted correctly.
Here’s how I approach it based on the task at hand:
Task | Recommended Datasets | Why? |
---|---|---|
Chatbot fine-tuning | OpenAssistant, ShareGPT | Human-like conversational data |
Legal/Finance/NLP | SEC filings, ArXiv papers | Domain-specific text |
Multilingual models | OSCAR, CC-100 | Diverse language coverage |
Cleaning Raw Data
Once you have a dataset, don’t assume it’s clean. I’ve had models fail mid-training because of inconsistent text formatting, artifacts, or imbalanced labels.
Here’s my quick checklist before using any dataset:
- Remove artifacts: Strange characters, HTML tags, excessive whitespace.
- Check balance: Ensure class distribution isn’t heavily skewed.
- Filter out duplicates: Avoid redundant training examples.
- Normalize text: Convert everything to lowercase (unless case sensitivity is required).
You can use Pandas for basic cleaning:
import pandas as pd
df = pd.read_csv("dataset.csv")
# Remove empty rows
df = df.dropna()
# Remove duplicates
df = df.drop_duplicates()
# Normalize text
df["text"] = df["text"].str.lower()
df.head()
3.2 Tokenization & Data Preprocessing
Once your dataset is clean, it’s time to tokenize it.
Using Falcon’s Tokenizer
Falcon 40B was pre-trained on RefinedWeb, so it expects a specific tokenization format. Here’s how you tokenize text properly:
from transformers import AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("tiiuae/falcon-40b")
example_text = "Fine-tuning Falcon 40B is efficient with PEFT."
tokens = tokenizer(example_text, return_tensors="pt")
print(tokens)
What’s happening here?
- We load Falcon’s tokenizer.
- We tokenize an example sentence.
- We return it as PyTorch tensors for training compatibility.
Pro tip: If you’re using LoRA or QLoRA, make sure tokenization aligns with the low-rank adaptation format.
Creating a Custom Dataset Class
For Hugging Face’s dataset format, I prefer wrapping raw text into a Dataset class. It keeps things clean and helps with batch processing later.
Here’s how I do it:
from datasets import Dataset
data = {"text": ["Your dataset sentence 1", "Your dataset sentence 2"]}
dataset = Dataset.from_dict(data)
print(dataset)
This lets you easily pass your data into a DataLoader for fine-tuning later.
Final Thoughts
I can’t stress this enough—a well-prepared dataset saves you hours of debugging later. I’ve seen people jump straight into training, only to realize their model is learning noise.
Take your time to clean, format, and tokenize your dataset properly, and you’ll set yourself up for a smooth fine-tuning experience.
4. Fine-Tuning Strategies
“Not everything that can be fine-tuned should be fine-tuned.”
When I first attempted to fine-tune Falcon 40B, I naively thought, “Why not just train the whole thing?” Then I saw my GPU utilization skyrocket, my VRAM instantly max out, and my system grind to a halt. Lesson learned.
Fine-tuning a 40B parameter model isn’t the same as tweaking a 1B model. It demands insane compute power, and unless you’re running an AI lab with unlimited budget, full fine-tuning is off the table.
So, what’s the smart way? Parameter-Efficient Fine-Tuning (PEFT)—a game-changer for training massive models without breaking the bank.
Let’s go over both approaches, and I’ll show you why full fine-tuning is impractical and why PEFT is the way to go.
4.1 Full Fine-Tuning (Not Recommended Due to Compute Costs)
Let me save you some time: Unless you have 400GB+ VRAM, forget about full fine-tuning. Here’s why:
- Compute Nightmare: You’ll need 8x A100 (80GB) GPUs just to hold the model in FP32.
- VRAM Explosion: A single forward + backward pass can consume 300GB+ VRAM.
- Slow & Expensive: Even with high-end hardware, expect days to weeks of training time.
Here’s an estimate of GPU memory usage for Falcon 40B fine-tuning:
Precision | Full Fine-Tuning VRAM Requirement |
---|---|
FP32 | ~400GB |
FP16 | ~200GB |
8-bit (with QLoRA) | ~40GB |
DeepSpeed ZeRO 3: A Workaround (Still Costly)
The only way to make full fine-tuning slightly feasible is by sharding the model across multiple GPUs using DeepSpeed ZeRO 3.
from transformers import TrainingArguments
from deepspeed.ops.adam import DeepSpeedCPUAdam
training_args = TrainingArguments(
per_device_train_batch_size=1, # Keep batch size low
gradient_accumulation_steps=8,
fp16=True, # Enable mixed precision
deepspeed="configs/deepspeed_zero3.json", # ZeRO 3 config
)
print("DeepSpeed ZeRO 3 Enabled!")
Does this help? Yes.
Is it still impractical? Absolutely.
For most use cases, full fine-tuning simply isn’t worth the cost. Let’s move on to the smarter approach.
4.2 Parameter-Efficient Fine-Tuning (PEFT)
This is where things get really interesting. Instead of retraining all 40B parameters, PEFT fine-tunes only a tiny subset, making it lightweight and scalable.
The two best techniques for Falcon 40B:
- LoRA (Low-Rank Adaptation) – For general fine-tuning with ~80GB VRAM
- QLoRA (Quantized LoRA) – For extreme memory efficiency, ~40GB VRAM
LoRA vs. QLoRA: Which One Should You Use?
Method | VRAM Requirement | Performance | Best For |
---|---|---|---|
LoRA | ~80GB | High | General NLP fine-tuning |
QLoRA | ~40GB | Medium | Low-resource fine-tuning |
Personally, I use LoRA when I have decent GPU availability and QLoRA when I’m tight on resources.
Implementing LoRA-Based Fine-Tuning
Here’s a practical LoRA implementation for Falcon 40B:
from peft import LoraConfig, get_peft_model
from transformers import AutoModelForCausalLM
# Load base Falcon model
base_model = AutoModelForCausalLM.from_pretrained("tiiuae/falcon-40b", device_map="auto")
# Configure LoRA for Falcon 40B
lora_config = LoraConfig(
r=16, # Low-rank dimension
lora_alpha=32, # Scaling factor
target_modules=["query_key_value"], # Target transformer blocks
lora_dropout=0.05,
bias="none",
task_type="CAUSAL_LM" # Causal Language Modeling
)
# Apply LoRA to the model
model = get_peft_model(base_model, lora_config)
model.print_trainable_parameters()
What’s Happening Here?
- We load Falcon 40B with automatic device mapping.
- We define LoRA hyperparameters (adjustable based on your hardware).
- We apply LoRA to specific transformer layers (query-key-value blocks).
- We print the number of trainable parameters (only a tiny fraction of 40B!).
Final Thoughts
PEFT is the way to go. LoRA & QLoRA make fine-tuning Falcon 40B actually possible on real-world hardware.
- My advice:
- If you have ~80GB VRAM, go with LoRA.
- If you have ~40GB VRAM, go with QLoRA.
- If you need full fine-tuning, make sure you’re prepared for the cost and complexity.
Next up: Training Falcon 40B efficiently with LoRA & DeepSpeed!
5. Training the Model
“Training a large-scale model isn’t about raw power—it’s about knowing how to make the most of what you have.”
I’ve run into out-of-memory (OOM) errors more times than I can count while training LLMs. The first time I tried training Falcon 40B, my setup froze within minutes. Lesson learned: Throwing hardware at the problem isn’t always the answer.
- Instead, you need a well-optimized training loop that leverages:
- DeepSpeed for memory efficiency
- LoRA to train only essential parameters
- Gradient accumulation to bypass batch size limits
- Mixed precision (FP16/8-bit) for performance gains
Here’s how you can train Falcon 40B without burning through your compute budget.
5.1 Training Loop Implementation
- A good training loop should:
- Prevent OOM errors (so your GPU doesn’t crash mid-training)
- Optimize memory usage (DeepSpeed + 8-bit AdamW)
- Speed up convergence (gradient accumulation + mixed precision)
Let’s break it down.
Step 1: Optimizing Training Arguments
DeepSpeed and LoRA are a powerful duo—they let you fine-tune without needing insane amounts of VRAM.
Here’s how I configure TrainingArguments for Falcon 40B fine-tuning:
from transformers import TrainingArguments, Trainer
training_args = TrainingArguments(
per_device_train_batch_size=2, # Keep batch size small
gradient_accumulation_steps=16, # Helps avoid OOM errors
optim="adamw_bnb_8bit", # 8-bit optimizer for lower VRAM usage
fp16=True, # Mixed precision for efficiency
evaluation_strategy="steps", # Periodic evaluation
save_steps=500, # Save model every 500 steps
output_dir="./falcon-finetuned", # Output directory
logging_dir="./logs", # Log training metrics
logging_steps=10, # Log progress frequently
report_to="wandb", # Use Weights & Biases for tracking
)
print("Training arguments initialized successfully!")
What’s happening here?
- Batch Size: 2 per GPU (helps prevent OOM errors)
- Gradient Accumulation: 16 steps (tricks the model into thinking we have a larger batch size)
- Optimizer: 8-bit AdamW (Reduces memory usage significantly)
- FP16 Training: Enables faster and more efficient computation
Step 2: Initializing the Trainer
Now, let’s put it all together using Hugging Face’s Trainer API:
trainer = Trainer(
model=model, # Our LoRA-optimized Falcon model
args=training_args,
train_dataset=dataset,
eval_dataset=val_dataset, # Optional: Include validation dataset
)
trainer.train()
🔹 Why Trainer?
- Handles automatic checkpointing
- Supports distributed training
- Manages gradient accumulation & mixed precision
🔹 Avoiding OOM Errors:
If you still hit CUDA OOM issues, reduce the batch size further or enable DeepSpeed ZeRO 3 for aggressive memory optimization:
training_args = TrainingArguments(
deepspeed="configs/deepspeed_zero3.json", # Enable ZeRO-3
per_device_train_batch_size=1,
gradient_accumulation_steps=32,
fp16=True,
)
Step 3: Monitoring Training Progress
“You can’t optimize what you don’t measure.”
I always use Weights & Biases (WandB) to monitor loss, accuracy, and GPU utilization.
import wandb
wandb.init(project="falcon-40b-finetuning", config=training_args)
Why use WandB?
- Logs loss curves in real-time
- Tracks GPU utilization
- Helps compare multiple training runs
6. Evaluation & Metrics
“A model is only as good as its weakest output.”
I’ve seen models that seemed promising during training but fell apart in real-world tests. That’s why evaluation is non-negotiable—you don’t just look at numbers, you validate performance qualitatively and quantitatively.
Let’s break it down.
6.1 Evaluating Fine-Tuned Falcon 40B
There are two ways to assess your fine-tuned model:
- Quantitative evaluation: Perplexity, BLEU, ROUGE
- Qualitative evaluation: Manual testing with diverse prompts
Step 1: Calculating Perplexity (PPL)
Why does PPL matter?
Perplexity tells us how well the model predicts the next token. Lower is better—a low PPL means the model generates fluent, predictable text.
Here’s how I calculate PPL for Falcon 40B:
import torch
from transformers import AutoModelForCausalLM, AutoTokenizer
# Load model & tokenizer
model = AutoModelForCausalLM.from_pretrained("tiiuae/falcon-40b").cuda()
tokenizer = AutoTokenizer.from_pretrained("tiiuae/falcon-40b")
model.eval()
# Sample input
input_text = "The future of AI is"
input_ids = tokenizer(input_text, return_tensors="pt").input_ids.cuda()
# Compute loss
with torch.no_grad():
outputs = model(input_ids, labels=input_ids)
perplexity = torch.exp(outputs.loss)
print("Perplexity:", perplexity.item())
🔹 What’s happening here?
- We compute loss on a sample input
- Exponentiating the loss gives us perplexity
Pro Tip:
If PPL is too high, your model might be overfitting. Try adding more diverse training data or regularizing with dropout.
Step 2: Evaluating with BLEU & ROUGE
I’ve found BLEU and ROUGE scores helpful for evaluating text coherence. These metrics compare generated outputs to reference responses.
Install evaluate
and run:
from evaluate import load
bleu = load("bleu")
rouge = load("rouge")
reference = ["The AI revolution is transforming industries."]
generated = ["The AI revolution is changing businesses."]
# Compute BLEU & ROUGE
bleu_score = bleu.compute(predictions=[generated], references=[reference])
rouge_score = rouge.compute(predictions=[generated], references=[reference])
print("BLEU:", bleu_score["bleu"])
print("ROUGE:", rouge_score)
Step 3: The Real Test—GPT-4 Evaluation
“If your model can’t pass a blind test against GPT-4, it’s not ready.”
GPT-based evaluation is becoming the gold standard. Here’s how I use GPT-4 to rank my model’s responses:
from openai import OpenAI
client = OpenAI(api_key="your-api-key")
response = client.chat.completions.create(
model="gpt-4",
messages=[
{"role": "system", "content": "Evaluate the response quality."},
{"role": "user", "content": "The AI revolution is transforming industries."},
{"role": "assistant", "content": "The AI revolution is changing businesses."}
]
)
print(response["choices"][0]["message"]["content"])
Why use GPT-4?
- Can grade responses on fluency, accuracy, and coherence
- More reliable than BLEU/ROUGE alone
7. Deployment: Running Inference Efficiently
“A model that can’t serve real users is just a research project.”
Once your Falcon 40B model is fine-tuned, you need to deploy it efficiently. Otherwise, inference will be painfully slow.
7.1 Quantization for Faster Inference
Falcon 40B is massive. You can cut inference costs by 50-75% using 4-bit or 8-bit quantization with bitsandbytes
.
Here’s how:
from transformers import AutoModelForCausalLM, AutoTokenizer, BitsAndBytesConfig
# Load quantized model
bnb_config = BitsAndBytesConfig(load_in_8bit=True)
model = AutoModelForCausalLM.from_pretrained("tiiuae/falcon-40b", quantization_config=bnb_config).cuda()
tokenizer = AutoTokenizer.from_pretrained("tiiuae/falcon-40b")
# Run inference
pipe = pipeline("text-generation", model=model, tokenizer=tokenizer)
result = pipe("What is the capital of France?", max_new_tokens=50)
print(result)
🔹 Why quantization?
- 8-bit cuts memory usage in half
- 4-bit is even more efficient, but sometimes reduces accuracy
Pro Tip:
For minimal performance loss, use QLoRA (Quantized LoRA) instead of direct 4-bit quantization.
7.2 Hosting on a Server (FastAPI)
If you want real-time inference, FastAPI + TGI (Text Generation Inference) is the best way to serve your model efficiently.
Step 1: Install dependencies
pip install fastapi uvicorn
Step 2: Deploy Falcon 40B with FastAPI
from fastapi import FastAPI
from transformers import pipeline
app = FastAPI()
# Load model
pipe = pipeline("text-generation", model="tiiuae/falcon-40b")
@app.post("/generate/")
async def generate_text(prompt: str):
result = pipe(prompt, max_new_tokens=50)
return {"response": result[0]["generated_text"]}
# Run API
if __name__ == "__main__":
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
- 🔹 Why FastAPI?
- Lightweight & fast
- Auto-generates API docs
- Handles multiple requests efficiently
🔹 Why use TGI instead of raw inference?
TGI optimizes inference by:
- Serving multiple requests in parallel
- Using flash attention for speed-ups
- Enabling efficient batching
To deploy on TGI, just run:
docker run -p 8080:80 ghcr.io/huggingface/text-generation-inference:latest
Conclusion: Mastering Falcon 40B Fine-Tuning & Deployment
“Every great AI model is just one fine-tune away from unlocking its full potential.”
We’ve covered everything—from fine-tuning Falcon 40B efficiently to deploying it for real-world use. Let’s take a moment to recap what you’ve learned and what comes next.
Key Takeaways
- Full fine-tuning is impractical for 40B+ models—PEFT (LoRA & QLoRA) is the way to go.
- Training efficiently requires DeepSpeed, gradient accumulation, and mixed precision.
- Evaluation isn’t just about numbers—use PPL, BLEU, ROUGE, and even GPT-4 to validate your model.
- Quantization (4-bit & 8-bit) can cut inference costs without sacrificing much performance.
- FastAPI + TGI makes Falcon 40B deployable at scale, ensuring fast and reliable real-time inference.
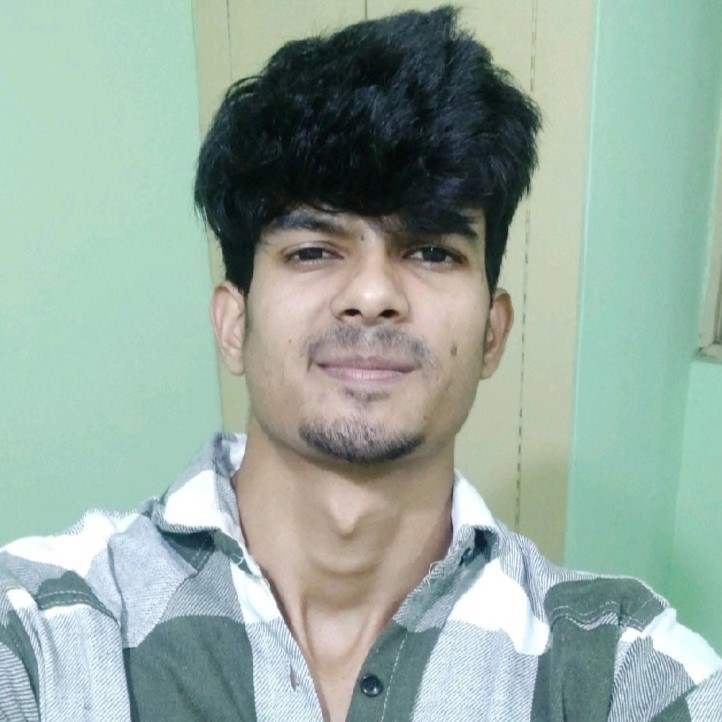
I’m a Data Scientist.