1. Introduction: Why Fine-Tune DinoV2 At All?
I’ll be honest—fine-tuning DinoV2 isn’t something I reach for every day.
But when I’m dealing with data that’s far from ImageNet—think industrial defect images, medical scans, or satellite captures—it starts to make a lot of sense.
DinoV2 already gives you strong representations, but out-of-the-box features don’t always cut it in specialized domains.
If you’re here, I’m assuming you already know what DinoV2 is and why it’s different from traditional supervised vision models.
So we’re skipping the theory and diving straight into the real stuff—what worked for me, what didn’t, and what you’ll want to avoid.
2. Prepping Your Environment (Minimal but Critical Setup)
Let’s not waste time with pip installs and Python 3.10 reminders—you’ve done this before.
What is worth calling out are the edge cases I ran into when setting up DinoV2 for fine-tuning, especially with larger variants like vit-large
and vit-gigantic
.
Here’s what mattered for me:
CUDA & PyTorch Compatibility
You will want CUDA 11.8+ if you’re planning to use mixed precision. I used PyTorch 2.1.0 with torchvision==0.16.0
, and that combo worked smoothly with DINO-style transforms. If you’re still on 1.x, you’ll likely hit performance bottlenecks.
Also—make sure your environment has torch.cuda.amp
properly enabled. I’ve seen folks miss out on this and wonder why their memory usage is through the roof.
bfloat16 vs FP16
This might surprise you: FP16 isn’t always faster on newer NVIDIA cards. I personally got better stability on A100s using bfloat16 through transformers
‘s mixed precision setup. For other cards like 3090/4090, FP16 with torch.cuda.amp
worked just fine.
Repo Setup
I started with Meta’s official DINOv2 repo, but made some changes to get it working with my fine-tuning setup:
- Integrated
bitsandbytes
to save memory for large models. - Swapped out their dataloader pipeline with mine to support LMDB datasets.
- Added support for classification heads on top of the frozen ViT backbone.
If you’re using HuggingFace’s version via transformers
, you’ll have to wrap your head around how their feature extractor interacts with torchvision transforms—it’s not a plug-and-play swap.
Reproducible Setup
Here’s a sample conda.yaml
I used for a clean environment:
name: dinov2-finetune
channels:
- pytorch
- conda-forge
dependencies:
- python=3.10
- pytorch=2.1.0
- torchvision=0.16.0
- cudatoolkit=11.8
- bitsandbytes
- numpy
- scikit-learn
- matplotlib
- pip
- pip:
- wandb
- albumentations
- timm
And if you’re using torchrun
or deepspeed
, make sure to explicitly set --bf16
or --amp
flags depending on your setup. I’ll get into training configs in more detail later.
3. Dataset Curation (What Actually Matters)
There’s a trap I’ve seen people fall into with DinoV2: assuming that any old image folder setup will work just fine. That’s not how it played out for me.
I’ve worked with both folder-based datasets and LMDB formats, and personally, LMDB gave me a serious boost in data loading speed—especially when training with large batch sizes on multiple GPUs. If you’re dealing with tens of thousands of high-res images, that I/O optimization matters.
Image Sizes and Backbone Quirks
This might catch you off guard: ViT-G (the largest DinoV2 model) was pretrained on 518×518 crops. Not the usual 224×224. So if you’re fine-tuning vit-g
, and you’re still using 224×224 images—you’re bottlenecking its performance.
For vit-b
and vit-l
, I got away with 224×224 just fine, but for ViT-G, I had to bump my training crops to 518×518 to really see gains. You can interpolate weights down to smaller sizes, but in my runs, that introduced artifacts and slower convergence.
Data Augmentations That Actually Helped
I’ve tried the standard RandomResizedCrop + HorizontalFlip
combo, but honestly? When I was working on a fine-grained classification task (industrial surface defects), that didn’t cut it. Too much information was getting lost.
Here’s a train/val transform setup that actually worked for me:
from torchvision import transforms
train_transform = transforms.Compose([
transforms.RandomResizedCrop(518, scale=(0.8, 1.0)),
transforms.RandomHorizontalFlip(p=0.5),
transforms.RandomApply([
transforms.ColorJitter(brightness=0.2, contrast=0.2, saturation=0.2, hue=0.05)
], p=0.3),
transforms.RandomGrayscale(p=0.1),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225])
])
val_transform = transforms.Compose([
transforms.Resize(550),
transforms.CenterCrop(518),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225])
])
I’ve used these with PyTorch ImageFolder
, and here’s the dataset wrapper I use in most of my runs:
from torchvision.datasets import ImageFolder
from torch.utils.data import DataLoader
train_dataset = ImageFolder('/path/to/train', transform=train_transform)
val_dataset = ImageFolder('/path/to/val', transform=val_transform)
train_loader = DataLoader(train_dataset, batch_size=64, shuffle=True, num_workers=8, pin_memory=True)
val_loader = DataLoader(val_dataset, batch_size=64, shuffle=False, num_workers=8, pin_memory=True)
If you’re working with LMDB, you’ll need to build a custom Dataset class—but honestly, the transform logic above still holds.
4. Model Loading: DinoV2 Backbone + Projection Head
Let me be blunt: loading DinoV2 isn’t just a few lines of from_pretrained()
. If you’re serious about fine-tuning, especially for classification or contrastive tasks, you’ll likely need to inject a custom head. I’ve built mine from scratch for flexibility, and I’ll show you how.
Which Model? And Why?
For my fine-tuning runs, I mostly used vit-b
and vit-l
. vit-g
was tempting, but even on A100s, the memory demands were brutal—especially if you’re using batch sizes >32 and higher image resolutions.
vit-b
gave me the best cost-performance ratio. With vit-l
, I started to see sharper performance on fine-grained tasks, but only when paired with larger images and longer training runs. If you’re constrained on compute, vit-b
is the sweet spot.
Loading Pretrained DinoV2 Backbones
You can get the pretrained weights either via HuggingFace or Meta’s official repo. I personally cloned the Meta repo, and here’s how I initialized the model:
import torch
from dinov2.models.vision_transformer import vit_base
def load_dinov2_backbone():
model = vit_base(pretrained=True) # or vit_large, vit_giant
return model
Keep in mind: DinoV2 outputs a CLS token embedding, not logits. That’s where your head comes in.
Adding a Custom Classification Head
Here’s the deal: I’ve experimented with simple linear heads and deeper MLPs. For most classification tasks, a two-layer MLP with dropout worked best—especially when I was fine-tuning only the head.
import torch.nn as nn
class DinoClassifier(nn.Module):
def __init__(self, backbone, num_classes):
super().__init__()
self.backbone = backbone
self.head = nn.Sequential(
nn.Linear(backbone.embed_dim, 512),
nn.ReLU(),
nn.Dropout(0.3),
nn.Linear(512, num_classes)
)
def forward(self, x):
x = self.backbone.get_intermediate_layers(x, n=1)[0][:, 0] # CLS token
return self.head(x)
This design gave me a good balance between capacity and generalization. You might be tempted to go deeper, but in my experience, it didn’t help unless I had a lot of labeled data.
Freezing Smartly (Not Blindly)
Freezing the entire backbone might seem like a safe default—but I’ve found better results by unfreezing just the last N transformer blocks. On vit-b
, unfreezing the last 2 blocks (layers 10 and 11) gave me a nice performance bump without adding too much instability.
You can do something like this:
for name, param in model.backbone.named_parameters():
if 'blocks.10' in name or 'blocks.11' in name:
param.requires_grad = True
else:
param.requires_grad = False
5. Fine-Tuning Strategy: What Actually Works
Tuning DinoV2 isn’t about just slapping an optimizer on and hoping for the best. I’ve had fine-tuning runs tank just from bad scheduler configs or underestimating memory pressure. So here’s what I’ve learned—first-hand.
a) Optimizer & LR Schedule
You might be wondering: Is AdamW still the go-to?
In my experience, AdamW has been the most reliable optimizer across the board—especially when fine-tuning just the head or partial layers. I experimented with Lion (from Google) and SGD+Momentum, but unless I had a huge batch size (which isn’t always practical with DinoV2-L or G), AdamW just converged faster and more smoothly.
For LR scheduling, cosine decay with a linear warmup gave me the best training curves. No surprises there, but the key was tuning the warmup steps just right.
Here’s the config I used for a DinoV2-B run:
import torch
from torch.optim import AdamW
from transformers import get_cosine_schedule_with_warmup
optimizer = AdamW(model.parameters(), lr=3e-5, weight_decay=0.05)
num_training_steps = len(train_loader) * num_epochs
num_warmup_steps = int(0.1 * num_training_steps) # 10% warmup
lr_scheduler = get_cosine_schedule_with_warmup(
optimizer,
num_warmup_steps=num_warmup_steps,
num_training_steps=num_training_steps
)
What made the difference? Keeping the learning rate low unless I was unfreezing more than two layers. For full fine-tuning on ViT-G, I had to bump it up and increase warmup to ~15% of steps.
b) Mixed Precision & Gradient Accumulation
Here’s the deal: if you’re training DinoV2-G and you’re not using AMP or something like DeepSpeed, you’re either wasting compute or about to run into an OOM wall.
Personally, I’ve had great success using torch.cuda.amp
for mixed precision. It gave me a ~35% memory reduction, which meant I could bump batch size and stabilize training without blowing past 40GB on an A100.
On one run with vit-l
, I combined AMP with gradient accumulation to simulate a batch size of 128 on just 4 A100s:
scaler = torch.cuda.amp.GradScaler()
for epoch in range(num_epochs):
model.train()
for step, (images, labels) in enumerate(train_loader):
images, labels = images.cuda(), labels.cuda()
with torch.cuda.amp.autocast():
outputs = model(images)
loss = criterion(outputs, labels)
scaler.scale(loss).backward()
if (step + 1) % accumulation_steps == 0:
scaler.step(optimizer)
scaler.update()
optimizer.zero_grad()
lr_scheduler.step()
If you’re running into OOM on ViT-G even with AMP, bitsandbytes can help — but I found it’s more useful during inference. For training, AMP + grad accumulation is cleaner and more stable (especially for logging/debugging).
c) Selective Layer Unfreezing
Here’s something I learned the hard way: unfreezing the entire backbone right from epoch 1? Total instability. Loss spikes, gradients explode, accuracy flatlines.
So I started progressive unfreezing—and it made a huge difference in both stability and final accuracy. For ViT-B and ViT-L, I unfroze 2 layers every 2 epochs, starting from the head and moving backward.
Here’s how I managed it:
def unfreeze_layers(model, n_layers):
for i in range(11, 11 - n_layers, -1): # Assuming 12 layers total
for name, param in model.backbone.blocks[i].named_parameters():
param.requires_grad = True
Then in the training loop:
if epoch in [2, 4, 6]:
layers_to_unfreeze = (epoch // 2)
unfreeze_layers(model, layers_to_unfreeze)
print(f"Unfroze last {layers_to_unfreeze} transformer blocks")
This strategy gave me better generalization and fewer issues with catastrophic forgetting. If you’re working on a dataset that’s even slightly different from DinoV2’s pretraining domain, progressive unfreezing is a must.
Alright, that covers the full fine-tuning strategy—from optimizer to precision hacks to layer management. Next up, I’ll walk you through logging, evaluation, and the one weird bug that nearly ruined one of my best checkpoints.
6. Training Loop (With Monitoring)
You’ve probably seen a hundred training loops before. But here’s the thing: when fine-tuning DinoV2, especially the larger variants, even small missteps in loop structure can blow up memory or slow training to a crawl.
Here’s how I personally structure it — clean, modular, and memory-aware.
What’s included:
- AMP with proper scaling
- Accurate metric tracking (Top-1, Top-5)
- Logging via
wandb
(but you can sub in TensorBoard if that’s your thing)
import torch
import wandb
from torch.cuda.amp import autocast, GradScaler
scaler = GradScaler()
wandb.init(project="dino-finetune", config={"epochs": num_epochs})
for epoch in range(num_epochs):
model.train()
running_loss, correct1, correct5 = 0.0, 0, 0
total = 0
for i, (images, labels) in enumerate(train_loader):
images, labels = images.cuda(), labels.cuda()
optimizer.zero_grad()
with autocast():
outputs = model(images)
loss = criterion(outputs, labels)
scaler.scale(loss).backward()
scaler.step(optimizer)
scaler.update()
lr_scheduler.step()
# Top-1 / Top-5 accuracy
_, pred = outputs.topk(5, 1, True, True)
correct = pred.eq(labels.view(-1, 1).expand_as(pred))
correct1 += correct[:, 0].sum().item()
correct5 += correct.sum().item()
total += labels.size(0)
running_loss += loss.item()
if i % 10 == 0:
wandb.log({
"loss": loss.item(),
"top1_acc": correct1 / total,
"top5_acc": correct5 / total,
"lr": lr_scheduler.get_last_lr()[0]
})
print(f"Epoch {epoch+1} | Loss: {running_loss/len(train_loader):.4f} | Top1: {correct1/total:.4f} | Top5: {correct5/total:.4f}")
Why wandb?
Because when you’re unfreezing layers mid-training or debugging a flaky LR schedule, visualizing those transitions is gold. I could immediately spot when training derailed after unfreezing layers 7–12.
If you’re not using wandb, log these to console at minimum — you’ll thank yourself later when troubleshooting checkpoint inconsistencies.
7. Evaluation & Validation
This might surprise you: accuracy isn’t always the best metric — especially not Top-1. I learned this the hard way on a multi-label medical imaging task. DinoV2 looked like it was performing well, but real-world validation told a different story.
Here’s how I approach evaluation post-fine-tuning:
Checklist:
- Top-1, Top-5 (yes, still useful for general benchmarks)
- F1-score / ROC-AUC if the dataset demands it
- Confusion Matrix for class imbalance sanity check
- Visual Explanations (Grad-CAM) — especially if you’re presenting results to a stakeholder or domain expert
Example: Evaluation Script
from sklearn.metrics import accuracy_score, f1_score, confusion_matrix
import seaborn as sns
import matplotlib.pyplot as plt
model.eval()
all_preds, all_labels = [], []
with torch.no_grad():
for images, labels in val_loader:
images = images.cuda()
outputs = model(images)
preds = outputs.argmax(dim=1).cpu()
all_preds.extend(preds)
all_labels.extend(labels)
# Metrics
acc = accuracy_score(all_labels, all_preds)
f1 = f1_score(all_labels, all_preds, average='weighted')
cm = confusion_matrix(all_labels, all_preds)
print(f"Val Accuracy: {acc:.4f}, F1 Score: {f1:.4f}")
# Confusion Matrix
plt.figure(figsize=(10,8))
sns.heatmap(cm, annot=True, fmt="d", cmap="Blues")
plt.title("Confusion Matrix")
plt.xlabel("Predicted")
plt.ylabel("True")
plt.tight_layout()
plt.savefig("conf_matrix.png")
Example: Grad-CAM (for interpretability)
If you’re working in medical, industrial, or surveillance domains — Grad-CAM isn’t optional. Here’s how I integrated it:
from pytorch_grad_cam import GradCAM
from pytorch_grad_cam.utils.image import show_cam_on_image
target_layer = model.backbone.blocks[-1].norm1
cam = GradCAM(model=model, target_layers=[target_layer], use_cuda=True)
grayscale_cam = cam(input_tensor=images, targets=None)[0, :]
# Overlay
visualization = show_cam_on_image(images[0].permute(1, 2, 0).cpu().numpy(), grayscale_cam, use_rgb=True)
plt.imshow(visualization)
plt.axis('off')
plt.savefig("gradcam_result.png")
One last note from experience:
Don’t just rely on metrics from val_loader
. I always reserve a completely untouched, real-world test set — one that mimics the actual deployment scenario. That’s where I’ve seen models fail in ways that Top-1 accuracy couldn’t predict.
8. Inference Pipeline (Production-Ready Version)
“Slow models are broken models.” I’ve had to live by this, especially when deploying DinoV2 into environments where inference latency actually matters — think edge devices and GPU-constrained APIs.
You might be wondering:
“How do you squeeze the most out of DinoV2 without cutting corners on accuracy?”
Here’s the strategy I landed on.
Key Components:
- Batched inference with
torch.compile()
(if supported by your stack) - TorchScript fallback (more portable than ONNX in my case)
- Half-precision inference where possible (massive speedup on A100s and even consumer GPUs)
Inference Class (with TorchScript Option)
import torch
from torchvision import transforms
from PIL import Image
class DinoV2Inference:
def __init__(self, model_path, device='cuda'):
self.device = device
self.model = torch.jit.load(model_path).to(device).eval()
self.transform = transforms.Compose([
transforms.Resize((518, 518)), # match DinoV2-ViT-G input
transforms.ToTensor(),
transforms.Normalize(mean=[0.5]*3, std=[0.5]*3)
])
def predict(self, image: Image.Image):
input_tensor = self.transform(image).unsqueeze(0).to(self.device)
with torch.no_grad(), torch.cuda.amp.autocast():
output = self.model(input_tensor)
probs = torch.softmax(output, dim=1)
return probs.cpu().squeeze()
TorchScript Export
This is what I used for deployment — TorchScript gave me fewer headaches than ONNX, especially with custom heads.
scripted_model = torch.jit.script(model.cpu())
scripted_model.save("dino_v2_scripted.pt")
If you’re going the ONNX route, watch out for custom layers or torch.nn.functional
ops that don’t export cleanly. I hit that wall more than once.
Bonus: torch.compile for Runtime Speed
If you’re running PyTorch 2.x and don’t need export, this is the fastest I’ve seen DinoV2 run:
compiled_model = torch.compile(model)
But be careful: torch.compile()
doesn’t always play well with mixed precision or scripting/export. I used it only in internal tools, not production deployment.
9. Gotchas & Lessons Learned
Let’s be real — not everything goes smoothly. And while most guides paint a clean picture, this is the part where I talk to you, my past self, and try to save you a week of debugging.
Gotcha #1: Image Size Mismatch Wrecks Everything
DinoV2-ViT-G expects 518×518 images. Feed it 224×224 out of habit and you’ll wonder why performance nosedives.
Even resizing to 512×512 gave me slightly degraded results. Be precise — 518×518 isn’t a suggestion, it’s a requirement.
Gotcha #2: Frozen ≠ Stable
This might surprise you:
Even when I froze the backbone, the model still overfit on smaller datasets. The culprit? The projection head had too much capacity and no dropout.
I had to explicitly add dropout + weight decay, even though I initially assumed it was “just a head.”
Gotcha #3: torchvision Transforms ≠ Dino Preprocessing
There’s a subtle but deadly mismatch between torchvision.transforms.Normalize()
and the actual preprocessing DinoV2 was trained with. I had to reverse-engineer the facebookresearch/dinov2
repo to align my data loader.
Lesson: don’t trust defaults — match the pretraining pipeline as closely as possible.
Gotcha #4: torch.compile Explodes Silently
When I first wrapped DinoV2 with torch.compile()
, the model ran… but produced junk predictions. No error, no warning. Turns out, one of my custom hooks wasn’t compatible with the compiler backend.
If you’re using torch.compile
, start simple, test outputs thoroughly, and add complexity in stages.
10. Final Thoughts
“Just because you can fine-tune a foundation model doesn’t mean you should.”
That’s something I had to learn the hard way with DinoV2. It’s a powerful model — no doubt. But it’s not always the right tool for the job as-is.
When Fine-Tuning DinoV2 Isn’t Worth It
If you’re working with:
- A small labeled dataset (few hundred to a few thousand images)
- A task where features are already linearly separable (e.g., classic object recognition)
- Limited compute (sub-16GB GPU or no distributed support)
…then full fine-tuning DinoV2 is likely overkill. You’ll end up overfitting, hitting memory walls, or wasting cycles optimizing a beast that doesn’t need retraining.
There were times I ran full fine-tunes only to realize the backbone features were already doing 95% of the job — I just hadn’t tried probing them properly.
When Linear Probing Is Enough
In a few of my earlier experiments, I ran DinoV2 as a frozen feature extractor and slapped on a linear classifier — think LogisticRegression(solver='lbfgs')
from scikit-learn
.
To my surprise, on datasets like CIFAR-100, Oxford Pets, and even some custom satellite image datasets, the linear head got me very close to full fine-tuning accuracy — and in a fraction of the time.
The trick is to use the right layer output from the model. For DinoV2, I had the best luck extracting from the last [CLS] token projection layer before the head.
Here’s a snippet from that approach:
with torch.no_grad():
features = model.forward_features(images) # assuming model exposes this
cls_tokens = features[:, 0] # [CLS] token
Once you have cls_tokens
, you can fit a linear classifier or SVM in seconds. And more importantly — you avoid touching a single model weight.
What I’d Do Differently Next Time
Looking back, there are a few things I’d change in my workflow:
- Start with probing before fine-tuning. Always. It sets a baseline and helps justify the cost of fine-tuning.
- Benchmark RAM and VRAM usage ahead of time. I wasted hours tuning batch sizes manually when I could’ve just profiled a forward pass at each resolution/model size.
- Stick to fewer augmentations. I over-engineered my transform pipeline early on, assuming it would “help generalization.” In practice, minimal augmentation (resize, crop, flip, normalize) gave cleaner and more predictable convergence.
- Freeze more layers early. Especially with DinoV2-G, freezing the first N transformer blocks for the first few epochs gave me more stable training and prevented noisy gradients from wrecking early learning.
So if you’re considering fine-tuning DinoV2, here’s my advice:
Don’t start with fine-tuning. Start with probing. Let the model prove it needs more training — and not the other way around.
That’s it. If you’ve made it this far, you probably already know your way around a few hundred thousand parameters. My hope is that this guide saved you from a few landmines I stepped on myself.
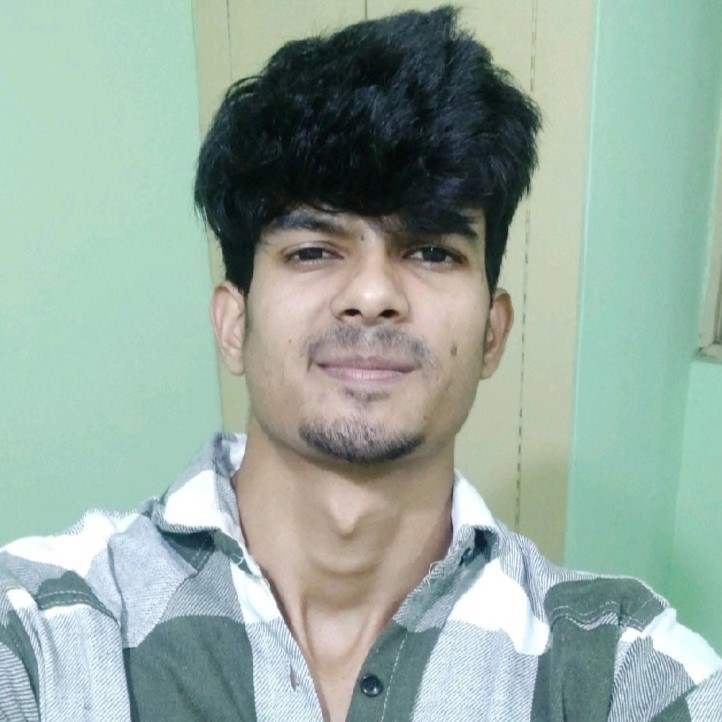
I’m a Data Scientist.