1. Introduction
Fine-tuning BERT for Question Answering isn’t new—but doing it right, especially in production setups or latency-sensitive environments, still takes a bit of finesse.
I’ve gone down the rabbit hole of fine-tuning BERT across multiple QA datasets—SQuAD, Natural Questions, even some messy internal corpora—and after all the trial and error, I’ve settled on a process that actually works. Efficiently. Reliably.
In this guide, I’m sharing the exact steps I follow—from loading and preprocessing your dataset, to fine-tuning, evaluation, and inference.
I’ll keep it tight and focused. You’ll get runnable code, hard-earned tips, and techniques to debug edge cases—like overlapping contexts or incorrect answer spans—without wasting compute cycles or GPU time.
Whether you’re working with SQuAD-style data or your own internal QA dataset, this is everything I wish someone had shown me earlier.
2. When to Fine-Tune BERT for QA (and When Not To)
Let’s be honest—BERT isn’t shiny anymore. You’ve probably heard folks say things like “Just use DeBERTa or jump to RAG”. And sure, if you’re swimming in GPU credits and want state-of-the-art scores, those models are tempting.
But here’s the deal: I still find BERT incredibly practical for many real-world QA tasks. Especially when:
- You’re deploying to CPU or edge devices
- Latency matters more than leaderboard scores
- You’re working with moderately sized documents (under 512 tokens)
- You want fast iteration and easier debugging
Personally, I’ve used bert-base-uncased
in production systems where startup latency was a concern, and even distilbert-base-uncased
for lightweight mobile inference.
In these setups, fine-tuning BERT gave me all the control I needed—without pulling in half the HuggingFace zoo.
That said, there are times I skip BERT entirely. If I’m dealing with long-form documents (e.g., legal or academic texts), I jump to Longformer or a retrieval-augmented setup.
Same goes for multi-hop QA—BERT just isn’t built for reasoning across disconnected passages.
So, before diving in, ask yourself this: Do you really need DeBERTa-level power? Or will a well-tuned BERT get the job done?
3. Dataset Setup: Choosing and Preparing QA Data
If you’re using prebuilt datasets like SQuAD v2, HuggingFace makes the process dead simple. But the moment you switch to custom data—maybe scraped FAQs, customer service logs, or annotated docs—things get messy fast. I’ve had my fair share of “why is this sample throwing an index error?” moments. So here’s how I handle it.
3.1 Supported Formats
When using HuggingFace’s AutoModelForQuestionAnswering
, your data needs to follow a SQuAD-like format. For reference, here’s the general structure:
{
"data": [
{
"title": "Example",
"paragraphs": [
{
"context": "Text that contains the answer.",
"qas": [
{
"id": "unique-id",
"question": "Your question goes here?",
"answers": [
{
"text": "the answer text",
"answer_start": 23
}
],
"is_impossible": false // SQuAD v2 only
}
]
}
]
}
]
}
Quick gotchas from experience:
- If your
answer_start
index is even a few characters off, the model will silently learn garbage. - For long contexts, overlapping span creation later in preprocessing becomes essential—or you’ll miss answers completely.
- Some tools round-trim whitespace, which can desync
answer_start
. I always write a quick validator script to catch that before training.
3.2 Using HuggingFace Datasets
If you’re just validating your fine-tuning pipeline or comparing baselines, squad_v2
is perfect.
from datasets import load_dataset
dataset = load_dataset("squad_v2")
You’ll get the usual train/validation splits, and both answerable and unanswerable samples. I like using SQuAD v2 to benchmark initial model performance before pushing on internal data.
3.3 Optional: Converting Custom QA Data
For custom pipelines, here’s the function I’ve been using lately. It converts raw data—CSV, JSONL, whatever—into a format compatible with HuggingFace’s QA pipeline:
def convert_to_squad_format(data):
squad_data = {"data": []}
for entry in data:
context = entry["context"]
question = entry["question"]
answer_text = entry["answer"]
answer_start = context.find(answer_text)
if answer_start == -1:
continue # bad annotation
squad_entry = {
"title": "custom",
"paragraphs": [{
"context": context,
"qas": [{
"id": entry["id"],
"question": question,
"answers": [{
"text": answer_text,
"answer_start": answer_start
}],
"is_impossible": False
}]
}]
}
squad_data["data"].append(squad_entry)
return squad_data
This has saved me from manually tweaking hundreds of entries. You can dump the result to disk and load it with load_dataset('json', data_files=...)
.
4. Tokenization and Preprocessing
Here’s where things get surgical. If your preprocessing is even slightly off—wrong stride, poor truncation, bad overflow mapping—your model will learn the wrong associations. I’ve debugged broken QA models before, and more than once the root cause was in this step.
Tokenization Strategy
Use the tokenizer’s built-in sliding window technique with truncation='only_second'
and stride
. That way, long contexts get chunked properly, and answers at the boundaries don’t get cut out.
from transformers import AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased", use_fast=True)
Preparing Features
You’ll need to preprocess the dataset so the model sees tokenized input with start and end positions for each answer. This function handles it all—plus a few debug checks I personally rely on:
def prepare_train_features(examples):
tokenized_examples = tokenizer(
examples["question"],
examples["context"],
truncation="only_second",
max_length=384,
stride=128,
return_overflowing_tokens=True,
return_offsets_mapping=True,
padding="max_length",
)
sample_mapping = tokenized_examples.pop("overflow_to_sample_mapping")
offset_mapping = tokenized_examples.pop("offset_mapping")
start_positions = []
end_positions = []
for i, offsets in enumerate(offset_mapping):
input_ids = tokenized_examples["input_ids"][i]
cls_index = input_ids.index(tokenizer.cls_token_id)
sequence_ids = tokenized_examples.sequence_ids(i)
sample_index = sample_mapping[i]
answers = examples["answers"][sample_index]
if len(answers["answer_start"]) == 0:
start_positions.append(cls_index)
end_positions.append(cls_index)
continue
start_char = answers["answer_start"][0]
end_char = start_char + len(answers["text"][0])
token_start_index = 0
while sequence_ids[token_start_index] != 1:
token_start_index += 1
token_end_index = len(input_ids) - 1
while sequence_ids[token_end_index] != 1:
token_end_index -= 1
if not (offsets[token_start_index][0] <= start_char and offsets[token_end_index][1] >= end_char):
start_positions.append(cls_index)
end_positions.append(cls_index)
else:
for idx in range(token_start_index, token_end_index + 1):
if offsets[idx][0] <= start_char and offsets[idx][1] > start_char:
start_positions.append(idx)
if offsets[idx][0] < end_char and offsets[idx][1] >= end_char:
end_positions.append(idx)
break
tokenized_examples["start_positions"] = start_positions
tokenized_examples["end_positions"] = end_positions
return tokenized_examples
I always log a few examples after this step to confirm the token-to-text alignment is still correct. Saves hours of debugging later.
5. Fine-Tuning: Model Setup and Training Loop
There’s a difference between “getting BERT to run” and “fine-tuning it well enough to deploy.” This section is everything I’ve learned the hard way while optimizing BERT for real-world QA tasks—from choosing the right variant to structuring the training loop with reproducibility and monitoring baked in.
5.1 Choosing the Right BERT Variant
Here’s where most people get stuck spinning their wheels. There are way too many BERT variants floating around, and I’ve personally wasted time running expensive experiments that didn’t yield much return.
Let me save you the trouble:
Variant | When I Use It |
---|---|
bert-base-uncased | Solid default. Good for 95% of tasks unless your dataset is huge. |
bert-large-uncased-whole-word-masking | When I need a few extra points on EM/F1, especially with large training sets. |
distilbert-base-uncased | For fast CPU inference or when latency is king. |
If you’re dealing with custom or noisy data, I tend to stick with bert-base-uncased
—the training is quicker, and the performance is often good enough with proper preprocessing.
5.2 Model Setup
Here’s the barebones setup. No magic tricks—just clean, standard code:
from transformers import AutoModelForQuestionAnswering
model = AutoModelForQuestionAnswering.from_pretrained("bert-base-uncased")
You can swap in any variant you prefer. I usually freeze the first few layers during early experimentation, then unfreeze everything once I’m sure the pipeline’s solid.
5.3 Training Code (Full Example)
I’ve tried both Trainer
and custom PyTorch loops. Honestly, unless you need ultra-custom scheduling or loss tricks, HuggingFace’s Trainer
gets the job done—especially if you configure it properly.
Here’s what I typically run with:
from transformers import TrainingArguments, Trainer
training_args = TrainingArguments(
output_dir="./bert-qa",
evaluation_strategy="epoch",
learning_rate=3e-5,
per_device_train_batch_size=12,
per_device_eval_batch_size=12,
num_train_epochs=3,
weight_decay=0.01,
fp16=True, # if you're using GPU with mixed precision
save_total_limit=2,
load_best_model_at_end=True,
metric_for_best_model="f1",
logging_dir="./logs",
logging_strategy="steps",
logging_steps=50,
gradient_accumulation_steps=2,
seed=42
)
And the trainer itself:
trainer = Trainer(
model=model,
args=training_args,
train_dataset=tokenized_train,
eval_dataset=tokenized_val,
tokenizer=tokenizer,
compute_metrics=compute_metrics_fn, # Define this for EM/F1
)
Pro tips from my experience:
- FP16 gives you a solid speedup if your GPU supports it. Never train without it on recent hardware.
- Gradient accumulation helps when your GPU memory is tight. I’ve used it on 8GB cards to train BERT-Large without issues.
- Checkpointing is critical. I once lost the best model due to a power blip—
load_best_model_at_end=True
now lives in all my scripts. - Logging with either TensorBoard or
wandb
is not optional. You won’t know when the model’s overfitting unless you see the curves.
If you’re fine-tuning on noisy or small data, enable early stopping. HuggingFace doesn’t have it built-in directly in Trainer
, but you can implement a simple callback.
Want to go lower-level? I’ve also written custom training loops with manual control over gradient clipping, warmup schedules, and per-batch eval—let me know if you want that version too.
6. Evaluation: Computing EM and F1
You’d think evaluation is the easy part—until it isn’t.
In my experience, getting evaluation right with BERT QA models is just as critical as the fine-tuning itself. Especially when unanswerable questions are in the mix. I’ve been burned before by mismatched spans, wrong thresholds, and silent failures in post-processing.
Quick Setup: EM and F1 with evaluate
Here’s the setup I personally use for SQuAD v2-style evaluation:
from evaluate import load
metric = load("squad_v2")
results = metric.compute(predictions=preds, references=refs)
predictions
should be a list of dicts like:
[
{
"id": "56ddde6b9a695914005b9628",
"prediction_text": "Normans"
},
...
]
references
should match this structure:
[
{
"id": "56ddde6b9a695914005b9628",
"answers": {
"text": ["Normans", "The Normans"],
"answer_start": [0, 0] # not used in scoring but required
}
},
...
]
This gives you both Exact Match (EM) and F1, which are the two core metrics I always track. F1 tends to be more forgiving and informative, especially on fuzzy or paraphrased answers.
Handling Unanswerables (Trust Me: Don’t Skip This)
Here’s the part that tripped me up early on: predicting when not to answer.
BERT gives you logits for both start and end positions—but what it doesn’t give you is a clean way to say: “Hey, I don’t think the answer exists in this context.”
What I’ve found works well is using the score of the [CLS]
token (which usually maps to no-answer) and setting a threshold. If the best answer span’s score is lower than the [CLS]
score minus a margin, we skip that prediction.
Here’s the post-processing logic I’ve used:
def is_impossible_answer(start_logits, end_logits, cls_index, threshold=-1.0):
cls_score = start_logits[cls_index] + end_logits[cls_index]
best_non_cls_score = max(start_logits) + max(end_logits)
return (cls_score - best_non_cls_score) > threshold
I’ve manually tuned the threshold
using the validation set. For SQuAD v2, anything around -1.0
to -1.5
tends to work okay—but honestly, your mileage will vary depending on how noisy your data is.
7. Inference Pipeline: Clean, Fast, Deployable
Once you’ve fine-tuned your model and validated it, you’ll want a clean way to get predictions from it—without dragging in all the training boilerplate.
Here’s what I’ve been using as a minimal, production-ready inference function:
def get_answer(question, context, model, tokenizer, threshold=-1.0):
inputs = tokenizer.encode_plus(
question,
context,
return_tensors="pt",
truncation=True,
max_length=512
)
input_ids = inputs["input_ids"]
outputs = model(**inputs)
start_logits = outputs.start_logits[0].detach()
end_logits = outputs.end_logits[0].detach()
cls_index = input_ids[0].tolist().index(tokenizer.cls_token_id)
if is_impossible_answer(start_logits, end_logits, cls_index, threshold):
return "No Answer"
# Softmax scores (optional for confidence)
import torch
start_probs = torch.nn.functional.softmax(start_logits, dim=-1)
end_probs = torch.nn.functional.softmax(end_logits, dim=-1)
start_idx = torch.argmax(start_probs)
end_idx = torch.argmax(end_probs)
if start_idx > end_idx:
return "No Answer"
answer_ids = input_ids[0][start_idx:end_idx + 1]
return tokenizer.decode(answer_ids)
I’ve tested this setup in both batch jobs and live APIs. It’s lean enough for fast inference but includes all the essentials: truncation, thresholding, span extraction, and sanity checks.
Optional: Wrapping It in FastAPI or Gradio
If I’m demoing the model to stakeholders or testing it interactively, I sometimes wrap it with Gradio for a quick UI. This has helped me catch a few edge cases in real-world text.
import gradio as gr
iface = gr.Interface(fn=get_answer, inputs=["text", "text"], outputs="text")
iface.launch()
Or if I’m going for an API:
pip install fastapi uvicorn
# main.py
from fastapi import FastAPI, Request
app = FastAPI()
@app.post("/qa")
async def qa_handler(req: Request):
data = await req.json()
answer = get_answer(data["question"], data["context"], model, tokenizer)
return {"answer": answer}
8. Real-World Tips (from Experience)
Let’s be honest—this is where things get messy.
Fine-tuning BERT for QA sounds straightforward on paper. But in practice? You’ll hit all sorts of weird edge cases, inconsistent results, and GPU bottlenecks. I’ve dealt with more than my fair share of these, so here’s what’s saved me (sometimes just in time).
When the Model Starts Memorizing (a.k.a. Overfitting)
This might surprise you: even massive pretrained models like bert-large-uncased
can start overfitting in just a few epochs—especially with small or noisy datasets.
What’s worked for me:
- Use gradient accumulation to simulate larger batch sizes.
- Add early stopping on exact match or F1 using
TrainerCallback
. - Most importantly, freeze lower layers during initial epochs. I usually keep
embeddings
and the first 4 encoder layers frozen for the first epoch or two, then unfreeze progressively.
# Freeze first few layers (custom training loop)
for name, param in model.named_parameters():
if name.startswith("bert.embeddings") or name.startswith("bert.encoder.layer.0"):
param.requires_grad = False
Handling Noisy QA Pairs
I’ve run into this more than I’d like to admit—misaligned start/end indices, duplicate answers, or even plain wrong answers in the dataset. My rule of thumb?
- Run a manual sanity check on 100 examples. No matter how clean your source claims to be, trust me—there’s always garbage.
- Use
prepare_train_features
to log mismatches. I add print statements for cases whereoffset_mapping
fails to find a match. - If answers are multi-span or fuzzy, normalize with lowercasing, strip punctuation, and compare token-level spans.
Peeking Under the Hood (Debugging Attention & Hidden States)
When something “just feels off” and the loss won’t budge, I’ve hooked into hidden states and attention layers to get a sense of what the model’s focusing on.
def get_attention_hook():
def hook(module, input, output):
print(output[0].shape) # [batch, heads, seq_len, seq_len]
return hook
model.bert.encoder.layer[0].attention.self.register_forward_hook(get_attention_hook())
If you see the attention heads zeroing in on irrelevant tokens, something’s wrong—either with tokenization or your input formatting.
Batch Size vs. GPU Reality
If you’re working with limited VRAM (hello, Colab or 8GB cards), large batch sizes can kill you. What I’ve done instead:
- Set
gradient_accumulation_steps
to 4 or 8. - Use
fp16=True
inTrainingArguments
(as long as you’re not on CPU). - Trim unnecessary features—don’t store
token_type_ids
unless you need them.
Freezing vs. Thawing: What Actually Works
One lesson I learned the hard way: you don’t always need to fine-tune the whole model. If you’re training on domain-specific QA (say legal, clinical, or financial docs), freezing half the layers + just training a few top ones often gives better results with less overfitting.
My go-to setup:
- Freeze bottom half of the encoder.
- Train with a lower learning rate (e.g.,
2e-5
). - Add layer-wise learning rate decay (using
transformers
‘sAdamW
with parameter groups).
9. When BERT Isn’t Enough: Scaling Up
Let’s not pretend BERT is a magic bullet.
Once you start dealing with multi-hop questions, long-form answers, or context windows that span thousands of tokens, BERT starts breaking down.
Here’s where I usually level up:
For Long Contexts: Longformer
If your context is longer than 512 tokens—and truncation isn’t cutting it—Longformer
is your friend. It replaces full self-attention with a sliding window + global attention mechanism, which makes it scalable for longer sequences.
from transformers import LongformerTokenizer, LongformerForQuestionAnswering
model = LongformerForQuestionAnswering.from_pretrained("allenai/longformer-base-4096")
tokenizer = LongformerTokenizer.from_pretrained("allenai/longformer-base-4096")
For Multi-Hop QA: Fusion-in-Decoder (FiD)
When questions require reasoning across multiple passages—think OpenBook QA or Natural Questions—FiD is what I reach for.
It’s an encoder-decoder model that encodes multiple chunks and fuses them in the decoder. HuggingFace has implementations you can build on.
For Hybrid Retrieval: RAG
, ColBERT
, or Custom
Sometimes, you’re not just answering from a static paragraph—you need to fetch relevant docs first. That’s where Retrieval-Augmented Generation (RAG) or ColBERT models shine.
They’re a bit heavier to set up (especially with Faiss or Elasticsearch in the loop), but worth it when dealing with open-domain or large corpora.
10. Final Thoughts + Resources
If you’ve made it this far—congrats. You now have everything you need to fine-tune BERT for question answering in the real world. But more importantly, you’ve got something harder to pick up from docs or tutorials: the practical mindset that comes from trial, error, and late-night debugging.
This guide wasn’t just theory—I’ve built QA pipelines myself, from prototype to production, and everything I’ve shared here comes from lessons learned the hard way.
That said, I know how valuable working examples are. So here’s what you might want to check out next:
Datasets Used
Throughout this guide, I’ve worked with:
squad_v2
– for handling unanswerable questions and real-world QA structure.- Custom CSVs – converted into SQuAD format using a utility function I shared in section 3.3.
If you’re working with internal or messy data, that function will save you a ton of time. (I know it has for me.)
What’s Next?
QA with BERT is just the beginning. If you’re working on real-world deployment or trying to squeeze more performance out of mid-sized hardware, here’s what I’ll be exploring next—and what you might want to look into too:
- Low-Rank Adaptation (LoRA) – lightweight fine-tuning for large models without full retraining.
- Quantization – reduce model size and inference time (especially if you’re heading toward mobile or edge deployment).
- Knowledge-Augmented QA – combining retrieval (e.g., with FAISS) and generation (like FiD or RAG) for complex, open-domain questions.
- Multi-task Learning – sharing encoders between QA and related tasks like NER or summarization.
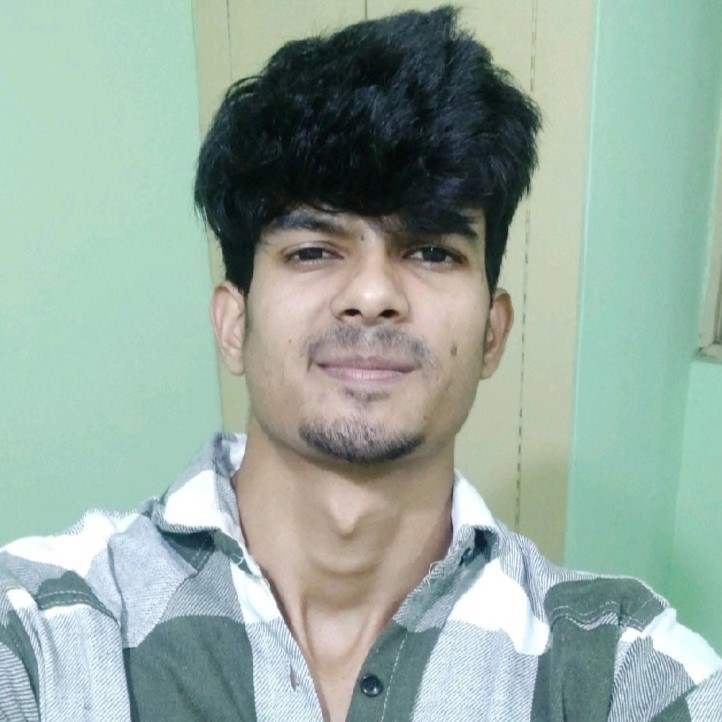
I’m a Data Scientist.