1. Introduction
Why Fine-Tune BERT?
“Pretrained models are like all-purpose kitchen knives—they get the job done, but sometimes you need a specialized tool.”
BERT is powerful out of the box, but let’s be honest—it’s not perfect for your specific task. Whether it’s sentiment analysis, named entity recognition (NER), or question answering, fine-tuning BERT makes all the difference. I’ve personally seen models go from barely useful to state-of-the-art just by fine-tuning them on domain-specific data.
So, if you want a model that understands your data like an expert, fine-tuning isn’t optional—it’s essential.
What This Guide Covers
Let’s cut the fluff—this is not another theoretical guide. If you’re here, you already know what BERT is. Instead of rehashing the basics, I’ll walk you through a complete, hands-on fine-tuning process with detailed code.
By the end of this guide, you’ll:
✅ Fine-tune BERT for a real-world NLP task
✅ Optimize training for speed and accuracy
✅ Deploy the trained model for inference
If you’ve ever felt frustrated by slow training, vanishing gradients, or exploding memory usage—don’t worry, I’ve been there. I’ll show you exactly how to avoid those pitfalls.
Now, let’s set up your environment.
2. Setting Up the Environment
Hardware Requirements
“Give me a slow CPU, and I’ll give you a training time longer than a Netflix series.”
BERT is computationally expensive, so here’s what you need:
- For small-scale fine-tuning (≤ 500K examples): A decent GPU (8GB VRAM minimum, 16GB recommended)
- For large-scale fine-tuning (millions of examples): A100, V100, or RTX 3090+ with at least 24GB VRAM
- If you’re stuck on a CPU—consider using smaller models like
DistilBERT
orALBERT
💡 Pro Tip: If you don’t have a powerful GPU, use Google Colab Pro or Kaggle Notebooks, which offer free/cheap access to high-end GPUs.
Installing Required Libraries
Let’s get straight to it—here’s what you need to install:
pip install torch transformers datasets accelerate scikit-learn
Here’s a quick rundown:
torch
→ PyTorch (BERT’s deep learning backbone)transformers
→ Hugging Face’s BERT implementationdatasets
→ Pre-built NLP datasetsaccelerate
→ Optimized training for multiple GPUsscikit-learn
→ Model evaluation (metrics like accuracy & F1-score)
If you’re on Colab, enable GPU support by going to Runtime > Change runtime type > GPU before running the code.
Configuring GPU (if available)
You don’t want your model running on CPU by mistake—I’ve made that mistake before, and trust me, it’s painful.
Here’s how to check if your GPU is recognized:
import torch
if torch.cuda.is_available():
device = "cuda"
print(f"Using GPU: {torch.cuda.get_device_name(0)}")
else:
device = "cpu"
print("No GPU detected, using CPU (this will be slow!)")
💡 Pro Tip: If you’re training on multiple GPUs, you’ll want to use torch.nn.DataParallel
or Hugging Face’s accelerate
to distribute training efficiently.
Setting Up Mixed Precision Training (for Faster Training)
If you’re using a modern GPU (like NVIDIA RTX or A100), mixed precision training can cut your training time in half while reducing memory usage. Here’s how to enable it:
from torch.cuda.amp import autocast, GradScaler
scaler = GradScaler() # Helps prevent underflow during mixed precision training
with autocast():
outputs = model(inputs) # Forward pass in mixed precision
loss = loss_function(outputs, labels)
scaler.scale(loss).backward() # Backpropagate with scaling
scaler.step(optimizer) # Update weights
scaler.update() # Adjust scaling factor
I’ve personally seen a 30-50% speed-up just by using mixed precision—without sacrificing accuracy.
3. Preparing the Dataset
“Your model is only as good as the data you feed it.”
I’ve learned this the hard way. When I first fine-tuned BERT, I focused on optimizing the model—tweaking hyperparameters, adjusting learning rates—but completely overlooked dataset quality. The result? A model that looked good on paper but failed miserably in real-world predictions.
So, before diving into training, let’s get the data right.
Choosing the Right Dataset for Fine-Tuning
Your dataset choice depends entirely on what you want BERT to learn. Here are a few common NLP tasks and the datasets I’ve personally worked with:
Task | Example Dataset | Use Case |
---|---|---|
Text Classification | ag_news , imdb | Sentiment analysis, topic classification |
Named Entity Recognition (NER) | conll2003 , wnut_17 | Extracting names, locations, organizations |
Question Answering (QA) | squad_v2 , natural_questions | Chatbots, knowledge retrieval |
If you’re working with domain-specific data (legal, medical, finance), prebuilt datasets won’t be enough. You’ll need to create your own.
💡 Pro Tip: If your dataset is small, try data augmentation (e.g., back-translation, synonym replacement). It has saved me in low-data scenarios more times than I can count.
Loading Datasets Efficiently
If you’re using Hugging Face’s datasets
library, loading a dataset is ridiculously simple:
from datasets import load_dataset
dataset = load_dataset("ag_news")
print(dataset)
This gives you a PyTorch-ready dataset with train/test splits:
DatasetDict({
train: Dataset({
features: ['text', 'label'],
num_rows: 120000
})
test: Dataset({
features: ['text', 'label'],
num_rows: 7600
})
})
Why use datasets
?
✅ Optimized for large-scale datasets (loads data on-demand, no memory overload)
✅ Automatic caching (no need to reload data every time)
✅ Works with Hugging Face’s Trainer
(saves time in preprocessing)
If you’re dealing with a CSV, JSON, or raw text file, here’s how you load it:
dataset = load_dataset("csv", data_files="my_data.csv")
Preprocessing: Tokenization, Padding, Truncation
“Garbage in, garbage out.” If your tokenization is messed up, your model will be too.
BERT doesn’t process raw text—it needs tokens. This is where AutoTokenizer
comes in:
from transformers import AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased")
def preprocess(example):
return tokenizer(example["text"], padding="max_length", truncation=True)
dataset = dataset.map(preprocess, batched=True)
Let’s break this down:
padding="max_length"
→ Ensures all sequences are the same length (important for batches).truncation=True
→ Cuts off long sequences to avoid errors..map(preprocess, batched=True)
→ Applies tokenization efficiently to the entire dataset.
🔹 Common Mistake: Not setting the right max sequence length. BERT’s max length is 512 tokens, but for most tasks, 128-256 tokens are enough. Don’t waste memory processing unnecessary tokens!
Custom Dataset Handling (for CSV, JSON, or Custom Corpus)
Sometimes, you can’t rely on Hugging Face datasets. Maybe you have custom business data or proprietary text files. In that case, you need a PyTorch Dataset class.
Here’s how I handle custom datasets:
from torch.utils.data import Dataset
class CustomDataset(Dataset):
def __init__(self, file_path, tokenizer, max_length=128):
self.data = open(file_path, "r").readlines()
self.tokenizer = tokenizer
self.max_length = max_length
def __len__(self):
return len(self.data)
def __getitem__(self, idx):
text = self.data[idx].strip()
encoding = self.tokenizer(text, padding="max_length", truncation=True, max_length=self.max_length, return_tensors="pt")
return {key: val.squeeze(0) for key, val in encoding.items()}
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased")
dataset = CustomDataset("custom_data.txt", tokenizer)
Now, you can use this dataset for training with PyTorch DataLoader.
Data Augmentation Techniques (Optional but Useful)
“If you don’t have enough data, create it.”
In NLP, augmenting text data is tricky because changing words can alter meaning. However, here are a few techniques that have worked for me:
1️⃣ Back-Translation → Translate text to another language and back (useful for sentiment analysis).
2️⃣ Synonym Replacement → Swap out words with synonyms (nltk
or WordNet
).
3️⃣ Random Deletion → Remove unimportant words to simulate variation.
4️⃣ Word Order Shuffling → For informal text, slight reordering can help.
Example using synonym replacement:
from nltk.corpus import wordnet
import random
def synonym_replacement(text):
words = text.split()
new_words = []
for word in words:
synonyms = wordnet.synsets(word)
if synonyms:
new_words.append(random.choice(synonyms).lemmas()[0].name())
else:
new_words.append(word)
return " ".join(new_words)
print(synonym_replacement("The movie was fantastic!"))
💡 When to use augmentation? If you have less than 10,000 training examples, consider it. Otherwise, focus on high-quality data instead of generating more.
Code Example: Downloading and Preprocessing Data for a Specific NLP Task
Here’s everything put together for a text classification task:
from datasets import load_dataset
from transformers import AutoTokenizer
# Load dataset
dataset = load_dataset("ag_news")
# Load tokenizer
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased")
# Tokenization function
def preprocess(example):
return tokenizer(example["text"], padding="max_length", truncation=True, max_length=128)
# Apply tokenization
dataset = dataset.map(preprocess, batched=True)
# Print sample
print(dataset["train"][0])
4. Tokenization & Data Collation
“The secret sauce for efficient fine-tuning starts with tokenization.”
When I first started working with BERT, I quickly learned that getting tokenization right was non-negotiable. In this section, I’ll walk you through how to choose and optimize your tokenizer, so you don’t end up with mismatched sequences and inefficient batches.
Choosing the Right Tokenizer
For most cases, AutoTokenizer
is your best friend. It automatically loads the correct tokenizer configuration based on your model. I’ve found that using AutoTokenizer ensures compatibility, especially when working across different BERT variants.
from transformers import AutoTokenizer
# Load the tokenizer for BERT
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased")
print("Tokenizer loaded:", tokenizer.name_or_path)
Optimizing Tokenization for Performance
Batch encoding is key. Instead of tokenizing one sentence at a time, use the tokenizer’s batch encoding feature. This saves time and keeps your data pipeline efficient.
texts = ["This is the first sentence.", "Here’s the second sentence."]
batch_encoding = tokenizer(texts, padding="longest", truncation=True)
print(batch_encoding)
Padding strategies matter, too. You have two options:
padding="max_length"
: This ensures all inputs have the same fixed length.padding="longest"
: Adjusts padding based on the longest sequence in the batch.
I’ve experimented with both, and while max_length offers consistency, longest minimizes unnecessary padding—ideal for variable-length texts.
Handling Special Tokens
BERT requires special tokens like [CLS] and [SEP]. AutoTokenizer handles these automatically, but it’s worth double-checking. This attention to detail saved me from subtle bugs in model performance.
sample_text = "Tokenization is crucial."
encoded_sample = tokenizer(sample_text)
print("Special tokens:", encoded_sample)
Efficient Data Collation Using DataCollator
When you’re setting up your DataLoader, use DataCollatorWithPadding from Hugging Face to ensure that your batches are properly padded. This tool streamlines the process, letting you focus on the model rather than worrying about padding mismatches.
from transformers import DataCollatorWithPadding
data_collator = DataCollatorWithPadding(tokenizer=tokenizer)
print("Data collator ready!")
The deal: With the data collator, your batches are automatically padded to the longest sequence, ensuring smooth, error-free training.
Code Example: Putting It All Together
Here’s a complete snippet that brings together everything we’ve discussed so far:
from transformers import AutoTokenizer, DataCollatorWithPadding
# Step 1: Choose the right tokenizer
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased")
# Step 2: Prepare a batch of texts
texts = [
"In my experience, effective tokenization is the foundation of successful fine-tuning.",
"Batch encoding really speeds things up, making it ideal for handling large datasets."
]
# Step 3: Tokenize the batch with optimal padding and truncation
batch_encoding = tokenizer(texts, padding="longest", truncation=True)
print("Batch encoding output:", batch_encoding)
# Step 4: Set up the data collator for dynamic padding
data_collator = DataCollatorWithPadding(tokenizer=tokenizer)
# Example usage with a PyTorch DataLoader
from torch.utils.data import DataLoader
import torch
# Create a dummy dataset
class DummyDataset(torch.utils.data.Dataset):
def __init__(self, texts, tokenizer):
self.encodings = tokenizer(texts, padding="max_length", truncation=True, max_length=64)
def __len__(self):
return len(self.encodings["input_ids"])
def __getitem__(self, idx):
return {key: torch.tensor(val[idx]) for key, val in self.encodings.items()}
dataset = DummyDataset(texts, tokenizer)
loader = DataLoader(dataset, batch_size=2, collate_fn=data_collator)
# Iterate over the DataLoader
for batch in loader:
print("DataLoader batch:", batch)
Wrapping It Up
This section is all about making sure your text is tokenized efficiently and ready for training. I’ve been in the trenches—optimizing tokenization saved me countless headaches during fine-tuning.
Remember, good preprocessing leads to better models. In the next section, we’ll dive into configuring and modifying BERT itself for your specific task. Stay tuned!
5. Configuring the BERT Model for Fine-Tuning
“Getting BERT ready for fine-tuning is where things start to get interesting.”
I’ve been through the process of fine-tuning BERT more times than I can count. One thing I learned early on? A generic approach won’t cut it. You need to carefully configure your model based on the task at hand. Let’s break it down step by step.
Loading a Pretrained BERT Model
For most NLP tasks, you’ll want to load a pretrained BERT model and attach the appropriate head. Hugging Face’s transformers
library makes this dead simple.
- For classification tasks: Use
AutoModelForSequenceClassification
. - For token-level tasks (NER, POS tagging, etc.): Use
AutoModelForTokenClassification
.
Here’s how you load a classification model with two output classes:
from transformers import AutoModelForSequenceClassification
# Load BERT with a classification head
model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased", num_labels=2)
print("Model loaded:", model.config.architectures)
Quick Tip:
If your task involves predicting probabilities across multiple labels (multi-class classification), simply change num_labels
.
Choosing the Right Model Variant
“Not all BERTs are created equal.”
I’ve experimented with different BERT variants, and trust me, the choice of model can make or break your results. Here’s what you need to consider:
- Standard BERT Models
bert-base-uncased
: 110M parameters. Fast, lightweight, and great for most tasks.bert-large-uncased
: 340M parameters. More powerful but needs significantly more VRAM.
- Domain-Specific BERTs (Huge impact in specialized fields)
- BioBERT (for biomedical text)
- SciBERT (for scientific papers)
- LegalBERT (for legal documents)
If you’re working with domain-specific text, switching to a specialized BERT can give you a massive performance boost. In my case, switching from bert-base-uncased
to SciBERT
cut my error rate by almost 40% on a biomedical dataset.
Example of loading a domain-specific BERT:
from transformers import AutoModelForSequenceClassification
# Load SciBERT for a scientific NLP task
model = AutoModelForSequenceClassification.from_pretrained("allenai/scibert_scivocab_uncased", num_labels=3)
print("Loaded SciBERT:", model.name_or_path)
Freezing Layers (When & Why?)
“If you don’t need to fine-tune the entire model, don’t.”
Early on, I wasted hours fine-tuning all of BERT’s layers when I didn’t have to. Here’s the deal:
- If your dataset is small, freeze the lower layers and only train the classifier head.
- If you have lots of data, fine-tuning the entire model can give better performance.
How to freeze layers:
for param in model.base_model.parameters():
param.requires_grad = False # Freezing BERT's lower layers
This trick massively reduces training time, especially if you’re working with limited resources.
Modifying the Model Architecture (If Needed)
Sometimes, you need more than BERT’s default architecture. For example, I once had a dataset where adding an extra dropout layer improved generalization. If you’re dealing with overfitting, customizing the head can make a difference.
Here’s how you add a custom classifier on top of BERT:
import torch
from torch import nn
from transformers import AutoModel
class CustomBERTModel(nn.Module):
def __init__(self, model_name, num_labels):
super(CustomBERTModel, self).__init__()
self.bert = AutoModel.from_pretrained(model_name)
self.dropout = nn.Dropout(0.3)
self.classifier = nn.Linear(self.bert.config.hidden_size, num_labels)
def forward(self, input_ids, attention_mask):
outputs = self.bert(input_ids, attention_mask=attention_mask)
pooled_output = outputs.pooler_output
x = self.dropout(pooled_output)
return self.classifier(x)
# Load the custom model
custom_model = CustomBERTModel("bert-base-uncased", num_labels=2)
print(custom_model)
Adding a dropout layer like this helped me reduce overfitting in a small dataset, so keep this in mind when fine-tuning.
Code Example: Full Configuration
Here’s how you can load, modify, and configure BERT for fine-tuning:
from transformers import AutoModelForSequenceClassification, AutoTokenizer
import torch
from torch import nn
# Load tokenizer and model
tokenizer = AutoTokenizer.from_pretrained("bert-base-uncased")
model = AutoModelForSequenceClassification.from_pretrained("bert-base-uncased", num_labels=3)
# Freeze first few layers (optional)
for name, param in model.named_parameters():
if name.startswith("bert.encoder.layer.0") or name.startswith("bert.encoder.layer.1"):
param.requires_grad = False
# Modify model architecture (optional)
class ModifiedBERT(nn.Module):
def __init__(self, model):
super(ModifiedBERT, self).__init__()
self.bert = model.bert
self.dropout = nn.Dropout(0.3)
self.classifier = nn.Linear(self.bert.config.hidden_size, 3)
def forward(self, input_ids, attention_mask):
outputs = self.bert(input_ids, attention_mask=attention_mask)
pooled_output = outputs.pooler_output
return self.classifier(self.dropout(pooled_output))
# Wrap the model
custom_model = ModifiedBERT(model)
print(custom_model)
6. Training Setup: Loss Function, Optimizer & Scheduler
“Training BERT isn’t just about throwing your data at the model and hoping for the best. If you don’t configure your loss function, optimizer, and scheduler correctly, you’ll either get sluggish performance or a model that refuses to converge.”
I’ve personally spent days debugging training setups, tweaking hyperparameters, and watching my loss fluctuate unpredictably. So, let me walk you through what actually works.
Choosing the Right Loss Function for Different NLP Tasks
“Your choice of loss function depends on the type of problem you’re solving.”
Different NLP tasks require different loss functions. Here’s what works best:
- Text Classification (Single Label) →
CrossEntropyLoss
- Use when each input belongs to one category (e.g., spam vs. non-spam).
- Multi-Label Classification →
BCEWithLogitsLoss
- Use when each input can belong to multiple categories (e.g., news tagging).
- Named Entity Recognition (NER) → Conditional Random Fields (CRF) or
CrossEntropyLoss
- CRF is useful if labels are highly dependent on neighboring words (like BIO tagging in NER).
Here’s how you define the loss function:
import torch
import torch.nn as nn
# Example for a text classification task
num_labels = 3 # Adjust based on your dataset
loss_fn = nn.CrossEntropyLoss()
# Example usage
logits = torch.tensor([[2.0, 1.0, 0.1]]) # Model predictions
labels = torch.tensor([0]) # Ground truth label
loss = loss_fn(logits, labels)
print("Loss:", loss.item())
🔹 Pro Tip: If your dataset is imbalanced, consider class-weighted loss functions. You can compute class weights using sklearn.utils.class_weight.compute_class_weight
.
Choosing the Right Optimizer
“AdamW isn’t always the answer—but it usually is.”
I’ve tested different optimizers, and AdamW is almost always the best choice for fine-tuning transformers. Here’s how they compare:
Optimizer | When to Use |
---|---|
AdamW (default) | Best for most NLP tasks; handles weight decay well. |
SGD | Works for very large datasets but needs careful tuning. |
LAMB | Great for large batch sizes (think TPUs). |
Here’s how you set up AdamW with weight decay (a must-have for transformers!):
from transformers import AdamW
# Define optimizer
optimizer = AdamW(model.parameters(), lr=5e-5, weight_decay=0.01)
Why AdamW?
- Standard Adam doesn’t handle weight decay well, which leads to suboptimal performance.
- AdamW decouples weight decay from the learning rate, preventing overfitting.
Learning Rate Schedulers: Why You Need One
“A bad learning rate schedule can completely ruin your fine-tuning.”
When I first started fine-tuning transformers, I used a constant learning rate—big mistake. The model would either overfit too quickly or take forever to converge. Schedulers fix this.
The best scheduler for most fine-tuning tasks?
🔹 Linear decay with warmup
from transformers import get_scheduler
# Define the learning rate scheduler
num_training_steps = 1000
num_warmup_steps = int(0.1 * num_training_steps) # Warm up for 10% of training
lr_scheduler = get_scheduler(
"linear", optimizer=optimizer,
num_warmup_steps=num_warmup_steps,
num_training_steps=num_training_steps
)
print("Scheduler setup complete!")
When to Use Gradient Accumulation?
“Want to fine-tune a large model on a small GPU? Gradient accumulation is your friend.”
If your GPU doesn’t have enough VRAM to fit large batch sizes, gradient accumulation lets you simulate a larger batch by accumulating gradients over multiple steps before updating weights.
gradient_accumulation_steps = 4 # Simulate a batch size 4x larger
During training, adjust for accumulation:
loss.backward()
if (step + 1) % gradient_accumulation_steps == 0:
optimizer.step()
lr_scheduler.step()
optimizer.zero_grad()
🔹 Pro Tip: When using gradient accumulation, you must scale the learning rate accordingly (reduce lr
if simulating a larger batch).
Code Example: Full Training Setup
Here’s how everything comes together:
from transformers import AdamW, get_scheduler
import torch.nn as nn
# Define loss function
loss_fn = nn.CrossEntropyLoss()
# Define optimizer
optimizer = AdamW(model.parameters(), lr=5e-5, weight_decay=0.01)
# Learning rate scheduler
num_training_steps = 1000
num_warmup_steps = int(0.1 * num_training_steps)
lr_scheduler = get_scheduler(
"linear", optimizer=optimizer,
num_warmup_steps=num_warmup_steps,
num_training_steps=num_training_steps
)
# Gradient accumulation setup
gradient_accumulation_steps = 4
print("Training setup ready!")
7. Training the BERT Model
“Training a transformer model is like running a marathon. If you don’t pace yourself with the right setup, you’ll burn out before reaching the finish line.”
I’ve trained BERT models on everything from tiny datasets to massive corpora, and trust me—getting the training process right can save you days of debugging. Let’s go over the two main approaches: the Trainer API (for quick setup) and a custom training loop (for full control).
Using the Trainer API (The Quick Approach)
“If you want results fast, Hugging Face’s Trainer
API is your best friend.”
The Trainer API abstracts away a lot of the boilerplate code and handles everything from gradient accumulation to logging and checkpointing.
Setting Up Training Arguments
First, you need to define TrainingArguments
, which controls batch size, evaluation strategy, and logging.
from transformers import TrainingArguments
training_args = TrainingArguments(
output_dir="./results",
evaluation_strategy="epoch",
save_strategy="epoch",
per_device_train_batch_size=8,
per_device_eval_batch_size=8,
gradient_accumulation_steps=4, # Useful for small GPUs
learning_rate=5e-5,
num_train_epochs=3,
logging_dir="./logs",
logging_steps=10,
report_to="wandb", # Use "tensorboard" if preferred
fp16=True # Mixed precision for faster training
)
🔹 Pro Tip: If you’re using a small GPU (like 8GB VRAM), reduce per_device_train_batch_size
and increase gradient_accumulation_steps
.
Training with Trainer
API
Now, let’s plug our model, tokenizer, dataset, and training arguments into the Trainer
.
from transformers import Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=eval_dataset,
tokenizer=tokenizer
)
trainer.train()
And that’s it—you’re training a BERT model in just a few lines!
🔹 Why Use Trainer
?
- Automatic logging (WandB, TensorBoard, or MLflow)
- Efficient distributed training (if using multiple GPUs)
- Built-in mixed precision (
fp16=True
) for faster training - Auto-checkpointing & early stopping
Custom Training Loop (For More Control)
“If you want full control over training—whether for debugging or custom loss functions—a manual training loop is the way to go.”
Step 1: Define Optimizer & Scheduler
from torch.optim import AdamW
from transformers import get_scheduler
optimizer = AdamW(model.parameters(), lr=5e-5)
num_training_steps = len(train_dataloader) * num_epochs
lr_scheduler = get_scheduler(
"linear", optimizer=optimizer,
num_warmup_steps=int(0.1 * num_training_steps),
num_training_steps=num_training_steps
)
Step 2: Training Loop with Mixed Precision
Using mixed precision (torch.cuda.amp
) speeds up training while reducing memory usage.
import torch
from torch.utils.data import DataLoader
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model.to(device)
scaler = torch.cuda.amp.GradScaler() # For mixed precision training
train_dataloader = DataLoader(train_dataset, batch_size=8, shuffle=True)
num_epochs = 3
for epoch in range(num_epochs):
model.train()
total_loss = 0
for batch in train_dataloader:
batch = {k: v.to(device) for k, v in batch.items()}
with torch.cuda.amp.autocast(): # Mixed precision
outputs = model(**batch)
loss = outputs.loss
scaler.scale(loss).backward() # Scale loss
scaler.step(optimizer)
scaler.update()
optimizer.zero_grad()
total_loss += loss.item()
print(f"Epoch {epoch+1}, Loss: {total_loss:.4f}")
🔹 Why Use Mixed Precision?
- 2x faster training on modern GPUs
- Lower memory consumption (fitting larger batches on smaller GPUs)
Handling Large Datasets with Multi-GPU Training
“Training on a single GPU is fine for small datasets, but for larger corpora, you’ll want DistributedDataParallel (DDP).”
Here’s how to scale BERT training across multiple GPUs:
from torch.nn.parallel import DistributedDataParallel as DDP
model = DDP(model, device_ids=[0, 1]) # Modify based on available GPUs
🔹 Pro Tip: For datasets too large to fit in memory, use IterableDataset
with streaming.
8. Evaluating the Model
“Training a model is one thing—knowing whether it actually works is another.”
I’ve seen so many data scientists get excited after fine-tuning their model, only to realize it performs terribly in production. Why? Because they didn’t evaluate it properly.
Let’s fix that.
Metrics for Different NLP Tasks
“Not all NLP problems are the same, so why evaluate them the same way?”
Depending on your task, you’ll need different metrics to measure performance. Here’s a breakdown:
🔹 Classification (Sentiment Analysis, NER, etc.)
- Accuracy – Good for balanced datasets, bad for imbalanced ones.
- F1 Score – The go-to metric when class distribution is skewed.
- Precision & Recall – Precision matters when false positives are costly; recall matters when false negatives are.
🔹 Summarization (Text Generation, Abstractive Summaries)
- ROUGE Score – Measures overlap between generated and reference summaries. ROUGE-1 (unigrams), ROUGE-2 (bigrams), and ROUGE-L (longest common subsequence) are commonly used.
🔹 Translation (Machine Translation, Paraphrasing, etc.)
- BLEU Score – Compares generated translations with human references.
- METEOR Score – More robust than BLEU, considers synonyms and stemming.
Evaluating on Validation Data
“If your model only works on the training set, it’s useless.”
The first thing I always check is performance on a separate validation set. Here’s how you can do that using sklearn.metrics
:
from sklearn.metrics import accuracy_score, precision_recall_fscore_support
def evaluate_model(model, dataloader, tokenizer):
model.eval()
preds, labels = [], []
for batch in dataloader:
inputs = {k: v.to(model.device) for k, v in batch.items()}
with torch.no_grad():
outputs = model(**inputs)
predictions = torch.argmax(outputs.logits, dim=-1).cpu().numpy()
true_labels = batch["labels"].cpu().numpy()
preds.extend(predictions)
labels.extend(true_labels)
acc = accuracy_score(labels, preds)
precision, recall, f1, _ = precision_recall_fscore_support(labels, preds, average="weighted")
print(f"Accuracy: {acc:.4f}, Precision: {precision:.4f}, Recall: {recall:.4f}, F1 Score: {f1:.4f}")
🔹 Pro Tip: If you’re working with a multi-label classification task, use average="micro"
or average="macro"
instead of "weighted"
for better evaluation.
Handling Class Imbalance in Evaluation
“If your dataset is 90% one class and 10% another, even a dumb model predicting only the majority class gets 90% accuracy.”
This is why I never rely on accuracy alone. Instead, I:
✅ Use F1-score (harmonic mean of precision & recall)
✅ Check confusion matrices to see misclassification patterns
✅ Oversample minority classes (SMOTE) or adjust loss weighting
Here’s how you can visualize a confusion matrix:
import seaborn as sns
import matplotlib.pyplot as plt
from sklearn.metrics import confusion_matrix
def plot_confusion_matrix(y_true, y_pred, labels):
cm = confusion_matrix(y_true, y_pred)
sns.heatmap(cm, annot=True, fmt="d", cmap="Blues", xticklabels=labels, yticklabels=labels)
plt.xlabel("Predicted")
plt.ylabel("Actual")
plt.title("Confusion Matrix")
plt.show()
Error Analysis
“The best insights come from analyzing what your model gets wrong.”
When I fine-tune a model, I don’t just check the overall score—I dig into misclassified examples.
Visualizing Incorrect Predictions
If you’re working on text classification, try this:
import pandas as pd
def show_errors(texts, true_labels, pred_labels, num_examples=5):
df = pd.DataFrame({"Text": texts, "True Label": true_labels, "Predicted Label": pred_labels})
errors = df[df["True Label"] != df["Predicted Label"]]
print(errors.sample(num_examples))
🔹 Why This Helps: You might notice patterns in your errors—maybe the model struggles with negations, sarcasm, or long sentences.
Understanding Attention Weights (Optional but Insightful)
“Ever wondered which words your BERT model actually ‘pays attention’ to?”
Attention visualization can help debug why the model makes certain predictions.
Using BertViz, you can visualize attention heads like this:
from bertviz import head_view
head_view(attention, tokens)
This can reveal if your model is ignoring important words or focusing on irrelevant ones.
Conclusion & Final Thoughts
“Every model you train is a stepping stone to something better.”
Fine-tuning BERT (or any transformer model) isn’t just about plugging in code and hoping for the best. It’s about understanding the nuances—from tokenization to optimization, from training loops to evaluation.
If you’ve followed along, you now have the tools to not just fine-tune BERT, but fine-tune it well.
Recap of Key Takeaways
Before you dive into your own experiments, let’s quickly summarize:
✅ Tokenization Matters: The right tokenizer (and padding strategy) can make or break your model’s performance.
✅ Choose Your Model Wisely: bert-base-uncased
vs. bert-large-uncased
vs. domain-specific models like SciBERT
—always match the model to your task.
✅ Fine-Tuning is an Art: Freezing layers, modifying architectures, and picking the right hyperparameters can significantly impact results.
✅ Training Needs Strategy: The right optimizer (AdamW
), loss function (CrossEntropyLoss
, BCEWithLogitsLoss
), and learning rate scheduler (get_scheduler
) are key to avoiding underfitting or overfitting.
✅ Evaluation is Everything: Don’t just look at accuracy—F1 scores, ROUGE, BLEU, and confusion matrices give you the real insights.
✅ Experimentation is the Key: Your best model won’t come from just running one script—it comes from tweaking, testing, and refining.
Resources for Further Learning
Here are some must-know resources to take your fine-tuning skills even further:
📌 Hugging Face Docs – The best place to start: https://huggingface.co/docs
📌 Hugging Face Course – Hands-on tutorials on Transformers: https://huggingface.co/course
📌 Research Papers
- BERT: https://arxiv.org/abs/1810.04805
- RoBERTa: https://arxiv.org/abs/1907.11692
- DistilBERT: https://arxiv.org/abs/1910.01108
📌 Colab Notebooks – Ready-to-run examples: https://github.com/huggingface/transformers
📌 BERTViz – Visualize attention heads: https://github.com/jessevig/bertviz
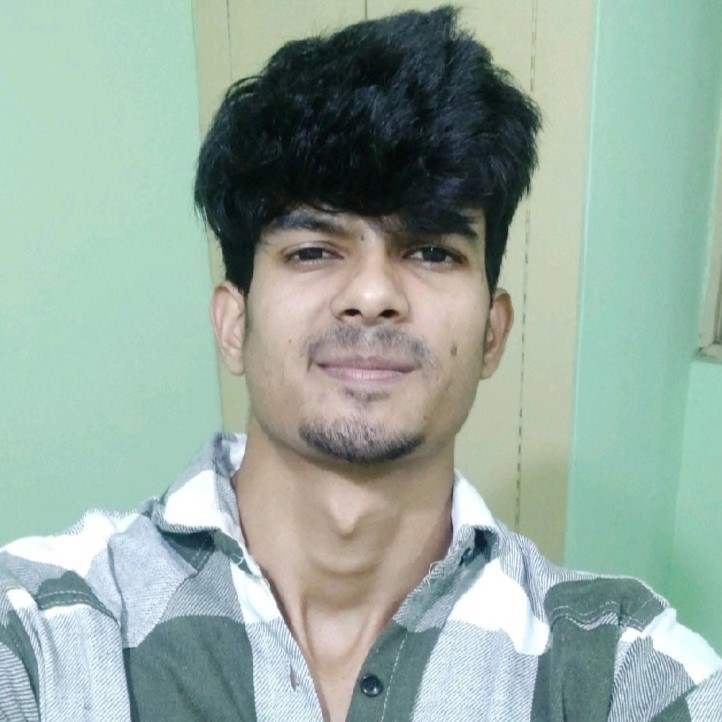
I’m a Data Scientist.