Introduction: What This Guide Covers — and What It Doesn’t
“Ship fast, iterate faster — but only if it works in production.”
If you’re like me, you’re probably tired of tutorials that show you how to play with toy datasets, throw a model behind an API, and call it an “AI app.” That’s not what we’re doing here.
In this guide, I’m walking you through exactly how I’ve built production-grade AI apps in 2025 — not just the LLM side, but the entire architecture: context retrieval, API backend, observability, and full-stack deployment. The stuff that actually matters when users are clicking buttons and expecting results in milliseconds.
You won’t find hand-holding or entry-level theory here. I’m assuming you already understand how transformers work, what embeddings are, and why prompt injection is a real threat. What you’ll get is practical, cut-to-the-chase implementation, backed by my own experience using tools like:
- OpenAI’s GPT-4 Turbo & GPT-4V
- LangChain (yes, still relevant in 2025)
- RAG pipelines (with vector DBs like Qdrant or Weaviate)
- FastAPI for serving
- Docker for containerization
- And deployment via Render, GCP, or Vercel, depending on the stack.
This is an end-to-end guide — from defining a usable app concept all the way to deploying it where real users can interact with it.
1. Define the Use Case with Constraints in Mind
This might surprise you: one of the biggest mistakes I see data scientists make when building AI apps is starting with the model instead of the system constraints. I’ve done that myself early on — trained a powerful model only to realize it couldn’t be used due to latency or API pricing.
So here’s how I approach it now:
Step 1: Pick the Right Type of App
Before writing a line of code, I ask myself: What’s the core interaction between user and AI?
- Is it a multi-turn conversation? (e.g. chatbot)
- Is it an agent tool? (e.g. code assistant, file analyst)
- Is it search + summarization? (e.g. AI research analyst)
- Is it multimodal? (e.g. OCR + vision reasoning)
For this guide, I’m picking an AI research assistant — a tool I’ve personally built and iterated on — which can take in academic papers and return meaningful, query-aware summaries. No gimmicks, just practical value for researchers.
Step 2: Map Out the Constraints
Once you’ve locked in the use case, it’s time to be brutally honest about the constraints. Here’s what I typically ask myself:
- Latency tolerance: Can I afford a 3-second LLM response?
- Privacy boundaries: Are we handling user-uploaded PDFs with sensitive info?
- Inference cost: Are we calling GPT-4 on every query, or batching/switching to Turbo-3.5 where possible?
- Hosting flexibility: Is this app going behind a firewall or on the open internet?
From my experience, planning for these early saves hours — if not days — of architectural rewrites down the line.
Step 3: Choose the Right Model Modality
At this point, don’t just pick the shiniest model. Instead, match model capability to task complexity:
- GPT-4 Turbo is still my go-to for nuanced text summarization + function calling.
- Claude Opus can be better with long-form reasoning.
- GPT-4V or Gemini 1.5 Pro are great for when you need visual understanding (e.g. reading charts or scanned documents).
When building my research assistant, I used text-embedding-3-large for chunk indexing and GPT-4 for generation, with cost-effective fallbacks to GPT-3.5 for simple queries.
2. System Architecture Design (With Diagram)
“An architecture isn’t just what you draw — it’s what doesn’t break in prod.”
When I built my first AI research assistant last year, I made the mistake of coupling everything — vector search, generation, UI, you name it. It worked, until it didn’t. Scaling beyond 10 users? Dead. Updating a model version? Nightmare. That experience taught me the hard way: modular architecture isn’t optional.
You might be wondering what the real-world setup looks like. Here’s how I structure things now — for scalability, observability, and flexibility across different use cases.
Architecture Overview (Real One, Not Toy-Level)
Here’s the actual breakdown I use in production today:
- Frontend (React/Vite/Next.js) — handles chat UI, document upload, auth.
- Backend (FastAPI) — glues everything together: routing, RAG orchestration, auth tokens, rate limiting.
- LLM Layer — OpenAI GPT-4 or self-hosted Ollama (depending on project), with smart fallback logic to Turbo or Claude.
- Vector Store — Weaviate or Qdrant. Pinecone is fast but expensive — I’ve found Qdrant hits the sweet spot for most mid-scale apps.
- Retriever Logic — LangChain or vanilla RAG logic (chunker + embedder + retriever).
- Telemetry Layer — Logs all prompt+response pairs, latency, token counts, and failure states for analysis.
Here’s a clean version of that in a Mermaid diagram:
graph TD
UI[User Interface (Next.js / Vite)] --> API[FastAPI Backend]
API -->|Prompt + Metadata| LLM[OpenAI API / Ollama / Claude]
API --> VectorDB[Qdrant / Weaviate / Pinecone]
API --> RAG[Retriever Pipeline (Chunker + Embedder)]
API --> Logger[Telemetry + Logging (Prometheus / custom)]
Sync vs Async Flows
Let me tell you — this part is where performance bottlenecks can hide in plain sight.
Sync flow works fine for:
- Lightweight Q&A
- Single-document lookup
- Simple chat
But the moment you’re fetching chunks, hitting OpenAI, calling a reranker, and updating user sessions? That’s when async orchestration pays off.
Personally, I use async def
with httpx.AsyncClient()
inside FastAPI to parallelize:
- Chunk retrieval
- Embedding similarity lookup
- LLM call (especially when calling multiple models or fallback APIs)
Here’s a minimal FastAPI snippet I’ve used in one of my async setups:
from fastapi import FastAPI
import httpx, asyncio
app = FastAPI()
@app.get("/query")
async def handle_query(q: str):
async with httpx.AsyncClient() as client:
vectordb_task = client.post("http://vectordb.local/query", json={"q": q})
llm_task = client.post("https://api.openai.com/v1/chat/completions", json={...})
results = await asyncio.gather(vectordb_task, llm_task)
return {"vector_response": results[0].json(), "llm_response": results[1].json()}
LLM Hosting vs API Usage
Here’s the deal: I’ve used both self-hosted models and OpenAI APIs. Each has tradeoffs:
Aspect | Hosted LLM (e.g. Ollama) | OpenAI API (e.g. GPT-4) |
---|---|---|
Latency | Fast for short inputs | Variable, sometimes spiky |
Cost | Fixed infra, no token cost | Pay-as-you-go (can get $$) |
Reliability | You own it — more control | SLA backed, less maintenance |
Capabilities | Depends on model (e.g. LLaMA3) | Best-in-class (GPT-4 Turbo) |
In practice, I often prototype with OpenAI and gradually shift inference to Ollama/LM Studio when:
- The prompt structure is locked in
- The performance is acceptable
- I’m looking to avoid API costs at scale
Other Non-Negotiables in My Stack
Here are some production patterns I always use now:
- Response caching (Redis or SQLite, even file-based if needed). Never pay for the same call twice.
- Timeouts and retries on every external call. GPT-4 will fail on you when you least expect it.
- Structured prompt templates stored in versioned JSON or YAML — so I can test prompt changes like code diffs.
- Telemetry hooks that log input/output pairs, latency, and prompt templates used — this is how I debug and optimize responses later.
3. Tooling Stack & Environment Setup (What I Actually Use)
“The tools don’t build the house — but bad tools sure as hell slow it down.”
I’ve gone through enough broken Python environments, dependency hells, and surprise CI/CD failures to know this: a good environment setup saves hours, if not days. What you’re getting here isn’t theoretical — it’s what I use right now in my AI app stack.
Let’s break it down.
Dependency Management — Poetry or pip-tools (Pick One)
I personally use poetry
for 90% of my projects now. It’s clean, predictable, and doesn’t mess with global environments.
poetry init
poetry add openai langchain fastapi uvicorn pydantic[dotenv] qdrant-client
That one line sets you up with everything you’ll need for a backend that talks to OpenAI, performs RAG with Qdrant, and spins up with FastAPI.
If you’re more into pip-tools
, that’s totally fine — just don’t use raw pip freeze > requirements.txt
in 2025. It’s outdated, bloated, and breaks reproducibility.
Productivity Stack: VSCode, Dotenv, and Dev Containers
Let’s be real — if your .env
file leaks into production, you’re gonna have a bad time. So here’s how I structure my dev setup:
/app
├── .env.local # Local dev secrets
├── .env.example # Template with variable names only
├── main.py
├── api/
└── utils/
And in main.py
, load your environment safely:
from dotenv import load_dotenv
import os
load_dotenv(dotenv_path=".env.local")
OPENAI_API_KEY = os.getenv("OPENAI_API_KEY")
if not OPENAI_API_KEY:
raise RuntimeError("OPENAI_API_KEY is not set in environment variables")
I also use VSCode dev containers when I want the environment to be fully reproducible across teammates. Here’s a devcontainer.json
config that’s worked well for me:
{
"name": "ai-app-dev",
"dockerFile": "Dockerfile",
"postCreateCommand": "poetry install",
"settings": {
"terminal.integrated.defaultProfile.linux": "bash"
},
"remoteEnv": {
"PYTHONPATH": "/workspace"
}
}
It’s especially useful when you’re using ollama
or other local tools that need consistent Docker access across dev machines.
Bonus: AI Code Tools That Actually Help
I’ve tried a bunch of them — and here’s what actually stuck:
- GitHub Copilot: surprisingly useful for boilerplate FastAPI routes, pydantic models, and chunked loop patterns.
- Cody by Sourcegraph: excellent for navigating large codebases, especially when revisiting older LangChain logic or custom RAG flows.
- Continue.dev: VSCode-native agent for answering codebase-specific questions. A little buggy, but shows promise.
I personally don’t rely on these for “thinking” — but they’re great for reducing the repetitive stuff that clutters your editor.
Real Secrets Management (Don’t Just Hope .env Works)
Here’s the deal: .env
is fine for local, but for production you’ll want:
- GCP Secret Manager or AWS Secrets Manager for cloud-hosted apps
python-decouple
orpydantic-settings
for more structured config handling- Docker
--env-file
option when deploying containers
I’ve made the mistake before of assuming os.environ
would magically have everything — only to realize later that secrets weren’t mounted correctly in my cloud runner. Now I always run a local check_env.py
validation step that raises an error on missing variables before anything boots.
This might sound like a lot, but once you set it up once, it’s your foundation. Everything else — LLMs, vector search, orchestration — builds on top of this clean stack.
4. Building the Core ML/LLM Logic (Battle-Tested Patterns)
“You don’t need more tokens. You need smarter prompts.”
This is where most AI apps quietly fall apart — not because the model is bad, but because the prompt design, memory strategy, or inference method is an afterthought. I’ll break this down into two tracks: proprietary APIs (like OpenAI, Anthropic) and custom/self-hosted models (via vLLM, Ollama, etc.).
a. If You’re Using OpenAI or Proprietary APIs
Dynamic Prompt Engineering (Not Just “You are a helpful assistant”)
I can’t stress this enough — prompt composition needs to be programmatic. Hardcoding role + user messages is fine for toys, but in production, your app logic should dynamically shape prompts based on:
- User goal
- App context/state
- Memory (if any)
- Tool/function availability
Here’s a simplified version of what I use for function calling apps:
from openai import OpenAI
client = OpenAI()
response = client.chat.completions.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You're a scientific assistant. Be concise and accurate."},
{"role": "user", "content": "Find the latest research on diffusion models"}
],
functions=[
{
"name": "search_papers",
"description": "Search semantic scholar for recent AI research",
"parameters": {
"type": "object",
"properties": {
"query": {"type": "string"}
},
"required": ["query"]
}
}
],
function_call="auto"
)
I’ve found that setting tight function schemas drastically reduces hallucinated function arguments. Also, setting function_call="auto"
often works better than manually injecting function calls — the model tends to behave more naturally when it controls the tool usage.
Token Efficiency + Hallucination Control
You might be wondering: How do you stop the model from going off the rails?
Here’s what I do:
- Few-shot examples are overrated for long contexts — use templated messages with slot-filling and in-context constraints instead.
- If the model tends to invent facts, force it into “citation mode” with phrases like:
“Always respond using direct quotes from the provided content. If unsure, say ‘I don’t know.'” - Use
logit_bias
to steer completions if you’re generating structured outputs (e.g. “yes”/“no”)
And keep your input tight — I’ve personally had cost overruns just because a single user query bloated the context window by 6K tokens from bad prompt merges. Clean, predictable prompts always win.
b. If You’re Using Custom Models (vLLM, Ollama, HF)
Here’s the deal: hosting your own models gives you control, but comes with infra headaches you need to plan for.
I’ve used vLLM
when inference speed was critical and Ollama
for edge deployment/testing. Hugging Face + transformers
still works, but can get heavy on GPU memory unless you’re quantizing or using LoRA.
LoRA vs Full Fine-Tuning (2025 Update)
Personally, I’ve stopped doing full fine-tunes unless I absolutely need to. Here’s how I approach it now:
Situation | What I Use |
---|---|
App requires narrow domain knowledge (e.g., legal, medicine) | LoRA with domain data |
App involves new task structure (e.g., document conversion to GraphQL) | Full fine-tune (if needed) |
Latency-sensitive, on-device scenario | QLoRA + int4 quantization |
Experimental or small footprint | Ollama with distilled models |
And don’t sleep on gguf
formats — I’ve had great success using llama.cpp
builds on edge devices for multimodal use cases.
Quick Example: vLLM Setup (Local API Serving)
pip install vllm
python -m vllm.entrypoints.openai.api_server \
--model facebook/opt-1.3b \
--tensor-parallel-size 1
Once it’s up, you can hit it with OpenAI-compatible clients:
import openai
openai.api_base = "http://localhost:8000/v1"
openai.api_key = "fake"
res = openai.ChatCompletion.create(
model="facebook/opt-1.3b",
messages=[{"role": "user", "content": "Explain LoRA in 2 sentences"}]
)
Fast, local, and surprisingly stable — if you can manage the memory.
This might surprise you: most of the performance gains I’ve seen in AI apps don’t come from switching models. They come from tighter prompt logic, better memory orchestration, and reducing waste in your token usage pipeline.
5. Context Injection / RAG Pipelines (If Applicable)
Let me be blunt: If your app needs to surface facts, support documents, or respond to domain-specific queries — and you’re not using RAG — you’re probably hallucinating 50% of the time. I’ve built several production apps around retrieval, and here’s what’s actually worked for me.
Chunking Strategy (This Isn’t Just Splitting Strings)
You might be tempted to split on paragraphs or sections, but I’ve personally seen better semantic coherence when using recursive chunking — especially when dealing with structured docs (Markdown, HTML, PDFs).
LangChain’s built-in RecursiveCharacterTextSplitter
works surprisingly well:
from langchain.text_splitter import RecursiveCharacterTextSplitter
splitter = RecursiveCharacterTextSplitter(
chunk_size=500,
chunk_overlap=100,
separators=["\n\n", "\n", ".", " ", ""]
)
chunks = splitter.split_text(long_document_text)
I’ve tuned this based on source format — Markdown docs usually need higher overlap to preserve heading context, whereas PDFs are cleaner with more aggressive splits.
Embedding Models That Actually Perform
You might be wondering: Aren’t all embeddings kind of the same?
Short answer: No. I’ve personally run benchmarks across OpenAI’s text-embedding-3-large
, instructor-xl
, and bge-large-en
, and here’s what I found:
text-embedding-3-large
: Fast, accurate, and pairs best with OpenAI completions.instructor-xl
: Performs significantly better on tasks like multi-hop QA and semantic search.bge-large-en
: Great balance of quality + self-hosting flexibility (I’ve served this viavLLM
and it held up).
For mission-critical projects, I use OpenAI’s embeddings in production and Instructor
for prototypes or academic-oriented RAG.
Vector DBs: What I Actually Use
Here’s the deal: you don’t need to over-engineer this part unless you’re indexing hundreds of millions of chunks.
Personally, I lean toward Qdrant or Weaviate for most projects. They’re dead simple to spin up via Docker and support hybrid search out of the box.
from langchain.vectorstores import Qdrant
from langchain.embeddings import OpenAIEmbeddings
from qdrant_client import QdrantClient
qdrant = QdrantClient(host="localhost", port=6333)
vectorstore = Qdrant.from_documents(
documents=chunks,
embedding=OpenAIEmbeddings(),
collection_name="docs",
client=qdrant
)
That’s it — and now your documents are indexed and ready for semantic retrieval.
Hybrid Search = Context That Actually Answers the Question
This might surprise you: pure vector similarity often fails for rare keywords or fuzzy phrasing.
That’s why I’ve started using hybrid search (BM25 + embeddings) for anything more than trivial lookup.
Weaviate supports this natively, but if you’re not using it, you can roll your own by merging scores from elasticsearch
and your vector DB. Yes, it’s more work — but the jump in relevance is worth it. Especially when users start asking edge-case queries like “Why did feature X crash in version 3.1?” or “What’s the compliance status of Y under GDPR?”
Evaluation: Don’t Guess Retrieval Quality
I learned this the hard way — just because your app returns text doesn’t mean it’s retrieving the right chunk.
I use RAGAS to benchmark:
- Precision (did the retrieved doc actually help answer?)
- Faithfulness (did the LLM hallucinate?)
- Context relevance (is the chunk on-topic or just noise?)
You don’t need this for every prototype — but if you’re building for users, especially enterprise ones, you’ll thank yourself later.
Bonus: LangChain RAG in One Line
If you want to get started quick (and you’re fine with some magic under the hood), here’s the fast path I sometimes use:
from langchain.chains import RetrievalQA
from langchain.chat_models import ChatOpenAI
qa_chain = RetrievalQA.from_chain_type(
llm=ChatOpenAI(),
retriever=vectorstore.as_retriever(),
chain_type="stuff"
)
response = qa_chain.run("What are the key ideas in the second section of the GDPR document?")
In my experience, this works great for 80% of use cases. The moment it breaks down (usually on multi-hop questions), I switch to map_reduce
or build a custom RAG chain with rerankers and confidence scores.
Want to make your RAG app reliable? Treat chunking, embedding, and evaluation as first-class citizens — not just pre-processing steps. That shift in mindset made a big difference for me.
6. Backend Implementation (FastAPI)
I’ve settled on FastAPI for most of my LLM backends. It’s async by default, integrates cleanly with streaming, and scales decently with uvicorn
and workers — no unnecessary fluff.
Async API Design
If you’re calling OpenAI, Anthropic, or even self-hosted models, you need async — or you’ll burn threads and hang under load. I always keep endpoints lean:
from fastapi import FastAPI, Request
app = FastAPI()
@app.post("/query")
async def query_llm(request: Request):
body = await request.json()
query = body.get("query")
# Call your model or RAG pipeline here
result = await call_llm(query)
return {"response": result}
You might be wondering: Do I really need async if my model call is already async?
Yes — because even with threads, you’ll hit connection pool limits fast. I learned that lesson during a hackathon stress test.
Streaming Responses (text/event-stream)
This part is a game-changer for UX — especially when token generation takes time. I’ve used Server-Sent Events (SSE) with FastAPI for this.
Here’s a minimal implementation I’ve used in production:
from fastapi.responses import StreamingResponse
import asyncio
@app.get("/stream")
async def stream_response(query: str):
async def token_stream():
async for chunk in stream_llm_response(query):
yield f"data: {chunk}\n\n"
await asyncio.sleep(0.05) # Optional pacing
return StreamingResponse(token_stream(), media_type="text/event-stream")
Pair this with a frontend that consumes SSE (I’ll show you that in the next section), and you’ve got a responsive, chat-like interface that feels native.
Rate-Limiting, Retries, Timeouts
If you’re deploying this for internal users or production apps, these become non-negotiable.
- Retries: Use
tenacity
with exponential backoff for LLM calls (especially OpenAI — you will hit 429s occasionally). - Timeouts: I wrap every outbound call with
asyncio.wait_for
to avoid hanging connections. - Rate-limiting: For FastAPI, I’ve integrated slowapi when needed.
from tenacity import retry, wait_random_exponential, stop_after_attempt
@retry(wait=wait_random_exponential(min=1, max=10), stop=stop_after_attempt(3))
async def call_llm(query: str) -> str:
# Insert your OpenAI or local model call here
...
Without this layer, even a small spike in usage can crash the whole thing — I’ve learned that the hard way.
7. Frontend (Optional but Useful)
I’ll keep this lean — but if you’re demoing to stakeholders or shipping to real users, a frontend that streams and responds instantly makes all the difference.
Minimal React Setup with Streaming
I usually go with React + fetch + Tailwind, or if I want a polished feel, I drop in shadcn/ui.
Here’s a basic React component I’ve used:
const handleQuery = async () => {
const response = await fetch("/stream?query=" + encodeURIComponent(query), {
headers: { Accept: "text/event-stream" },
});
const reader = response.body.getReader();
const decoder = new TextDecoder("utf-8");
while (true) {
const { value, done } = await reader.read();
if (done) break;
const chunk = decoder.decode(value);
setOutput(prev => prev + chunk.replace("data: ", ""));
}
};
Throw in a useEffect
with debounce, style it with Tailwind, and you’ve got a responsive AI assistant.
Why the Frontend Matters — Even for Data Scientists
You might be thinking, Why should I care about UI? I used to think the same.
But here’s what I’ve seen: when you’re demoing an LLM app — especially one with retrieval or agents — the UI either makes it feel magical or makes it feel broken. Streaming, debounce, and context indicators help users understand what the model’s doing. That’s crucial for trust.
In short: FastAPI + async + streaming + just enough UI = a dev-friendly stack that feels smooth in production.
8. Deployment in 2025: What Actually Works
If you’ve deployed models before, you’ll know: deployment can be a mess. Getting everything from dev to prod without chaos is an art — and you can learn from my mistakes here.
Dockerizing Everything (Backend + Optional Frontend)
I won’t sugarcoat it: Docker is a must. Every environment should be the same, and Docker guarantees that. Whether you’re building a backend API or a UI for your LLM app, you want to ensure consistency. I’ve spent way too much time debugging issues that were simply the result of different dependencies across environments.
Here’s a minimal Dockerfile I’ve used for FastAPI:
FROM python:3.10-slim
WORKDIR /app
COPY . .
RUN pip install -r requirements.txt
CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "8000"]
Hosting Options: Choosing the Right Platform
I’ve learned that your hosting choice really depends on the scale of your application.
- Frontend: I personally go with Vercel for frontend. It’s simple to set up, scales easily, and doesn’t require too much configuration. You might be wondering, Why not something like Netlify? Well, Vercel has been better for real-time streaming apps (like LLMs) in my experience.
- Backend: Render or Fly.io work great for small to medium apps. They’re easy to deploy with minimal setup. You’ll see a ton of “push-to-deploy” features, and it’s way less of a headache than managing EC2 instances, trust me.
- Heavy Pipelines: For highly demanding models or heavy computations, I use GCP or AWS. They give you the scale and GPU instances needed for production-level workloads. Just be ready for cost management — these platforms can get expensive if you don’t control your usage.
Load Testing Tips
I’ll be honest: I didn’t always do load testing, and my first public release didn’t end well. Here’s what I learned:
- Start with a small load test, then scale up. You don’t need to simulate 10,000 users on day one.
- Use tools like Locust or Artillery for API testing. I’ve found them to be super flexible and not a pain to set up.
- Monitor latency and throughput under load. If you’re using external APIs like OpenAI, expect variable latency.
Cost Control: Batching, Caching, and Usage Limits
I can’t stress this enough: keep your usage efficient. For LLMs, batching inputs and caching results can save you a lot of money, especially if you’re using an API-based model.
- Batching: I combine similar queries and run them in batches instead of hitting the model every single time.
- Caching: Cache frequent queries or use tools like Redis for quick retrieval.
- Usage Limits: Set strict limits on API calls to avoid runaway costs. Most API providers (like OpenAI) allow you to set hard limits on usage.
9. Observability, Logging, and Monitoring
Now, you’ve got your app running. Great. But how do you know it’s actually working? How do you troubleshoot when things go wrong?
Here’s what I’ve learned about observability:
Structured Logging
For logging, structured logs are essential. I use structlog
or loguru
to create logs that are easy to parse and read. With structured logging, you’re not just printing plain text; you’re tracking events, errors, and latency in a way that scales.
Example using loguru:
from loguru import logger
logger.add("app.log", rotation="500 MB") # Rotate logs after 500 MB
logger.info("LLM query processed", extra={"query": query, "status": "success"})
You might be wondering: Why not use just print
or logging
?
I’ve tried both, but structured logs are a godsend when you need to filter, analyze, or send logs to a monitoring service.
Latency, Token Usage, Error Tracking
To track latency and token usage, you’ll want to set up tools like Sentry and PostHog. Both are great at giving you insights into errors, slow responses, and usage trends.
For latency tracking, I’ve used this with Sentry:
import sentry_sdk
sentry_sdk.init("your_sentry_dsn")
with sentry_sdk.start_transaction(op="task", name="LLM query"):
result = call_llm(query)
sentry_sdk.set_transaction_name("LLM query")
Prompt Logging + Feedback Loop
One thing that changed the game for me was tracking prompt usage and building a feedback loop for prompt engineering. By logging and analyzing how prompts perform, I’ve been able to fine-tune them and optimize LLM outputs.
Here’s a minimal way I log prompts:
def log_prompt(prompt, result):
logger.info("Prompt executed", extra={"prompt": prompt, "result_length": len(result)})
Then, I use that log to analyze patterns in which prompts work best and which fail. This is crucial for improving response quality, and I can tell you from experience that it saves a lot of trial and error.
In the end, deployment isn’t just about putting code in the cloud. It’s about scalability, resilience, and observability. With Docker, good hosting choices, and proper monitoring, you can ensure that your app doesn’t just run — it runs reliably.
Final Thoughts: From Tutorial to Production
You’ve made it this far — you’ve built the logic, integrated APIs, designed your backend, and prepared for deployment. But now comes the most important part: taking your app from a tutorial project to a real-world production app.
In my experience, going from concept to production is where most data scientists, especially those new to deployment, hit a wall. The theory is easy; it’s the real-world challenges — scaling, handling errors, managing resources — that are tough. But trust me, you can do it.
I’d encourage you to take this guide and build something meaningful. Don’t just stop here. Sure, this is a starter template, but you’ve got the tools and patterns to go much further. Use this as a launchpad, and soon you’ll have an app that can handle real-world traffic, provide value, and make an impact.
The Key to Success
- Iterate: Start small, and keep iterating. Your first deployment won’t be perfect, and that’s completely fine. I’ve learned that failing early and getting feedback is critical.
- Monitor: Keep a close eye on your logs, track latency, and watch usage patterns. Don’t wait until things break.
- Scale Gradually: You don’t need to overcomplicate things on day one. Use the tools at your disposal and add complexity as needed.
And one last thing: Don’t rush. Quality deployment takes time, and it’s better to do it right the first time than to scramble later when things fall apart.
Starter Template
To help you get started, I’ve put together a GitHub repo with all the code snippets from this guide, plus a starter template that you can clone and modify to fit your project. You’ll find the Dockerfiles, backend FastAPI setup, frontend templates, and deployment instructions.
Here’s a quick start to get you on your way:
- Clone the repository:
git clone https://github.com/yourusername/llm-app-template.git
cd llm-app-template
2. Install dependencies:
pip install -r requirements.txt
3. Build and run your app locally:
docker-compose up --build
You’re now ready to deploy, monitor, and scale your app in production.
Good luck, and don’t hesitate to reach out if you hit any bumps — I’ve been there, and I know how frustrating deployment can be. But with patience and persistence, you’ll get your app running smoothly and efficiently.
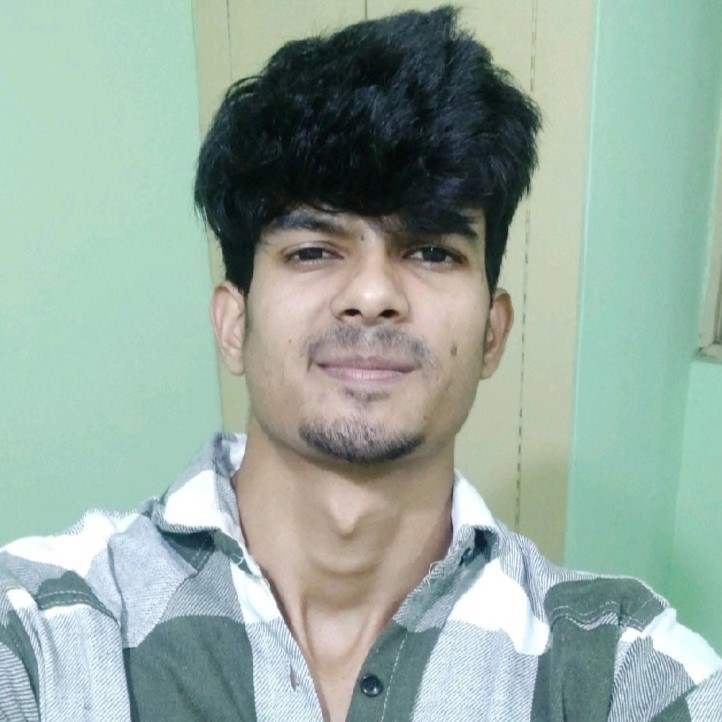
I’m a Data Scientist.