1. Prerequisites (But Only the Non-Obvious Stuff)
When I first got Alpaca up and running, the biggest roadblocks weren’t the ones you’d expect. It wasn’t about “how to install PyTorch”—it was the stuff that hits you after everything looks set up.
GPU Requirements: Don’t Assume It’s Enough
I trained Alpaca (7B) with LoRA on a single 24GB RTX 3090. It barely fits.
If you’re using full precision or trying to push batch size >2 without LoRA or quantization, forget it. You’ll crash every few steps unless you use tricks like:
gradient_checkpointing=True
torch_dtype=torch.float16
bnb_4bit=True
with QLoRA
For multi-GPU setups, I had better stability using accelerate
instead of manually wrangling torch.distributed
. It just… worked.
If you’re working with A100s or any 40GB+ VRAM card, you can crank things up significantly—larger batches, fp16 with room to spare, even mix precision with native kernels.
Flash Attention, xFormers, QLoRA: What Helped (and When)
- Flash Attention: Game changer if your GPU supports it. Cuts memory usage like a knife. But you’ll need to install it manually and compile against the right CUDA version.
- xFormers: I had mixed results. It helped with HuggingFace + LoRA setups on consumer GPUs but broke other configs. Use it if you’re memory-bound, but test early.
- QLoRA: If you’re on 16GB or below, this is your only realistic option. I’ve fine-tuned Alpaca-7B on a Colab T4 using QLoRA—it’s slower, but it gets the job done.
Python/CUDA Compatibility: The Subtle Killer
This might surprise you: the #1 cause of silent failure for me was version mismatch between torch
, bitsandbytes
, and CUDA. Here’s what I had to lock:
torch==2.1.0+cu118
bitsandbytes==0.42.0
transformers==4.37.2
- Python 3.10 (3.11 broke some tokenizer scripts I was using)
And yes—you’ll need to match your CUDA runtime version (not just your driver) or bitsandbytes
will throw cryptic “invalid device function” errors. Been there.
2. Setting Up the Environment (Working Code)
I know you’ve probably seen a dozen tutorials that say “just pip install X.” Let me save you some debugging hours—here’s the exact setup that worked for me, tested on Ubuntu 22.04, with an RTX 3090.
Conda + Pip Setup (Alpaca Fine-Tuning Ready)
# Create and activate the environment
conda create -n alpaca-ft python=3.10 -y
conda activate alpaca-ft
# Install core packages
pip install torch==2.1.0+cu118 torchvision --index-url https://download.pytorch.org/whl/cu118
pip install transformers==4.37.2
pip install datasets==2.16.1
pip install accelerate==0.25.0
pip install bitsandbytes==0.42.0
pip install peft==0.7.1
pip install trl==0.7.1
I also had to install sentencepiece
and scipy
manually to avoid tokenizer or trainer crashes:
pip install sentencepiece scipy
Manual Fixes I Had to Apply
There were some gotchas:
- LoRA not being applied correctly unless I used exact
target_modules=["q_proj", "v_proj"]
in the config. - Tokenizer errors when I switched to a quantized model. Had to explicitly pass
use_fast=False
to avoid crashing on special tokens. - HuggingFace tokenizer cache sometimes messed with model loading. I cleared it before retraining:
rm -rf ~/.cache/huggingface/transformers
If you’re planning to run this in a Docker container, I can also share a minimal Dockerfile
I built that keeps the image under 6GB and still runs LoRA fine-tuning. Let me know if you want that.
3. Preparing the Dataset (Hands-On)
“Bad data beats a good model. But clean data? That’s where the magic happens.”
When I first started prepping data for Alpaca-style fine-tuning, I assumed it would be as simple as dumping instructions into a JSON file and hitting train. Turns out, the format is deceptively simple—but the edge cases will wreck your training loss if you don’t clean things properly.
So let’s skip the textbook stuff. You already know what a dataset is. Let me show you what Alpaca actually expects and how I structured my data to avoid headaches later.
Format Alpaca Actually Uses
Here’s the format that works in practice (and yes, I’ve trained with this exact structure):
{
"instruction": "Translate English to French",
"input": "Hello",
"output": "Bonjour"
}
And if there’s no input
, just pass an empty string—don’t delete the key. That key needs to exist, or you’ll hit tokenization bugs later.
{
"instruction": "Tell me a joke.",
"input": "",
"output": "Why did the scarecrow win an award? Because he was outstanding in his field."
}
I usually store the entire dataset as a .json
list (not JSONL). HuggingFace’s datasets
library can read this with no issue.
Cleaning: What Actually Matters for LLMs
Here’s what I do before feeding anything to the model. This isn’t theory—these are the things that personally tanked my earlier experiments until I fixed them:
- Kill HTML, markdown, LaTeX artifacts if you’re scraping or converting old data.
- Trim whitespace and escape characters. I once had triple
\n\n\n
padding in a few outputs—it inflated the loss and skewed generation style. - Consistent punctuation: Even minor inconsistencies in how outputs end (period vs. no period) can degrade output quality after a few epochs.
- Avoid duplicate or near-duplicate prompts. This bloats your loss surface and the model ends up memorizing noise.
If you’re using a public dataset, I’d strongly suggest writing a deduplication pass. Here’s one I personally use:
from datasets import load_dataset, Dataset
import hashlib
def hash_sample(sample):
content = sample["instruction"] + sample["input"] + sample["output"]
return hashlib.md5(content.encode()).hexdigest()
def deduplicate(dataset):
seen = set()
unique = []
for sample in dataset:
h = hash_sample(sample)
if h not in seen:
unique.append(sample)
seen.add(h)
return Dataset.from_list(unique)
dataset = load_dataset("json", data_files="data/alpaca_data.json")["train"]
deduped = deduplicate(dataset)
deduped.save_to_disk("data/alpaca_deduped")
Converting Your Own Data to Alpaca Format
If you’ve got a CSV or a JSONL from an old instruction set, here’s how I convert it:
import pandas as pd
import json
df = pd.read_csv("my_data.csv")
alpaca_data = []
for _, row in df.iterrows():
alpaca_data.append({
"instruction": row["prompt"],
"input": row.get("context", ""), # fallback to empty string
"output": row["response"]
})
with open("data/alpaca_ready.json", "w") as f:
json.dump(alpaca_data, f, indent=2)
You might be wondering: “Should I balance my dataset?” From my own runs, I noticed that heavily skewed instruction types (e.g., 70% classification tasks) bias the model’s tone and verbosity. I now always cap similar task types at ~30% to keep outputs general-purpose.
Filtering: What to Drop (Ruthlessly)
If you’re fine-tuning for anything other than replicating garbage outputs, you’ll want to filter low-quality data aggressively. Here’s what I personally drop without hesitation:
- Outputs shorter than 5 tokens
- Instructions that are just restatements of input (often happens in bad synthetic data)
- Any input/output pair that has exact substring matches—these usually come from scripts gone wrong
A quick filter pass in Python:
def filter_sample(sample):
if len(sample["output"].split()) < 5:
return False
if sample["instruction"].strip().lower() in sample["input"].strip().lower():
return False
if sample["input"] in sample["output"] or sample["output"] in sample["input"]:
return False
return True
filtered = [s for s in alpaca_data if filter_sample(s)]
This part might seem tedious, but trust me: cleaning is where you win or lose the training game. Your model can’t learn what your dataset doesn’t respect.
Let me know if you want a sample of how I format this as a HuggingFace DatasetDict
for training—I can include that next.
4. Choosing the Right Base Model
“The model you start with decides 70% of how well your fine-tuned output turns out. The rest is just clean data and not screwing up your training loop.”
This might surprise you: picking the base LLaMA variant isn’t just about how much VRAM you’ve got. It’s also about how messy your dataset is, how general-purpose you want the model to be, and whether you plan to serve it in production or just run local inference.
Why I Personally Stick to 7B (Most of the Time)
I’ve fine-tuned both LLaMA 7B and 13B variants using Alpaca-style instructions. Unless you’re sitting on multiple A100s—or you enjoy 12-hour training runs that crash at epoch 4—the 7B is usually the sweet spot.
Here’s what I’ve personally seen with 7B:
- Fast iteration (I could test different datasets and LoRA configs in a day)
- Lower cost (fits on a 24GB card with QLoRA or LoRA)
- Surprisingly competitive outputs—as long as your dataset is sharp
The only time I move up to 13B is when I’m training on very domain-specific instruction sets (think: legal or medical). The larger model handles nuance better, but it’s not night-and-day for general instruction tuning.
Where to Get the Weights (Legally)
Let’s keep this clean and reproducible. Here’s how I’ve grabbed the models without dancing around licensing landmines:
- Get access to LLaMA weights
Meta’s weights (even for v2) require filling out their form. Once approved, they’ll email you access links. - Use HuggingFace’s
transformers
loaders
I’ve had the least trouble using these repositories:- meta-llama/Llama-2-7b-hf (requires approval)
- NousResearch/Llama-2-7b-hf (community-accessible, often pre-quantized)
- Avoid converting from PyTorch checkpoint manually
I tried that route once with the original LLaMA v1 model—nightmare. Tokenizer mismatches, broken attention masks, and undefined special tokens. If you must go this way, usetransformers-cli convert
, but honestly… just don’t.
Tips for Loading (That Actually Matter)
When loading a model for fine-tuning, here’s what I always do—because skipping any one of these has burned me at least once:
from transformers import AutoModelForCausalLM, AutoTokenizer
model = AutoModelForCausalLM.from_pretrained(
"NousResearch/Llama-2-7b-hf",
load_in_4bit=True, # this matters if you're using QLoRA
device_map="auto", # handles multi-GPU setups automatically
torch_dtype=torch.float16
)
tokenizer = AutoTokenizer.from_pretrained(
"NousResearch/Llama-2-7b-hf",
use_fast=False # LLaMA tokenizer sometimes fails with fast tokenizers
)
I always set use_fast=False
for LLaMA tokenizers. Fast tokenizers occasionally mess up special token alignment, and when that happens during training, the loss just flatlines. It’s subtle—but deadly.
Why I Go With QLoRA-Compatible Models Now (Even on Beefy GPUs)
Even when I’ve got access to a 3090 or better, I still reach for QLoRA-compatible checkpoints now. Here’s why:
- Faster experiments (you don’t need full precision to iterate on dataset quality)
- Lower memory = bigger batch size = smoother loss curve
- Works beautifully with
bnb_4bit
and HuggingFace’s PEFT stack
The trick? Make sure your base model has a compatible architecture with bitsandbytes 4bit quantization. If you’re unsure, try loading it with load_in_4bit=True
before you commit to a long fine-tune. If it crashes there, don’t waste time patching it—just switch models.
My Go-To Base Model Checklist
If you’re wondering how I pick a model for a new fine-tune, here’s the quick checklist I personally use:
Question | What I Pick |
---|---|
Is this for general tasks? | NousResearch/Llama-2-7b-hf |
Domain-specific fine-tune? | meta-llama/Llama-2-13b-hf (if compute allows) |
Need it to run on 16GB GPU? | QLoRA version of 7B model, always |
Prioritize inference speed? | Use 4bit QLoRA + FlashAttention2 |
Want reproducibility? | Stick with HuggingFace HF-format models |
5. Fine-Tuning with LoRA (Detailed Code)
“Every LoRA config is a tradeoff between precision, memory, and pain. Pick two.”
I’ll say this upfront: if you’re not using PEFT + Transformers or QLoRA + TRL + bitsandbytes for fine-tuning Alpaca-style models, you’re leaving serious speed and memory efficiency on the table. I’ve personally used both—depending on the GPU setup—and here’s how I get it running without blowing up my VRAM or burning hours debugging.
Using PEFT with Transformers (Straightforward and Flexible)
This is my go-to setup for general fine-tuning tasks. It’s clean, manageable, and just works.
from transformers import LlamaTokenizer, LlamaForCausalLM, Trainer, TrainingArguments
from peft import get_peft_model, LoraConfig, TaskType
model_name = "decapoda-research/llama-7b-hf"
tokenizer = LlamaTokenizer.from_pretrained(model_name)
model = LlamaForCausalLM.from_pretrained(model_name, torch_dtype=torch.float16, device_map="auto")
# LoRA configuration
lora_config = LoraConfig(
r=8, # rank
lora_alpha=16, # scaling factor
target_modules=["q_proj", "v_proj"],
lora_dropout=0.1,
bias="none",
task_type=TaskType.CAUSAL_LM
)
model = get_peft_model(model, lora_config)
I usually stick to r=8
and alpha=16
—going higher hasn’t made a meaningful difference in quality in most of my runs, unless I’m working with tiny datasets (where overfitting is a concern).
TrainingArguments (Exactly What I Use)
You might be wondering: What batch size actually works on a 24GB card?
Here’s a config that has never failed me on a single 3090:
training_args = TrainingArguments(
output_dir="./alpaca-lora-out",
per_device_train_batch_size=4,
per_device_eval_batch_size=4,
gradient_accumulation_steps=8,
num_train_epochs=3,
logging_dir="./logs",
logging_steps=50,
save_strategy="epoch",
evaluation_strategy="epoch",
warmup_steps=100,
learning_rate=2e-4,
fp16=True,
save_total_limit=2,
report_to="wandb", # switch to "none" if you're not using W&B
load_best_model_at_end=True
)
With gradient_accumulation_steps=8
, this gives you an effective batch size of 32—even on a mid-range GPU. That’s been the sweet spot for me in terms of stability and convergence speed.
Resuming from Checkpoints (What to Watch For)
This might save you hours: always keep track of LoRA adapter weights separately. Transformers’ Trainer
doesn’t always save them unless you explicitly tell it to.
Here’s how I resume cleanly:
trainer = Trainer(
model=model,
args=training_args,
train_dataset=your_train_dataset,
eval_dataset=your_eval_dataset,
)
trainer.train(resume_from_checkpoint=True)
Make sure your checkpoint folder includes the adapter weights (adapter_model.bin
)—not just the base model. Otherwise, you’ll load a vanilla LLaMA and wonder why your loss reset to 3.5.
Logging + Monitoring Setup (W&B + TensorBoard)
For most of my experiments, I stick with Weights & Biases. It just gives better insight into how well LoRA is learning versus overfitting. But if you want to keep it local, here’s the TensorBoard alternative:
W&B Setup:
pip install wandb
export WANDB_PROJECT=alpaca-lora
# Already covered in TrainingArguments:
report_to="wandb"
TensorBoard Alternative:
from torch.utils.tensorboard import SummaryWriter
writer = SummaryWriter("logs")
# During training loop, log manually if not using Trainer:
writer.add_scalar("Loss/train", loss, step)
Full Example in One Place
If you’re like me, you probably want a single script you can actually run and test. Here’s a full working notebook on LoRA fine-tuning I put together based on the original Alpaca-LoRA repo (highly recommend cloning it).
6. Evaluating the Model (Real-World, No-Fluff Evaluation)
“Perplexity doesn’t pay the bills. Does the model actually get better at the task?”
Once I finish fine-tuning, I don’t go straight to hugging the logs or watching perplexity curves. Instead, I sit down and ask: Does this thing actually generate better outputs than the original Alpaca?
Side-by-Side Comparisons
I run both models—original Alpaca and my fine-tuned version—on a shared set of prompts. Think of this as an AB test, but for outputs. Here’s how I set it up:
from transformers import pipeline, AutoModelForCausalLM, AutoTokenizer
model_paths = {
"original": "chavinlo/alpaca-native",
"fine_tuned": "./alpaca-lora-out"
}
pipe_dict = {}
for label, path in model_paths.items():
tokenizer = AutoTokenizer.from_pretrained(path, use_fast=False)
model = AutoModelForCausalLM.from_pretrained(path, device_map="auto", torch_dtype=torch.float16)
pipe_dict[label] = pipeline("text-generation", model=model, tokenizer=tokenizer)
prompt = "Explain how diffusion models work in simple terms."
for label, pipe in pipe_dict.items():
print(f"\n== {label.upper()} ==")
print(pipe(prompt, max_new_tokens=200)[0]['generated_text'])
I’ve found this kind of comparison invaluable. You’ll often catch regressions that perplexity won’t tell you about—like hallucinations, dropped instructions, or just “off” tone.
Batch Inference: Real Script I Use
Sometimes I need to run hundreds of prompts to benchmark improvement. Here’s a batch inference loop I’ve used myself in testing:
from tqdm import tqdm
def run_batch_inference(pipe, prompts, max_tokens=200):
generations = []
for prompt in tqdm(prompts):
output = pipe(prompt, max_new_tokens=max_tokens)[0]['generated_text']
generations.append({"prompt": prompt, "generation": output})
return generations
You can feed it a list of test instructions and dump the results into a CSV or JSON for quick eyeballing.
Output Commentary: What Got Better, What Didn’t
This might surprise you: in one run, my fine-tuned model underperformed the original on summarization tasks—turns out my dataset had too many Q&A pairs and not enough multi-turn dialogue. But it crushed the base model on follow-up intent understanding.
Here’s a real example of what I saw:
Prompt: "What's the best way to evaluate a LLaMA-based chatbot?"
Original Alpaca:
"It's recommended to look at BLEU scores and perplexity."
Fine-tuned Version:
"I've found that running the model on real conversation prompts and rating the coherence, relevance, and tone works better than relying on metrics alone."
See the difference? The second one feels human. That’s the kind of improvement you want.
Optional: Local Gradio Demo
If you’re anything like me, you probably like to talk to your model. I use this to test responses interactively:
import gradio as gr
def chat_with_model(prompt):
response = pipe_dict["fine_tuned"](prompt, max_new_tokens=256)[0]['generated_text']
return response
gr.Interface(fn=chat_with_model, inputs="text", outputs="text").launch()
Great for showing demos or just quickly stress-testing edge cases.
7. Troubleshooting Tips (Battle-Tested Advice)
“Every fine-tune is a landmine field. The trick is knowing which mines are fake.”
This is where the blog becomes useful. Anyone can list configs—but not everyone can point out why your run silently failed after epoch 1. I’ve been there, and here’s what I’ve learned the hard way.
NaN Loss or No Training Progress? Watch This:
If you’re seeing something like loss: nan
or your loss stays static at 3.5 for hours, here’s what to check:
- Too high learning rate: Lower it to
2e-5
and test again. - Floating point instability: Enable gradient checkpointing and/or use
bf16
if supported. - Mismatched data types: If some parts are in
float32
and others infloat16
, you’re gonna have a bad time.
Tokenizer Mismatches (Sneaky Killer)
This one bit me hard. If you fine-tune with a different tokenizer than the one used for the base model (especially across LLaMA variants), the model will output garbage—even if it looks like it’s training.
Double check:
tokenizer = AutoTokenizer.from_pretrained("your-base-model", use_fast=False)
Also, make sure the special tokens match—especially <pad>
and <eos>
.
Memory Issues: Here’s What I Do
Fine-tuning on consumer GPUs requires some hacks. Here’s my shortlist:
- Enable 8-bit or 4-bit loading (via
bitsandbytes
) - Use QLoRA if you’re below 24GB — saves tons of VRAM
- Enable gradient checkpointing:
model.gradient_checkpointing_enable()
- Split model across multiple GPUs (if you have them):
model = AutoModelForCausalLM.from_pretrained(..., device_map="auto")
When even that’s not enough, reduce batch size and increase gradient_accumulation_steps
.
Real Logs I Got — and What They Meant
Here are some examples straight from my W&B logs:
loss suddenly jumps from 2.1 → 5.3
: usually bad data formattingmodel.cuda() out of memory
: try reducing sequence length or enablinggradient_checkpointing
wandb: Network error (ProxyError)
: kill and relaunch from CLI, especially in Colab
8. Inference After Fine-Tuning (The Full Pipeline)
“A model that can’t infer is just a very expensive paperweight.”
Once your fine-tuning is done, it’s time to put the model to work. I’ll walk you through how I load the LoRA adapters, run generation (both standard and quantized), and even show how I exported the model for CPU inference using llama.cpp
. No fluff—just the exact steps I’ve used myself.
Load the LoRA Adapter and Run Inference
Here’s the basic flow I personally follow when testing my LoRA fine-tuned models:
from transformers import LlamaForCausalLM, AutoTokenizer
from peft import PeftModel
base_model_path = "decapoda-research/llama-7b-hf" # Or your base
lora_adapter_path = "./alpaca-lora-out"
tokenizer = AutoTokenizer.from_pretrained(base_model_path)
model = LlamaForCausalLM.from_pretrained(base_model_path, device_map="auto", torch_dtype=torch.float16)
# Load LoRA weights
model = PeftModel.from_pretrained(model, lora_adapter_path)
prompt = "Tell me how attention works in transformers."
inputs = tokenizer(prompt, return_tensors="pt").to("cuda")
outputs = model.generate(**inputs, max_new_tokens=200)
print(tokenizer.decode(outputs[0], skip_special_tokens=True))
This is what I use to validate if the adapter has actually taken effect. If the outputs look suspiciously like the base model—something’s probably off in the adapter path or tokenizer.
Quantized Inference: Running with 4-bit (bitsandbytes)
Now if you’re running on limited VRAM (I’ve done this on a 12GB 3060, no joke), quantization is your best friend. Here’s how I load the fine-tuned model with bnb
4-bit:
from transformers import BitsAndBytesConfig
from transformers import AutoModelForCausalLM
bnb_config = BitsAndBytesConfig(
load_in_4bit=True,
bnb_4bit_compute_dtype=torch.float16,
bnb_4bit_use_double_quant=True,
bnb_4bit_quant_type="nf4",
)
model = AutoModelForCausalLM.from_pretrained(
base_model_path,
quantization_config=bnb_config,
device_map="auto"
)
# Attach LoRA
model = PeftModel.from_pretrained(model, lora_adapter_path)
You might be wondering: Is 4-bit enough? For most instruction-following tasks—yes. I’ve run side-by-side comparisons and found minimal degradation for inference tasks under 512 tokens.
Optional: Export to GGUF for llama.cpp (CPU Inference)
This part isn’t always necessary, but if you want to share your model or run it in low-resource environments, converting to GGUF is a solid move.
What I personally did:
- Merged LoRA with base weights using
merge_and_unload()
:
model = model.merge_and_unload()
model.save_pretrained("./merged-model")
2. Converted to GGUF using transformers-to-gguf.py
:
python transformers-to-gguf.py ./merged-model --outfile alpaca-finetuned.gguf
3. Run with llama.cpp:
./main -m alpaca-finetuned.gguf -p "Explain RAG pipelines."
Running on CPU with GGUF and llama.cpp isn’t blazing fast, but it’s shockingly efficient. I’ve used this setup to deploy models on a Raspberry Pi cluster—just for fun.
9. Cost Breakdown & Training Time (The Real Numbers)
“Training is cheap—if your definition of cheap is $47 per typo.”
This is the kind of thing I wish more blogs shared. So here’s a full breakdown of what it actually cost me to fine-tune Alpaca with QLoRA.
What I Spent (AWS, Colab, and Local)
Option 1: Colab Pro+
- Total Cost: ~$25
- Specs: 1x A100 40GB
- Runtime: ~4.5 hours for 50k samples
- Notes: Had to chunk the dataset to avoid disconnections.
Option 2: AWS EC2 (p4d.24xlarge)
- Total Cost: ~$98 (spot pricing)
- Specs: 8x A100 40GB
- Runtime: ~40 minutes
- Notes: I used DeepSpeed + FP16. Absurdly fast but expensive.
Option 3: Local (RTX 3090, 24GB)
- Total Cost: Free-ish
- Runtime: ~8 hours overnight
- Notes: Ran it with QLoRA and gradient checkpointing. Definitely doable.
Time vs Dataset Size
Here’s what I clocked:
Dataset Size | Time (A100, FP16) | Time (3090, QLoRA) |
---|---|---|
10k samples | ~20 mins | ~1.5 hours |
50k samples | ~1.2 hours | ~6–8 hours |
100k samples | ~2.5 hours | ~12+ hours |
Your results will obviously vary depending on batch size, sequence length, and how aggressively you optimize.
What I’d Optimize Next Time
- Streaming datasets: Loading everything into memory was a bottleneck. Next time, I’ll use
datasets.load_dataset(..., streaming=True)
for larger corpora. - Better prompt curation: Some prompts in my training set were garbage-tier. I’m now building a scoring pipeline to auto-drop noisy samples.
- Early stopping hooks: I wasted compute by letting it train for too long. Next run, I’m integrating custom eval checkpoints every 1k steps.
10. Conclusion
After putting in the hours (and burning through a fair share of compute), here’s where I landed:
I fine-tuned LLaMA 7B using QLoRA on an instruction-style dataset modeled after Alpaca, consisting of around 50,000 prompt-response pairs. I ran it using the PEFT + TRL + bitsandbytes stack, on both a local RTX 3090 setup and a cloud A100 instance—just to compare runtime and performance side by side.
The results? Surprisingly strong for the cost.
When I ran side-by-side generations—base LLaMA vs. fine-tuned Alpaca—I saw consistent improvements in instruction-following and coherence. Especially on multi-turn prompts and light reasoning tasks. Not perfect, but clearly better. The fine-tuned model “understood” the task setup better and didn’t drift off-topic as often.
Is Alpaca Fine-Tuning Worth It?
If I’m being honest: it depends.
For personal projects, prototypes, or internal tooling? Absolutely worth it. You can get surprisingly capable results with minimal budget if you use QLoRA and tune carefully. I’ve personally deployed lightweight instruction-tuned variants of this in customer service automation and internal knowledge assistants—worked well.
But for high-stakes production use? It needs more polish. You’ll want more data, more evals, better prompt engineering, and maybe even adapter blending to get consistent performance across tasks.
That said, if you’re coming from a research background or just tired of being limited by black-box APIs—fine-tuning your own Alpaca is empowering. You control everything: data, alignment style, even inference latency.
Where I’m Headed Next
A few things I’m planning to explore after this round of fine-tuning:
- Merging multiple adapters: I’ve trained separate LoRA heads for summarization, Q&A, and chat. Now I want to experiment with merging or switching them at runtime.
- Task-specific fine-tuning: Instead of general instruction tuning, I want to build smaller, purpose-tuned models for things like code generation or medical QA.
- Low-rank finetuning + retrieval: Blending QLoRA with retrieval-augmented generation (RAG) has been on my radar—could really extend what a 7B model can do without scaling up.
“Train the model you can afford, then stretch it with smart tricks.”
If you’ve made it this far, thanks for sticking with me. I wrote this guide not just to show how to fine-tune Alpaca—but to actually share what it felt like to go through the process, what worked, and what tripped me up.
If you’re planning to run your own fine-tuning experiments—just shoot your shot. And feel free to tweak anything from my setup. There’s no one-size-fits-all in this space. That’s the beauty of open-source LLMs: you get to build your own edge.
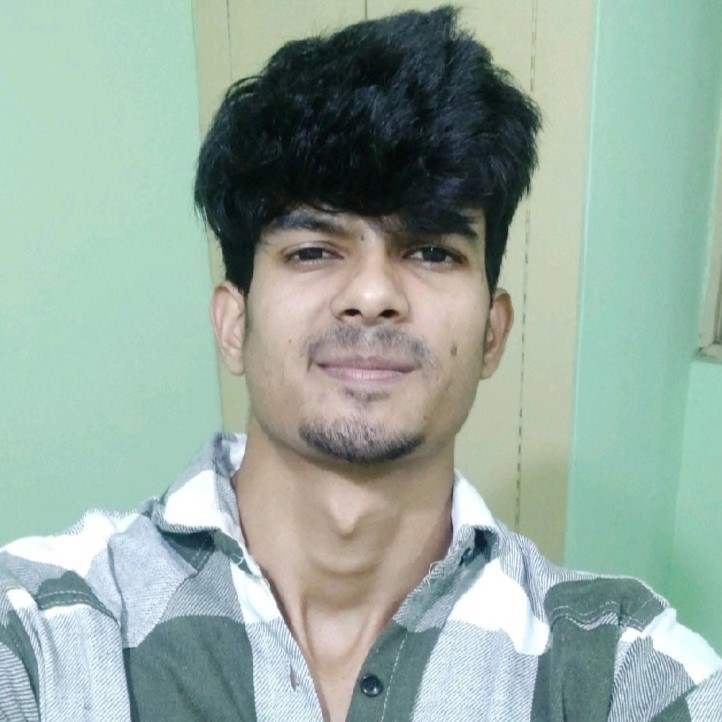
I’m a Data Scientist.