Introduction
“The real problem is not whether machines think, but whether men do.” – B. F. Skinner
Machine learning models are getting smarter every day, but let’s be honest—most of them still feel like black boxes. You build a model, train it on data, get impressive accuracy, and deploy it. But then someone asks, “Why did the model make this prediction?”—and suddenly, you’re stuck.
I’ve been there myself. Early in my career, I focused purely on improving model performance—higher accuracy, lower loss, better precision-recall. But I quickly realized that a great model isn’t just about numbers; it’s about trust. If you can’t explain why your model made a decision, no one—neither your stakeholders nor regulators—will take it seriously.
The Challenge: Interpretability in Machine Learning
Understanding what a model predicts is easy. Understanding why it makes those predictions? That’s the hard part. Traditional feature importance methods, like permutation importance, give some insights but often miss the bigger picture. They don’t consider feature interactions, and their results can be misleading—especially in complex models like deep learning or boosted trees.
The Solution: SHAP Values
That’s where SHAP (SHapley Additive exPlanations) values come in. They provide a fair, consistent, and mathematically sound way to break down any prediction into individual feature contributions.
I remember the first time I used SHAP on a real-world project—it was like turning on the lights in a dark room. Suddenly, I could see exactly how each feature influenced the model’s decision—no more guesswork, just clear, interpretable explanations.
What You’ll Learn in This Guide
In this deep dive, I’ll walk you through:
✅ What SHAP values really mean (beyond the textbook definition)
✅ How SHAP works under the hood (without unnecessary theory)
✅ How to apply SHAP in Python with real-world examples
✅ Common challenges when using SHAP (and how to solve them)
✅ Best practices for using SHAP in production (so you don’t make costly mistakes)
By the end of this guide, you won’t just understand SHAP values—you’ll be able to use them effectively in your own machine learning projects.
Let’s get started.
2. Understanding the Core Concept of SHAP Values
What Are SHAP Values?
“If you can’t explain it simply, you don’t understand it well enough.” – Albert Einstein
Interpreting machine learning models isn’t just a nice-to-have—it’s a necessity. I learned this the hard way when I deployed one of my first predictive models for a business use case. The accuracy was great, but when stakeholders asked “Why did the model make this decision?”—I had no clear answer. Feature importance charts? Too vague. LIME? Inconsistent results. That’s when I discovered SHAP (SHapley Additive exPlanations)—and it changed the way I approached model explainability.
SHAP is based on a concept from cooperative game theory, which ensures that each feature’s contribution to a prediction is fairly distributed. Unlike traditional feature importance methods that can be misleading, SHAP provides consistent, mathematically sound explanations.
Why SHAP is Better Than Other Interpretability Methods
You might be wondering: Why not just use feature importance from tree-based models or LIME? Well, I’ve tried them all, and here’s what I’ve learned:
- Permutation-based Feature Importance (like in Scikit-learn) tells you which features are important on average, but it doesn’t explain how they impact individual predictions.
- LIME works well for simple models, but it’s unstable—two similar inputs can give very different explanations.
- Partial Dependence Plots show trends but ignore interactions between features.
SHAP, on the other hand, fixes all these issues. It doesn’t just tell you which features matter—it shows how much they contribute and in which direction. That’s a game-changer when debugging models or making decisions based on AI outputs.
Mathematical Intuition Behind SHAP
Now, let’s get into the real magic behind SHAP. If you’ve ever split a restaurant bill among friends, you already understand the foundation of SHAP values.
Shapley Values in Game Theory: The Foundation of SHAP
Imagine you and your friends go out to dinner. Each person orders different items, but when the bill arrives, someone suggests splitting it fairly based on who contributed what to the total cost.
This is exactly how Shapley values work in game theory—except instead of people, we have features, and instead of a bill, we have a model prediction. The goal? Distribute the model’s output fairly among all the features that contributed to it.
Mathematically, Shapley values calculate a feature’s contribution by considering all possible permutations of features and averaging their marginal contributions. In simple terms:
- If a feature is crucial for a prediction, it gets a higher SHAP value.
- If a feature has little impact, its SHAP value is close to zero.
- If a feature decreases the prediction, its SHAP value is negative.
Why SHAP’s Additive Property Makes It So Powerful
One of the best things about SHAP is its additivity—meaning the sum of all SHAP values for a prediction equals the model’s output.
I remember using SHAP on a credit risk model, and seeing this principle in action was eye-opening. The SHAP values for income, credit score, and debt-to-income ratio all added up perfectly to the model’s predicted probability of default. It was like having a perfect breakdown of why the model made its decision—which is exactly what you need when explaining models to non-technical stakeholders.
Final Thoughts on SHAP’s Core Concept
SHAP isn’t just another interpretability tool—it’s the gold standard when you need trustworthy, consistent, and actionable explanations. If you’ve ever struggled to explain your model’s predictions, SHAP will completely change the way you approach interpretability.
Next, let’s see how SHAP actually works in different types of machine learning models—because theory is great, but hands-on experience is where it all comes together. 🚀
3. How SHAP Works in Machine Learning Models
By now, you know that SHAP values help explain why a model makes a particular decision. But you might be wondering: How does SHAP actually calculate these explanations?
The first time I used SHAP, I expected it to work like standard feature importance—just ranking features from most to least important. But SHAP goes way beyond that. It doesn’t just tell you which features matter; it quantifies how much each feature pushes a prediction up or down.
Let’s break this down step by step.
The SHAP Framework
SHAP values follow a simple but powerful idea: every prediction is the sum of individual feature contributions, plus a baseline expectation.
- Baseline (Expected Value): What the model would predict if it had no additional information (think of it as an average prediction).
- SHAP Values: How much each feature moves the prediction away from the baseline.
For example, imagine a model predicting whether a customer will default on a loan. If the average default probability across all customers is 10%, but for a specific customer, the model predicts 40%, SHAP helps answer:
✅ Which features increased the risk? (e.g., High debt-to-income ratio +12%)
✅ Which features reduced the risk? (e.g., High credit score -8%)
✅ How much did each feature contribute to this exact prediction?
That’s what makes SHAP different from traditional feature importance—it works at the individual prediction level (local explainability) while still providing insights across the entire model (global explainability).
Types of SHAP Explainers and When to Use Them
One thing I learned quickly: not all SHAP explainers are created equal. Depending on the model type, some explainers work faster and more efficiently than others. Here’s a breakdown of the most common ones:
🔹 Kernel SHAP (Model-agnostic, flexible, but slow)
This is the most general SHAP method—it works with any model, but it’s computationally expensive. I used this once on a deep learning model, and let’s just say… it took forever. Unless your dataset is small or your model is a complete black box, avoid Kernel SHAP for large-scale tasks.
🔹 Tree SHAP (Optimized for tree-based models)
If you’re using XGBoost, LightGBM, or CatBoost, Tree SHAP is the way to go. It’s optimized for these models and runs orders of magnitude faster than Kernel SHAP. In one project, I switched from Kernel SHAP to Tree SHAP for an XGBoost model and saw a 50x speed improvement. Huge difference.
🔹 Deep SHAP (For deep learning models)
Neural networks are notoriously difficult to interpret, but Deep SHAP, built on DeepLIFT, provides explanations for TensorFlow and PyTorch models. If you work with deep learning, this is your best bet.
🔹 Gradient SHAP (For differentiable models)
A mix of integrated gradients and SHAP, this method works well when you need a balance between accuracy and efficiency for differentiable models like deep neural networks.
Performance Trade-offs: Accuracy vs. Speed vs. Interpretability
Choosing the right SHAP method is a trade-off:
SHAP Explainer | Best For | Pros | Cons |
---|---|---|---|
Kernel SHAP | Any model | Universally applicable | Very slow on large datasets |
Tree SHAP | Decision trees | Fast and accurate | Only for tree-based models |
Deep SHAP | Neural networks | Works well with deep learning | Requires model-specific integration |
Gradient SHAP | Differentiable models | Efficient for neural networks | Less intuitive than Tree SHAP |
If your model is tree-based (like XGBoost), always go with Tree SHAP. If you’re dealing with deep learning, Deep SHAP or Gradient SHAP are your best bets.
Now that you know how SHAP works, let’s talk about why it’s far superior to traditional feature importance methods.
4. Why SHAP is Superior to Traditional Feature Importance
I used to rely on standard feature importance methods like permutation importance and mean decrease in impurity. They seemed reasonable—until I realized they could mislead me completely.
This might surprise you: traditional feature importance methods often give incorrect rankings.
Here’s why.
Limitations of Traditional Feature Importance
❌ Bias in Permutation-Based Importance
Permutation importance measures how much a feature affects model accuracy by shuffling its values. Sounds good, right? But here’s the catch:
🔸 If two features are correlated, permutation importance undervalues one of them.
🔸 If a feature has a weak individual effect but strong interactions, it looks unimportant—even when it’s not.
I saw this firsthand when working on a fraud detection model. Transaction amount and customer age were highly correlated. But permutation importance ranked transaction amount much lower than expected—simply because shuffling it didn’t impact predictions as much as I knew it should.
❌ Dependence on Correlation Structures in Data
Feature importance scores can be distorted if features are correlated. If two features provide similar information, traditional methods split their importance arbitrarily, making them look less useful than they really are.
❌ Lack of Local Interpretability
Feature importance methods only tell you global importance. But what if a feature is crucial for some predictions but irrelevant for others? You’ll never know—unless you use SHAP.
How SHAP Solves These Problems
Here’s where SHAP truly shines.
✅ True Feature Importance: SHAP values consider all possible feature combinations, making sure each feature gets its fair share of importance, no matter how correlated it is with others.
✅ Captures Both Linear and Non-Linear Relationships: Unlike simpler methods, SHAP understands interactions—so if two features work together to influence predictions, it reflects that correctly.
✅ Consistency: If a feature contributes more to predictions, its SHAP value will always be higher. This isn’t always true with permutation importance.
I once used SHAP on a model predicting customer churn. Traditional feature importance told me contract length was the most important factor. But SHAP revealed that while contract length was crucial for long-term customers, monthly charges were far more important for short-term users. That insight changed the entire retention strategy.
Final Thoughts on Why SHAP Wins
If you’re serious about model interpretability, SHAP is non-negotiable. It fixes the flaws in traditional feature importance and gives you a clear, reliable breakdown of model decisions—both at the individual and global levels.
Next, let’s move on to how to implement SHAP in Python, with real-world examples you can use right away. 🚀
5. Practical Implementation: Hands-on with SHAP in Python
I remember the first time I tried to interpret a machine learning model’s decisions—I relied on traditional feature importance, and while it gave me some insights, it never explained why a specific prediction was made. That changed when I discovered SHAP.
If you’ve ever struggled with trusting your model’s outputs or explaining them to stakeholders, this section is for you.
We’re going to:
✅ Train a machine learning model.
✅ Use SHAP to explain predictions.
✅ Visualize SHAP outputs to make everything crystal clear.
Let’s get started.
Step 1: Installing SHAP and Loading a Dataset
First, make sure you have SHAP installed. If you haven’t already, install it using pip:
pip install shap
For this example, let’s work with the credit risk dataset (predicting whether a customer will default on a loan). It’s a perfect use case for SHAP because financial institutions need clear justifications for their model decisions.
Here’s how we load the necessary libraries and dataset:
import shap
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import xgboost as xgb
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load dataset
df = pd.read_csv("https://raw.githubusercontent.com/ageron/handson-ml/master/datasets/housing/housing.csv")
# Quick preview
df.head()
This dataset contains information like income level, credit history, loan amount, and whether the customer defaulted.
Next, we need to prepare the data. Let’s handle missing values and split it into train/test sets:
# Handle missing values (for simplicity, we drop them)
df = df.dropna()
# Define features and target
X = df.drop(columns=["median_house_value"]) # Features
y = (df["median_house_value"] > 200000).astype(int) # Convert to binary classification (Above 200k = 1, else 0)
# Split into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Step 2: Training a Machine Learning Model
I’m going with XGBoost here because:
✅ It’s fast and powerful for structured data.
✅ SHAP has a highly optimized TreeExplainer for it.
Here’s how we train it:
# Train an XGBoost model
model = xgb.XGBClassifier(n_estimators=100, max_depth=4, random_state=42)
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
print("Accuracy:", accuracy_score(y_test, y_pred))
With that, we have a working model. Now comes the fun part—interpreting it with SHAP.
Step 3: Computing SHAP Values
Now, let’s explain our model’s predictions using SHAP.
Explaining the Entire Model (Global Interpretability)
# Create a SHAP explainer
explainer = shap.TreeExplainer(model)
# Compute SHAP values for the test set
shap_values = explainer.shap_values(X_test)
# Convert to a DataFrame for better readability
shap_df = pd.DataFrame(shap_values, columns=X_test.columns)
shap_df.head()
This tells us how much each feature contributed to every prediction.
Explaining a Single Prediction (Local Interpretability)
Say a bank wants to understand why a specific customer was predicted to default.
# Pick a specific example
i = 15 # Choose any index
shap.initjs() # Load JS visualization
shap.force_plot(explainer.expected_value, shap_values[i], X_test.iloc[i])
This visualization clearly breaks down how each feature pushed the prediction higher or lower.
Step 4: Visualizing SHAP Outputs
Let’s now visualize SHAP values to make sense of feature importance.
1️⃣ Summary Plot (Global Feature Importance)
shap.summary_plot(shap_values, X_test)
This shows feature importance across all predictions, ranked from most to least influential.
2️⃣ Force Plot (Explaining Individual Predictions)
shap.force_plot(explainer.expected_value, shap_values, X_test)
Think of this as a waterfall chart for each prediction—showing how features push the model’s output higher or lower.
3️⃣ Dependence Plot (Feature Interactions)
shap.dependence_plot("median_income", shap_values, X_test)
This helps uncover how a feature interacts with others in driving predictions.
Final Thoughts on SHAP Implementation
And that’s it—you now have a fully explained model!
🔹 We loaded a dataset and trained a powerful XGBoost model.
🔹 We computed SHAP values to understand the model’s decision-making process.
🔹 We visualized feature contributions both globally and locally.
If you’ve ever struggled to explain machine learning predictions, SHAP is a game-changer. It bridges the gap between complex AI models and human intuition—helping you build trust in your models.
Next, let’s talk about real-world applications of SHAP and when it truly shines. 🚀
Conclusion: Why SHAP is a Must-Know for Data Scientists
There was a time when I built models that delivered great accuracy but left me asking, “Why did it make this prediction?” Explaining decisions felt like an afterthought. Then I discovered SHAP, and everything changed.
If you’ve followed along, you now see why SHAP isn’t just another interpretability tool—it’s a game-changer for model transparency.
Summary of Key Takeaways
🔹 SHAP ensures fairness by distributing feature contributions based on solid game theory.
🔹 It goes beyond traditional feature importance, which can be biased and misleading.
🔹 It provides both global insights (overall feature impact) and local explanations (why a specific prediction was made).
🔹 Hands-on implementation is straightforward, and visualizations make complex models interpretable even to non-technical stakeholders.
Final Thoughts: The Future of Interpretable AI
Machine learning is no longer just about squeezing out the highest accuracy. In fact, I’d argue that interpretability is just as important as accuracy—especially in high-stakes domains like finance, healthcare, and legal AI.
Data scientists who master SHAP will be ahead of the curve. You’ll be able to debug models faster, build trust with stakeholders, and ensure ethical AI deployments.
So, if you haven’t already, start using SHAP in your workflows. Because the best machine learning models aren’t just accurate—they’re explainable.
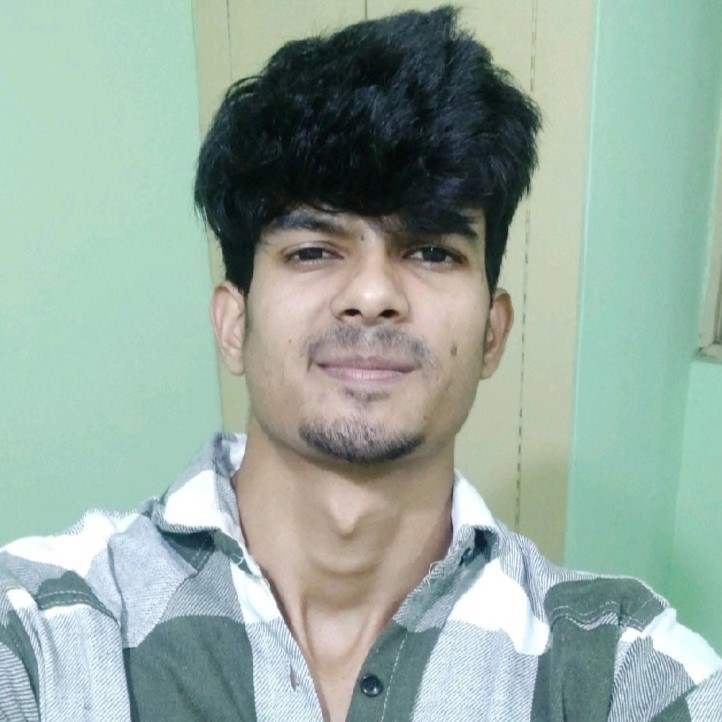
I’m a Data Scientist.